Simulate Bitwise Cyclic Tag
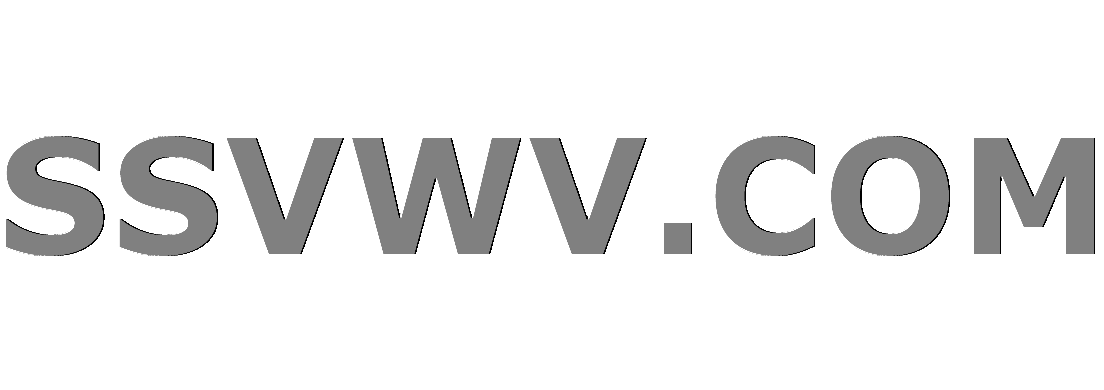
Multi tool use
$begingroup$
Challenge
Given two strings in any default I/O format, do the following:
NOTE: The challenge will refer to the first string as the "data" and the second referred to as the "program".
- Change the program to an infinite string which is just the program repeated infinitely (e.g.
10
-->1010101010...
). The challenge will refer to this as the "infinite program"
While the data is non-empty, do the following while looping over the infinite program:
a. If the current command is "0", delete the left-most bit in the data. If the data is empty, "0" does not do anything.
b. If the current command is "1", append the next character in the program to the data if the left-most bit in the data is a one.
c. If the data is not empty now, output the data.
Test Cases
Data is the left side of the input and the program is the right side.
100, 0 --> 00, 0
1111, 1 --> 11111, 111111, 1111111, ...
10, 011 --> 0, 0, 0
1110, 011 --> 110, 1101, 11010, 1010...
Notes
- The data and program will consist of only 0s and 1s
- For data/programs that do not halt, your program does not need to halt.
- The data and program will not be empty in the input.
- You may have multiple trailing and leading newlines
Standard Loopholes are forbidden- You can use any convenient I/O format
As always with code-golf, shortest code wins!
code-golf interpreter
$endgroup$
|
show 10 more comments
$begingroup$
Challenge
Given two strings in any default I/O format, do the following:
NOTE: The challenge will refer to the first string as the "data" and the second referred to as the "program".
- Change the program to an infinite string which is just the program repeated infinitely (e.g.
10
-->1010101010...
). The challenge will refer to this as the "infinite program"
While the data is non-empty, do the following while looping over the infinite program:
a. If the current command is "0", delete the left-most bit in the data. If the data is empty, "0" does not do anything.
b. If the current command is "1", append the next character in the program to the data if the left-most bit in the data is a one.
c. If the data is not empty now, output the data.
Test Cases
Data is the left side of the input and the program is the right side.
100, 0 --> 00, 0
1111, 1 --> 11111, 111111, 1111111, ...
10, 011 --> 0, 0, 0
1110, 011 --> 110, 1101, 11010, 1010...
Notes
- The data and program will consist of only 0s and 1s
- For data/programs that do not halt, your program does not need to halt.
- The data and program will not be empty in the input.
- You may have multiple trailing and leading newlines
Standard Loopholes are forbidden- You can use any convenient I/O format
As always with code-golf, shortest code wins!
code-golf interpreter
$endgroup$
$begingroup$
@Sanchises Seems like a borderline duplicate to that, but you have to get the result at a certain generation and that is for any cyclic tag system.
$endgroup$
– MilkyWay90
Apr 7 at 17:49
$begingroup$
in the first test case,100
goes to10
on cmd0
, whose definition is "delete the left-most bit in the data." wouldn't the leftmost bit of100
be1
?
$endgroup$
– Jonah
Apr 7 at 17:52
$begingroup$
@Jonah Oh, missed that
$endgroup$
– MilkyWay90
Apr 7 at 17:55
$begingroup$
in case (b), if you do the append, does the instruction pointer move right one or two characters?
$endgroup$
– Sparr
Apr 7 at 18:08
$begingroup$
@Sparr It moves right one. See the section labeled Challenge.
$endgroup$
– MilkyWay90
Apr 7 at 18:11
|
show 10 more comments
$begingroup$
Challenge
Given two strings in any default I/O format, do the following:
NOTE: The challenge will refer to the first string as the "data" and the second referred to as the "program".
- Change the program to an infinite string which is just the program repeated infinitely (e.g.
10
-->1010101010...
). The challenge will refer to this as the "infinite program"
While the data is non-empty, do the following while looping over the infinite program:
a. If the current command is "0", delete the left-most bit in the data. If the data is empty, "0" does not do anything.
b. If the current command is "1", append the next character in the program to the data if the left-most bit in the data is a one.
c. If the data is not empty now, output the data.
Test Cases
Data is the left side of the input and the program is the right side.
100, 0 --> 00, 0
1111, 1 --> 11111, 111111, 1111111, ...
10, 011 --> 0, 0, 0
1110, 011 --> 110, 1101, 11010, 1010...
Notes
- The data and program will consist of only 0s and 1s
- For data/programs that do not halt, your program does not need to halt.
- The data and program will not be empty in the input.
- You may have multiple trailing and leading newlines
Standard Loopholes are forbidden- You can use any convenient I/O format
As always with code-golf, shortest code wins!
code-golf interpreter
$endgroup$
Challenge
Given two strings in any default I/O format, do the following:
NOTE: The challenge will refer to the first string as the "data" and the second referred to as the "program".
- Change the program to an infinite string which is just the program repeated infinitely (e.g.
10
-->1010101010...
). The challenge will refer to this as the "infinite program"
While the data is non-empty, do the following while looping over the infinite program:
a. If the current command is "0", delete the left-most bit in the data. If the data is empty, "0" does not do anything.
b. If the current command is "1", append the next character in the program to the data if the left-most bit in the data is a one.
c. If the data is not empty now, output the data.
Test Cases
Data is the left side of the input and the program is the right side.
100, 0 --> 00, 0
1111, 1 --> 11111, 111111, 1111111, ...
10, 011 --> 0, 0, 0
1110, 011 --> 110, 1101, 11010, 1010...
Notes
- The data and program will consist of only 0s and 1s
- For data/programs that do not halt, your program does not need to halt.
- The data and program will not be empty in the input.
- You may have multiple trailing and leading newlines
Standard Loopholes are forbidden- You can use any convenient I/O format
As always with code-golf, shortest code wins!
code-golf interpreter
code-golf interpreter
edited Apr 7 at 21:46
MilkyWay90
asked Apr 7 at 17:09


MilkyWay90MilkyWay90
745316
745316
$begingroup$
@Sanchises Seems like a borderline duplicate to that, but you have to get the result at a certain generation and that is for any cyclic tag system.
$endgroup$
– MilkyWay90
Apr 7 at 17:49
$begingroup$
in the first test case,100
goes to10
on cmd0
, whose definition is "delete the left-most bit in the data." wouldn't the leftmost bit of100
be1
?
$endgroup$
– Jonah
Apr 7 at 17:52
$begingroup$
@Jonah Oh, missed that
$endgroup$
– MilkyWay90
Apr 7 at 17:55
$begingroup$
in case (b), if you do the append, does the instruction pointer move right one or two characters?
$endgroup$
– Sparr
Apr 7 at 18:08
$begingroup$
@Sparr It moves right one. See the section labeled Challenge.
$endgroup$
– MilkyWay90
Apr 7 at 18:11
|
show 10 more comments
$begingroup$
@Sanchises Seems like a borderline duplicate to that, but you have to get the result at a certain generation and that is for any cyclic tag system.
$endgroup$
– MilkyWay90
Apr 7 at 17:49
$begingroup$
in the first test case,100
goes to10
on cmd0
, whose definition is "delete the left-most bit in the data." wouldn't the leftmost bit of100
be1
?
$endgroup$
– Jonah
Apr 7 at 17:52
$begingroup$
@Jonah Oh, missed that
$endgroup$
– MilkyWay90
Apr 7 at 17:55
$begingroup$
in case (b), if you do the append, does the instruction pointer move right one or two characters?
$endgroup$
– Sparr
Apr 7 at 18:08
$begingroup$
@Sparr It moves right one. See the section labeled Challenge.
$endgroup$
– MilkyWay90
Apr 7 at 18:11
$begingroup$
@Sanchises Seems like a borderline duplicate to that, but you have to get the result at a certain generation and that is for any cyclic tag system.
$endgroup$
– MilkyWay90
Apr 7 at 17:49
$begingroup$
@Sanchises Seems like a borderline duplicate to that, but you have to get the result at a certain generation and that is for any cyclic tag system.
$endgroup$
– MilkyWay90
Apr 7 at 17:49
$begingroup$
in the first test case,
100
goes to 10
on cmd 0
, whose definition is "delete the left-most bit in the data." wouldn't the leftmost bit of 100
be 1
?$endgroup$
– Jonah
Apr 7 at 17:52
$begingroup$
in the first test case,
100
goes to 10
on cmd 0
, whose definition is "delete the left-most bit in the data." wouldn't the leftmost bit of 100
be 1
?$endgroup$
– Jonah
Apr 7 at 17:52
$begingroup$
@Jonah Oh, missed that
$endgroup$
– MilkyWay90
Apr 7 at 17:55
$begingroup$
@Jonah Oh, missed that
$endgroup$
– MilkyWay90
Apr 7 at 17:55
$begingroup$
in case (b), if you do the append, does the instruction pointer move right one or two characters?
$endgroup$
– Sparr
Apr 7 at 18:08
$begingroup$
in case (b), if you do the append, does the instruction pointer move right one or two characters?
$endgroup$
– Sparr
Apr 7 at 18:08
$begingroup$
@Sparr It moves right one. See the section labeled Challenge.
$endgroup$
– MilkyWay90
Apr 7 at 18:11
$begingroup$
@Sparr It moves right one. See the section labeled Challenge.
$endgroup$
– MilkyWay90
Apr 7 at 18:11
|
show 10 more comments
11 Answers
11
active
oldest
votes
$begingroup$
Haskell, 77 71 62 bytes
f@(d:e)#(p:q)=f:[e,f++take d q]!!p#q
_#_=
a!b=tail$a#cycle b
Try it online!
Edit: -9 bytes thanks to @xnor.
$endgroup$
1
$begingroup$
In the first line, you can dof:[e,f++take d q]!!p#q
.
$endgroup$
– xnor
2 days ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
m=>n=>{for(int i=0;m!="";Print(m=n[i++]<49?m.Substring(1):m[0]>48?m+n[i]:m))n+=n;}
Try it online!
$endgroup$
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
1
$begingroup$
@Jonah 48 is the ASCII value of0
, and 49 is the ASCII value of1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@EmbodimentofIgnorance why not use aList
andSkip
, or something like that
$endgroup$
– ASCII-only
Apr 8 at 1:04
|
show 1 more comment
$begingroup$
J, 65 bytes
(([:(][echo)(}.@[)`([,{.@[#1{],])@.({.@]));1|.])&>/^:(0<0#@{>)^:5
Try it online!
I may golf this further later. Note the 5
at the end would be infinity _
in the actual program, but I've left it there to make running the non-halting examples easier.
$endgroup$
add a comment |
$begingroup$
Python 3, 74 bytes
def f(d,p):
while d:c,*p=p+p[:1];d=(d[1:],d+p[:1]*d[0])[c];d and print(d)
Try it online!
Arguments: d
: data, p
: program.
$endgroup$
add a comment |
$begingroup$
05AB1E, 24 21 bytes
[¹Nèi¬i¹N>è«}ë¦}DõQ#=
Takes the program as first input and data as second input.input.
Try it online.
Explanation:
[ # Start an infinite loop:
¹Nè # Get the N'th digit of the first (program) input
# (NOTES: N is the index of the infinite loop;
# indexing in 05AB1E automatically wraps around)
i # If this digit is 1:
¬ # Push the head of the current data (without popping it)
# (will take the second (data) input implicitly if it's the first iteration)
i } # If this head is 1:
¹N>è # Get the (N+1)'th digit of the first (program) input
« # And append it to the current data
ë } # Else (the digit is a 0 instead):
¦ # Remove the first digit from the current data
# (will take the second input (data) implicitly if it's the first iteration)
DõQ # If the current data is an empty string:
# # Stop the infinite loop
= # Print the current data with trailing newline (without popping it)
$endgroup$
add a comment |
$begingroup$
Ruby, 62 59 bytes
->c,d{p(d)while(a,*c=c;b,*d=d;c<<a;!=d=[b]*a+d+c[0,a*b])}
Try it online!
How
- Get the first bit from code
c
and datad
, call thema
andb
. Puta
back at the end ofc
. - Put back
b
at the beginning ofd
ifa==1
. This can be shortened to[b]*a
- Put the first byte of
c
at the end ofd
ifa==1 and b==1
. This can be shortened toc[0,a*b]
. - If we have more data, print and repeat.
$endgroup$
add a comment |
$begingroup$
Python 2, 96 82 bytes
def g(d,p):
while d:
c=p[0];p=p[1:]+[c];d=[d[1:],d+[p[0]]*d[0]][c]
if d:yield d
Try it online!
Stealing a bit from Emodiment of Ignorance's answer...
A generator which uses lists of 1's and 0's for input / output.
$endgroup$
add a comment |
$begingroup$
Jelly, 40 bytes
;€Ø2œịxØ1œị$Ʋ$Ḋ€2,1œị$?1¦ṙ€1$2¦µ⁺1ịGṄƲ¿Ḣ
Try it online!
I’ve assumed trailing newlines are ok. I’ve also gone with a list of two lists of zeros and ones as input, and output to stdout.
$endgroup$
add a comment |
$begingroup$
Python 1, 75 bytes
a,b=input()
while a:b=b[1:]+b[:1];a=[a[1:],a+b[:1]*a[0]][b[0]];print a or''
Try it online!
$endgroup$
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty arrayinstead of a newline on e.g., data
[1,0,0]
, program[0]
.
$endgroup$
– Chas Brown
Apr 7 at 23:47
1
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
add a comment |
$begingroup$
C++ (gcc), 178 bytes
void a(std::string s,std::string d){while(!s.empty())for(int i=0;i<d.size();i++){if(d[i]=='0')s.erase(0,1);else if(s[0]=='1')s.push_back(d[(i+1)>=d.size()?0:i+1]);std::cout<<s;}}
Try it online!
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
add a comment |
$begingroup$
C++ (gcc), 294 289 bytes
-5 bytes thanks to @ceilingcat
#import<iostream>
#import<queue>
void a(char*e,char*p){std::queue<char>d;for(;*e;)d.push(*e++);for(char*c=p;d.size();c=*++c?c:p){*c-49?d.pop():d.front()-48?d.push(c[1]?c[1]:*p):a("","");if(d.size()){for(int i=0;i++<d.size();d.pop())std::cout<<d.front(),d.push(d.front());std::cout<<" ";}}}
Try it online!
Fairly straightforward algorithm. Copies the data into a queue, and repeatedly loops through the program. On a "0", it removes the first element in the queue (the first "bit"). On a 1, it adds the next "bit" of the program to the data if the first "bit" of the data is 1. Then it loops through the data, printing it "bit" by "bit", and finally prints a space to separate successive data entries.
$endgroup$
$begingroup$
@ceilingcat Clever (ab)use ofc[1]
! Updated.
$endgroup$
– Neil A.
yesterday
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f182788%2fsimulate-bitwise-cyclic-tag%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
11 Answers
11
active
oldest
votes
11 Answers
11
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Haskell, 77 71 62 bytes
f@(d:e)#(p:q)=f:[e,f++take d q]!!p#q
_#_=
a!b=tail$a#cycle b
Try it online!
Edit: -9 bytes thanks to @xnor.
$endgroup$
1
$begingroup$
In the first line, you can dof:[e,f++take d q]!!p#q
.
$endgroup$
– xnor
2 days ago
add a comment |
$begingroup$
Haskell, 77 71 62 bytes
f@(d:e)#(p:q)=f:[e,f++take d q]!!p#q
_#_=
a!b=tail$a#cycle b
Try it online!
Edit: -9 bytes thanks to @xnor.
$endgroup$
1
$begingroup$
In the first line, you can dof:[e,f++take d q]!!p#q
.
$endgroup$
– xnor
2 days ago
add a comment |
$begingroup$
Haskell, 77 71 62 bytes
f@(d:e)#(p:q)=f:[e,f++take d q]!!p#q
_#_=
a!b=tail$a#cycle b
Try it online!
Edit: -9 bytes thanks to @xnor.
$endgroup$
Haskell, 77 71 62 bytes
f@(d:e)#(p:q)=f:[e,f++take d q]!!p#q
_#_=
a!b=tail$a#cycle b
Try it online!
Edit: -9 bytes thanks to @xnor.
edited 2 days ago
answered Apr 7 at 21:00
niminimi
32.7k32489
32.7k32489
1
$begingroup$
In the first line, you can dof:[e,f++take d q]!!p#q
.
$endgroup$
– xnor
2 days ago
add a comment |
1
$begingroup$
In the first line, you can dof:[e,f++take d q]!!p#q
.
$endgroup$
– xnor
2 days ago
1
1
$begingroup$
In the first line, you can do
f:[e,f++take d q]!!p#q
.$endgroup$
– xnor
2 days ago
$begingroup$
In the first line, you can do
f:[e,f++take d q]!!p#q
.$endgroup$
– xnor
2 days ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
m=>n=>{for(int i=0;m!="";Print(m=n[i++]<49?m.Substring(1):m[0]>48?m+n[i]:m))n+=n;}
Try it online!
$endgroup$
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
1
$begingroup$
@Jonah 48 is the ASCII value of0
, and 49 is the ASCII value of1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@EmbodimentofIgnorance why not use aList
andSkip
, or something like that
$endgroup$
– ASCII-only
Apr 8 at 1:04
|
show 1 more comment
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
m=>n=>{for(int i=0;m!="";Print(m=n[i++]<49?m.Substring(1):m[0]>48?m+n[i]:m))n+=n;}
Try it online!
$endgroup$
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
1
$begingroup$
@Jonah 48 is the ASCII value of0
, and 49 is the ASCII value of1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@EmbodimentofIgnorance why not use aList
andSkip
, or something like that
$endgroup$
– ASCII-only
Apr 8 at 1:04
|
show 1 more comment
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
m=>n=>{for(int i=0;m!="";Print(m=n[i++]<49?m.Substring(1):m[0]>48?m+n[i]:m))n+=n;}
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 82 bytes
m=>n=>{for(int i=0;m!="";Print(m=n[i++]<49?m.Substring(1):m[0]>48?m+n[i]:m))n+=n;}
Try it online!
edited Apr 7 at 22:59
answered Apr 7 at 18:55
Embodiment of IgnoranceEmbodiment of Ignorance
2,926127
2,926127
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
1
$begingroup$
@Jonah 48 is the ASCII value of0
, and 49 is the ASCII value of1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@EmbodimentofIgnorance why not use aList
andSkip
, or something like that
$endgroup$
– ASCII-only
Apr 8 at 1:04
|
show 1 more comment
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
1
$begingroup$
@Jonah 48 is the ASCII value of0
, and 49 is the ASCII value of1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@EmbodimentofIgnorance why not use aList
andSkip
, or something like that
$endgroup$
– ASCII-only
Apr 8 at 1:04
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
$begingroup$
what are the significance of the 48 and 49, out of curiosity?
$endgroup$
– Jonah
Apr 7 at 18:59
1
1
$begingroup$
@Jonah 48 is the ASCII value of
0
, and 49 is the ASCII value of 1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
@Jonah 48 is the ASCII value of
0
, and 49 is the ASCII value of 1
$endgroup$
– Embodiment of Ignorance
Apr 7 at 19:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
shouldn't you use 0 and 1 instead here :P
$endgroup$
– ASCII-only
Apr 8 at 1:02
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@ASCII-only I'm using a string, not an array.
$endgroup$
– Embodiment of Ignorance
Apr 8 at 1:03
$begingroup$
@EmbodimentofIgnorance why not use a
List
and Skip
, or something like that$endgroup$
– ASCII-only
Apr 8 at 1:04
$begingroup$
@EmbodimentofIgnorance why not use a
List
and Skip
, or something like that$endgroup$
– ASCII-only
Apr 8 at 1:04
|
show 1 more comment
$begingroup$
J, 65 bytes
(([:(][echo)(}.@[)`([,{.@[#1{],])@.({.@]));1|.])&>/^:(0<0#@{>)^:5
Try it online!
I may golf this further later. Note the 5
at the end would be infinity _
in the actual program, but I've left it there to make running the non-halting examples easier.
$endgroup$
add a comment |
$begingroup$
J, 65 bytes
(([:(][echo)(}.@[)`([,{.@[#1{],])@.({.@]));1|.])&>/^:(0<0#@{>)^:5
Try it online!
I may golf this further later. Note the 5
at the end would be infinity _
in the actual program, but I've left it there to make running the non-halting examples easier.
$endgroup$
add a comment |
$begingroup$
J, 65 bytes
(([:(][echo)(}.@[)`([,{.@[#1{],])@.({.@]));1|.])&>/^:(0<0#@{>)^:5
Try it online!
I may golf this further later. Note the 5
at the end would be infinity _
in the actual program, but I've left it there to make running the non-halting examples easier.
$endgroup$
J, 65 bytes
(([:(][echo)(}.@[)`([,{.@[#1{],])@.({.@]));1|.])&>/^:(0<0#@{>)^:5
Try it online!
I may golf this further later. Note the 5
at the end would be infinity _
in the actual program, but I've left it there to make running the non-halting examples easier.
edited Apr 7 at 19:05
answered Apr 7 at 18:57


JonahJonah
2,6711017
2,6711017
add a comment |
add a comment |
$begingroup$
Python 3, 74 bytes
def f(d,p):
while d:c,*p=p+p[:1];d=(d[1:],d+p[:1]*d[0])[c];d and print(d)
Try it online!
Arguments: d
: data, p
: program.
$endgroup$
add a comment |
$begingroup$
Python 3, 74 bytes
def f(d,p):
while d:c,*p=p+p[:1];d=(d[1:],d+p[:1]*d[0])[c];d and print(d)
Try it online!
Arguments: d
: data, p
: program.
$endgroup$
add a comment |
$begingroup$
Python 3, 74 bytes
def f(d,p):
while d:c,*p=p+p[:1];d=(d[1:],d+p[:1]*d[0])[c];d and print(d)
Try it online!
Arguments: d
: data, p
: program.
$endgroup$
Python 3, 74 bytes
def f(d,p):
while d:c,*p=p+p[:1];d=(d[1:],d+p[:1]*d[0])[c];d and print(d)
Try it online!
Arguments: d
: data, p
: program.
answered Apr 7 at 21:56


Erik the OutgolferErik the Outgolfer
33k429106
33k429106
add a comment |
add a comment |
$begingroup$
05AB1E, 24 21 bytes
[¹Nèi¬i¹N>è«}ë¦}DõQ#=
Takes the program as first input and data as second input.input.
Try it online.
Explanation:
[ # Start an infinite loop:
¹Nè # Get the N'th digit of the first (program) input
# (NOTES: N is the index of the infinite loop;
# indexing in 05AB1E automatically wraps around)
i # If this digit is 1:
¬ # Push the head of the current data (without popping it)
# (will take the second (data) input implicitly if it's the first iteration)
i } # If this head is 1:
¹N>è # Get the (N+1)'th digit of the first (program) input
« # And append it to the current data
ë } # Else (the digit is a 0 instead):
¦ # Remove the first digit from the current data
# (will take the second input (data) implicitly if it's the first iteration)
DõQ # If the current data is an empty string:
# # Stop the infinite loop
= # Print the current data with trailing newline (without popping it)
$endgroup$
add a comment |
$begingroup$
05AB1E, 24 21 bytes
[¹Nèi¬i¹N>è«}ë¦}DõQ#=
Takes the program as first input and data as second input.input.
Try it online.
Explanation:
[ # Start an infinite loop:
¹Nè # Get the N'th digit of the first (program) input
# (NOTES: N is the index of the infinite loop;
# indexing in 05AB1E automatically wraps around)
i # If this digit is 1:
¬ # Push the head of the current data (without popping it)
# (will take the second (data) input implicitly if it's the first iteration)
i } # If this head is 1:
¹N>è # Get the (N+1)'th digit of the first (program) input
« # And append it to the current data
ë } # Else (the digit is a 0 instead):
¦ # Remove the first digit from the current data
# (will take the second input (data) implicitly if it's the first iteration)
DõQ # If the current data is an empty string:
# # Stop the infinite loop
= # Print the current data with trailing newline (without popping it)
$endgroup$
add a comment |
$begingroup$
05AB1E, 24 21 bytes
[¹Nèi¬i¹N>è«}ë¦}DõQ#=
Takes the program as first input and data as second input.input.
Try it online.
Explanation:
[ # Start an infinite loop:
¹Nè # Get the N'th digit of the first (program) input
# (NOTES: N is the index of the infinite loop;
# indexing in 05AB1E automatically wraps around)
i # If this digit is 1:
¬ # Push the head of the current data (without popping it)
# (will take the second (data) input implicitly if it's the first iteration)
i } # If this head is 1:
¹N>è # Get the (N+1)'th digit of the first (program) input
« # And append it to the current data
ë } # Else (the digit is a 0 instead):
¦ # Remove the first digit from the current data
# (will take the second input (data) implicitly if it's the first iteration)
DõQ # If the current data is an empty string:
# # Stop the infinite loop
= # Print the current data with trailing newline (without popping it)
$endgroup$
05AB1E, 24 21 bytes
[¹Nèi¬i¹N>è«}ë¦}DõQ#=
Takes the program as first input and data as second input.input.
Try it online.
Explanation:
[ # Start an infinite loop:
¹Nè # Get the N'th digit of the first (program) input
# (NOTES: N is the index of the infinite loop;
# indexing in 05AB1E automatically wraps around)
i # If this digit is 1:
¬ # Push the head of the current data (without popping it)
# (will take the second (data) input implicitly if it's the first iteration)
i } # If this head is 1:
¹N>è # Get the (N+1)'th digit of the first (program) input
« # And append it to the current data
ë } # Else (the digit is a 0 instead):
¦ # Remove the first digit from the current data
# (will take the second input (data) implicitly if it's the first iteration)
DõQ # If the current data is an empty string:
# # Stop the infinite loop
= # Print the current data with trailing newline (without popping it)
edited 2 days ago
answered 2 days ago


Kevin CruijssenKevin Cruijssen
42.6k571217
42.6k571217
add a comment |
add a comment |
$begingroup$
Ruby, 62 59 bytes
->c,d{p(d)while(a,*c=c;b,*d=d;c<<a;!=d=[b]*a+d+c[0,a*b])}
Try it online!
How
- Get the first bit from code
c
and datad
, call thema
andb
. Puta
back at the end ofc
. - Put back
b
at the beginning ofd
ifa==1
. This can be shortened to[b]*a
- Put the first byte of
c
at the end ofd
ifa==1 and b==1
. This can be shortened toc[0,a*b]
. - If we have more data, print and repeat.
$endgroup$
add a comment |
$begingroup$
Ruby, 62 59 bytes
->c,d{p(d)while(a,*c=c;b,*d=d;c<<a;!=d=[b]*a+d+c[0,a*b])}
Try it online!
How
- Get the first bit from code
c
and datad
, call thema
andb
. Puta
back at the end ofc
. - Put back
b
at the beginning ofd
ifa==1
. This can be shortened to[b]*a
- Put the first byte of
c
at the end ofd
ifa==1 and b==1
. This can be shortened toc[0,a*b]
. - If we have more data, print and repeat.
$endgroup$
add a comment |
$begingroup$
Ruby, 62 59 bytes
->c,d{p(d)while(a,*c=c;b,*d=d;c<<a;!=d=[b]*a+d+c[0,a*b])}
Try it online!
How
- Get the first bit from code
c
and datad
, call thema
andb
. Puta
back at the end ofc
. - Put back
b
at the beginning ofd
ifa==1
. This can be shortened to[b]*a
- Put the first byte of
c
at the end ofd
ifa==1 and b==1
. This can be shortened toc[0,a*b]
. - If we have more data, print and repeat.
$endgroup$
Ruby, 62 59 bytes
->c,d{p(d)while(a,*c=c;b,*d=d;c<<a;!=d=[b]*a+d+c[0,a*b])}
Try it online!
How
- Get the first bit from code
c
and datad
, call thema
andb
. Puta
back at the end ofc
. - Put back
b
at the beginning ofd
ifa==1
. This can be shortened to[b]*a
- Put the first byte of
c
at the end ofd
ifa==1 and b==1
. This can be shortened toc[0,a*b]
. - If we have more data, print and repeat.
edited yesterday
answered yesterday
G BG B
8,2661429
8,2661429
add a comment |
add a comment |
$begingroup$
Python 2, 96 82 bytes
def g(d,p):
while d:
c=p[0];p=p[1:]+[c];d=[d[1:],d+[p[0]]*d[0]][c]
if d:yield d
Try it online!
Stealing a bit from Emodiment of Ignorance's answer...
A generator which uses lists of 1's and 0's for input / output.
$endgroup$
add a comment |
$begingroup$
Python 2, 96 82 bytes
def g(d,p):
while d:
c=p[0];p=p[1:]+[c];d=[d[1:],d+[p[0]]*d[0]][c]
if d:yield d
Try it online!
Stealing a bit from Emodiment of Ignorance's answer...
A generator which uses lists of 1's and 0's for input / output.
$endgroup$
add a comment |
$begingroup$
Python 2, 96 82 bytes
def g(d,p):
while d:
c=p[0];p=p[1:]+[c];d=[d[1:],d+[p[0]]*d[0]][c]
if d:yield d
Try it online!
Stealing a bit from Emodiment of Ignorance's answer...
A generator which uses lists of 1's and 0's for input / output.
$endgroup$
Python 2, 96 82 bytes
def g(d,p):
while d:
c=p[0];p=p[1:]+[c];d=[d[1:],d+[p[0]]*d[0]][c]
if d:yield d
Try it online!
Stealing a bit from Emodiment of Ignorance's answer...
A generator which uses lists of 1's and 0's for input / output.
edited Apr 7 at 19:57
answered Apr 7 at 19:19
Chas BrownChas Brown
5,2291523
5,2291523
add a comment |
add a comment |
$begingroup$
Jelly, 40 bytes
;€Ø2œịxØ1œị$Ʋ$Ḋ€2,1œị$?1¦ṙ€1$2¦µ⁺1ịGṄƲ¿Ḣ
Try it online!
I’ve assumed trailing newlines are ok. I’ve also gone with a list of two lists of zeros and ones as input, and output to stdout.
$endgroup$
add a comment |
$begingroup$
Jelly, 40 bytes
;€Ø2œịxØ1œị$Ʋ$Ḋ€2,1œị$?1¦ṙ€1$2¦µ⁺1ịGṄƲ¿Ḣ
Try it online!
I’ve assumed trailing newlines are ok. I’ve also gone with a list of two lists of zeros and ones as input, and output to stdout.
$endgroup$
add a comment |
$begingroup$
Jelly, 40 bytes
;€Ø2œịxØ1œị$Ʋ$Ḋ€2,1œị$?1¦ṙ€1$2¦µ⁺1ịGṄƲ¿Ḣ
Try it online!
I’ve assumed trailing newlines are ok. I’ve also gone with a list of two lists of zeros and ones as input, and output to stdout.
$endgroup$
Jelly, 40 bytes
;€Ø2œịxØ1œị$Ʋ$Ḋ€2,1œị$?1¦ṙ€1$2¦µ⁺1ịGṄƲ¿Ḣ
Try it online!
I’ve assumed trailing newlines are ok. I’ve also gone with a list of two lists of zeros and ones as input, and output to stdout.
answered Apr 7 at 21:36
Nick KennedyNick Kennedy
1,50649
1,50649
add a comment |
add a comment |
$begingroup$
Python 1, 75 bytes
a,b=input()
while a:b=b[1:]+b[:1];a=[a[1:],a+b[:1]*a[0]][b[0]];print a or''
Try it online!
$endgroup$
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty arrayinstead of a newline on e.g., data
[1,0,0]
, program[0]
.
$endgroup$
– Chas Brown
Apr 7 at 23:47
1
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
add a comment |
$begingroup$
Python 1, 75 bytes
a,b=input()
while a:b=b[1:]+b[:1];a=[a[1:],a+b[:1]*a[0]][b[0]];print a or''
Try it online!
$endgroup$
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty arrayinstead of a newline on e.g., data
[1,0,0]
, program[0]
.
$endgroup$
– Chas Brown
Apr 7 at 23:47
1
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
add a comment |
$begingroup$
Python 1, 75 bytes
a,b=input()
while a:b=b[1:]+b[:1];a=[a[1:],a+b[:1]*a[0]][b[0]];print a or''
Try it online!
$endgroup$
Python 1, 75 bytes
a,b=input()
while a:b=b[1:]+b[:1];a=[a[1:],a+b[:1]*a[0]][b[0]];print a or''
Try it online!
edited 2 days ago
answered Apr 7 at 19:14
Embodiment of IgnoranceEmbodiment of Ignorance
2,926127
2,926127
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty arrayinstead of a newline on e.g., data
[1,0,0]
, program[0]
.
$endgroup$
– Chas Brown
Apr 7 at 23:47
1
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
add a comment |
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty arrayinstead of a newline on e.g., data
[1,0,0]
, program[0]
.
$endgroup$
– Chas Brown
Apr 7 at 23:47
1
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
Nice! A niggle: for data '100', program '0', this will print the empty string once: but rule c says "If the data is not empty now, output the data."
$endgroup$
– Chas Brown
Apr 7 at 19:33
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown Small typo, I'm waiting for clarification from the OP if trailing newlines are ok
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:13
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
@ChasBrown The OP says multiple trailing newlines are allowed, see here
$endgroup$
– Embodiment of Ignorance
Apr 7 at 21:46
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty array
instead of a newline on e.g., data [1,0,0]
, program [0]
.$endgroup$
– Chas Brown
Apr 7 at 23:47
$begingroup$
But after switching to arrays of 1's and 0s, now you're printing an empty array
instead of a newline on e.g., data [1,0,0]
, program [0]
.$endgroup$
– Chas Brown
Apr 7 at 23:47
1
1
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
$begingroup$
python 1? python 2 doesn't work?
$endgroup$
– ASCII-only
Apr 8 at 0:29
add a comment |
$begingroup$
C++ (gcc), 178 bytes
void a(std::string s,std::string d){while(!s.empty())for(int i=0;i<d.size();i++){if(d[i]=='0')s.erase(0,1);else if(s[0]=='1')s.push_back(d[(i+1)>=d.size()?0:i+1]);std::cout<<s;}}
Try it online!
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
add a comment |
$begingroup$
C++ (gcc), 178 bytes
void a(std::string s,std::string d){while(!s.empty())for(int i=0;i<d.size();i++){if(d[i]=='0')s.erase(0,1);else if(s[0]=='1')s.push_back(d[(i+1)>=d.size()?0:i+1]);std::cout<<s;}}
Try it online!
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
add a comment |
$begingroup$
C++ (gcc), 178 bytes
void a(std::string s,std::string d){while(!s.empty())for(int i=0;i<d.size();i++){if(d[i]=='0')s.erase(0,1);else if(s[0]=='1')s.push_back(d[(i+1)>=d.size()?0:i+1]);std::cout<<s;}}
Try it online!
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
C++ (gcc), 178 bytes
void a(std::string s,std::string d){while(!s.empty())for(int i=0;i<d.size();i++){if(d[i]=='0')s.erase(0,1);else if(s[0]=='1')s.push_back(d[(i+1)>=d.size()?0:i+1]);std::cout<<s;}}
Try it online!
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered yesterday
peterzugerpeterzuger
235
235
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
peterzuger is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
add a comment |
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
$begingroup$
163 bytes
$endgroup$
– ceilingcat
8 hours ago
add a comment |
$begingroup$
C++ (gcc), 294 289 bytes
-5 bytes thanks to @ceilingcat
#import<iostream>
#import<queue>
void a(char*e,char*p){std::queue<char>d;for(;*e;)d.push(*e++);for(char*c=p;d.size();c=*++c?c:p){*c-49?d.pop():d.front()-48?d.push(c[1]?c[1]:*p):a("","");if(d.size()){for(int i=0;i++<d.size();d.pop())std::cout<<d.front(),d.push(d.front());std::cout<<" ";}}}
Try it online!
Fairly straightforward algorithm. Copies the data into a queue, and repeatedly loops through the program. On a "0", it removes the first element in the queue (the first "bit"). On a 1, it adds the next "bit" of the program to the data if the first "bit" of the data is 1. Then it loops through the data, printing it "bit" by "bit", and finally prints a space to separate successive data entries.
$endgroup$
$begingroup$
@ceilingcat Clever (ab)use ofc[1]
! Updated.
$endgroup$
– Neil A.
yesterday
add a comment |
$begingroup$
C++ (gcc), 294 289 bytes
-5 bytes thanks to @ceilingcat
#import<iostream>
#import<queue>
void a(char*e,char*p){std::queue<char>d;for(;*e;)d.push(*e++);for(char*c=p;d.size();c=*++c?c:p){*c-49?d.pop():d.front()-48?d.push(c[1]?c[1]:*p):a("","");if(d.size()){for(int i=0;i++<d.size();d.pop())std::cout<<d.front(),d.push(d.front());std::cout<<" ";}}}
Try it online!
Fairly straightforward algorithm. Copies the data into a queue, and repeatedly loops through the program. On a "0", it removes the first element in the queue (the first "bit"). On a 1, it adds the next "bit" of the program to the data if the first "bit" of the data is 1. Then it loops through the data, printing it "bit" by "bit", and finally prints a space to separate successive data entries.
$endgroup$
$begingroup$
@ceilingcat Clever (ab)use ofc[1]
! Updated.
$endgroup$
– Neil A.
yesterday
add a comment |
$begingroup$
C++ (gcc), 294 289 bytes
-5 bytes thanks to @ceilingcat
#import<iostream>
#import<queue>
void a(char*e,char*p){std::queue<char>d;for(;*e;)d.push(*e++);for(char*c=p;d.size();c=*++c?c:p){*c-49?d.pop():d.front()-48?d.push(c[1]?c[1]:*p):a("","");if(d.size()){for(int i=0;i++<d.size();d.pop())std::cout<<d.front(),d.push(d.front());std::cout<<" ";}}}
Try it online!
Fairly straightforward algorithm. Copies the data into a queue, and repeatedly loops through the program. On a "0", it removes the first element in the queue (the first "bit"). On a 1, it adds the next "bit" of the program to the data if the first "bit" of the data is 1. Then it loops through the data, printing it "bit" by "bit", and finally prints a space to separate successive data entries.
$endgroup$
C++ (gcc), 294 289 bytes
-5 bytes thanks to @ceilingcat
#import<iostream>
#import<queue>
void a(char*e,char*p){std::queue<char>d;for(;*e;)d.push(*e++);for(char*c=p;d.size();c=*++c?c:p){*c-49?d.pop():d.front()-48?d.push(c[1]?c[1]:*p):a("","");if(d.size()){for(int i=0;i++<d.size();d.pop())std::cout<<d.front(),d.push(d.front());std::cout<<" ";}}}
Try it online!
Fairly straightforward algorithm. Copies the data into a queue, and repeatedly loops through the program. On a "0", it removes the first element in the queue (the first "bit"). On a 1, it adds the next "bit" of the program to the data if the first "bit" of the data is 1. Then it loops through the data, printing it "bit" by "bit", and finally prints a space to separate successive data entries.
edited yesterday
answered 2 days ago


Neil A.Neil A.
1,358120
1,358120
$begingroup$
@ceilingcat Clever (ab)use ofc[1]
! Updated.
$endgroup$
– Neil A.
yesterday
add a comment |
$begingroup$
@ceilingcat Clever (ab)use ofc[1]
! Updated.
$endgroup$
– Neil A.
yesterday
$begingroup$
@ceilingcat Clever (ab)use of
c[1]
! Updated.$endgroup$
– Neil A.
yesterday
$begingroup$
@ceilingcat Clever (ab)use of
c[1]
! Updated.$endgroup$
– Neil A.
yesterday
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f182788%2fsimulate-bitwise-cyclic-tag%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8AmlbXcFu8FrD1srtJnrCua9nGJaIb2C0N 62 l,7ANTUSGUSgeJRL cdsy5H,E6f1lJ,8irdIu,isZXmz
$begingroup$
@Sanchises Seems like a borderline duplicate to that, but you have to get the result at a certain generation and that is for any cyclic tag system.
$endgroup$
– MilkyWay90
Apr 7 at 17:49
$begingroup$
in the first test case,
100
goes to10
on cmd0
, whose definition is "delete the left-most bit in the data." wouldn't the leftmost bit of100
be1
?$endgroup$
– Jonah
Apr 7 at 17:52
$begingroup$
@Jonah Oh, missed that
$endgroup$
– MilkyWay90
Apr 7 at 17:55
$begingroup$
in case (b), if you do the append, does the instruction pointer move right one or two characters?
$endgroup$
– Sparr
Apr 7 at 18:08
$begingroup$
@Sparr It moves right one. See the section labeled Challenge.
$endgroup$
– MilkyWay90
Apr 7 at 18:11