ASCII art H trees
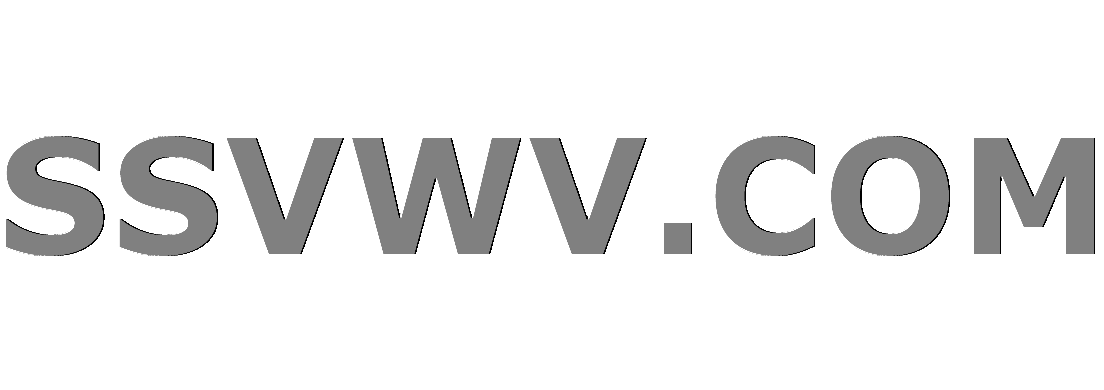
Multi tool use
up vote
6
down vote
favorite
An H tree is a fractal tree structure that starts with a line. In each iteration, T branches are added to all endpoints. In this challenge, you have to create an ASCII representation of every second H tree level.
The first level simply contains three hyphen-minus characters:
---
The next levels are constructed recursively:
- Create a 2x2 matrix of copies from the previous level, separated by three spaces or lines.
- Connect the centers of the copies with ASCII art lines in the form of an H. Use
-
for horizontal lines,|
for vertical lines, and+
whenever lines meet each other.
Second level
-+- -+-
| |
+-----+
| |
-+- -+-
Third level
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
Rules
- Input is an integer representing the level of the ASCII art H tree as described above (not the actual H tree level), either zero- or one-indexed.
- Output is flexible. For example, you can print the result or return a newline-separated string, a list of strings for each line, or a 2D array of characters.
- You must use
-
,|
,+
and space characters. - Trailing space and up to three trailing white-space lines are allowed.
This is code golf. The shortest answer in bytes wins.
code-golf ascii-art fractal
add a comment |
up vote
6
down vote
favorite
An H tree is a fractal tree structure that starts with a line. In each iteration, T branches are added to all endpoints. In this challenge, you have to create an ASCII representation of every second H tree level.
The first level simply contains three hyphen-minus characters:
---
The next levels are constructed recursively:
- Create a 2x2 matrix of copies from the previous level, separated by three spaces or lines.
- Connect the centers of the copies with ASCII art lines in the form of an H. Use
-
for horizontal lines,|
for vertical lines, and+
whenever lines meet each other.
Second level
-+- -+-
| |
+-----+
| |
-+- -+-
Third level
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
Rules
- Input is an integer representing the level of the ASCII art H tree as described above (not the actual H tree level), either zero- or one-indexed.
- Output is flexible. For example, you can print the result or return a newline-separated string, a list of strings for each line, or a 2D array of characters.
- You must use
-
,|
,+
and space characters. - Trailing space and up to three trailing white-space lines are allowed.
This is code golf. The shortest answer in bytes wins.
code-golf ascii-art fractal
2
Related: Create an “H” from smaller “H”s
– nwellnhof
16 hours ago
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
An H tree is a fractal tree structure that starts with a line. In each iteration, T branches are added to all endpoints. In this challenge, you have to create an ASCII representation of every second H tree level.
The first level simply contains three hyphen-minus characters:
---
The next levels are constructed recursively:
- Create a 2x2 matrix of copies from the previous level, separated by three spaces or lines.
- Connect the centers of the copies with ASCII art lines in the form of an H. Use
-
for horizontal lines,|
for vertical lines, and+
whenever lines meet each other.
Second level
-+- -+-
| |
+-----+
| |
-+- -+-
Third level
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
Rules
- Input is an integer representing the level of the ASCII art H tree as described above (not the actual H tree level), either zero- or one-indexed.
- Output is flexible. For example, you can print the result or return a newline-separated string, a list of strings for each line, or a 2D array of characters.
- You must use
-
,|
,+
and space characters. - Trailing space and up to three trailing white-space lines are allowed.
This is code golf. The shortest answer in bytes wins.
code-golf ascii-art fractal
An H tree is a fractal tree structure that starts with a line. In each iteration, T branches are added to all endpoints. In this challenge, you have to create an ASCII representation of every second H tree level.
The first level simply contains three hyphen-minus characters:
---
The next levels are constructed recursively:
- Create a 2x2 matrix of copies from the previous level, separated by three spaces or lines.
- Connect the centers of the copies with ASCII art lines in the form of an H. Use
-
for horizontal lines,|
for vertical lines, and+
whenever lines meet each other.
Second level
-+- -+-
| |
+-----+
| |
-+- -+-
Third level
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
Rules
- Input is an integer representing the level of the ASCII art H tree as described above (not the actual H tree level), either zero- or one-indexed.
- Output is flexible. For example, you can print the result or return a newline-separated string, a list of strings for each line, or a 2D array of characters.
- You must use
-
,|
,+
and space characters. - Trailing space and up to three trailing white-space lines are allowed.
This is code golf. The shortest answer in bytes wins.
code-golf ascii-art fractal
code-golf ascii-art fractal
asked 17 hours ago
nwellnhof
6,2681125
6,2681125
2
Related: Create an “H” from smaller “H”s
– nwellnhof
16 hours ago
add a comment |
2
Related: Create an “H” from smaller “H”s
– nwellnhof
16 hours ago
2
2
Related: Create an “H” from smaller “H”s
– nwellnhof
16 hours ago
Related: Create an “H” from smaller “H”s
– nwellnhof
16 hours ago
add a comment |
2 Answers
2
active
oldest
votes
up vote
6
down vote
Charcoal, 22 bytes
P-²FNF²«⟲T²+×⁺²κX²ι←‖O
Try it online! Link is to verbose version of code. 0-indexed. Explanation:
P-²
Print the initial three -
s, leaving the cursor in the middle.
FN
Repeat for the number of times given.
F²«
Repeat twice for each H
. Each loop creates a slightly bigger H
from the previous loop, but we only want alternate H
s.
⟲T²
Rotate the figure.
+×⁺²κX²ι←
Draw half of the next line.
‖O
Reflect to complete the step.
The result at each iteration is as follows:
---
| |
+---+
| |
-+- -+-
| |
+-----+
| |
-+- -+-
| | | |
+-+-+ +-+-+
| | | | | |
| |
+-------+
| |
| | | | | |
+-+-+ +-+-+
| | | |
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
If you wonder how a 5-th levelH
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png
– Paul
10 hours ago
add a comment |
up vote
4
down vote
Canvas, 20 19 bytes
ø⁸«╵[↷L⇵;l⇵└┌├-×╋‼│
Try it here!
Explanation:
ø push an empty canvas
⁸«╵[ repeat input*2 + 1 times
↷ rotate clockwise
L⇵ ceil(width/2)
;l⇵ ceil(height/2); leaves stack as [ ⌈½w⌉, canvas, ⌈½h⌉ ]
└┌ reorder stack to [ canvas, ⌈½w⌉, ⌈½h⌉, ⌈½w⌉ ]
├ add 2 to the top ⌈w÷2⌉
-× "-" * (2 + ⌈w÷2⌉)
╋ in the canvas, at (⌈w÷2⌉; ⌈h÷2⌉) insert the dashes
‼ normalize the canvas (the 0th iteration inserts at (0; 0) breaking things)
│ and palindromize horizontally
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
6
down vote
Charcoal, 22 bytes
P-²FNF²«⟲T²+×⁺²κX²ι←‖O
Try it online! Link is to verbose version of code. 0-indexed. Explanation:
P-²
Print the initial three -
s, leaving the cursor in the middle.
FN
Repeat for the number of times given.
F²«
Repeat twice for each H
. Each loop creates a slightly bigger H
from the previous loop, but we only want alternate H
s.
⟲T²
Rotate the figure.
+×⁺²κX²ι←
Draw half of the next line.
‖O
Reflect to complete the step.
The result at each iteration is as follows:
---
| |
+---+
| |
-+- -+-
| |
+-----+
| |
-+- -+-
| | | |
+-+-+ +-+-+
| | | | | |
| |
+-------+
| |
| | | | | |
+-+-+ +-+-+
| | | |
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
If you wonder how a 5-th levelH
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png
– Paul
10 hours ago
add a comment |
up vote
6
down vote
Charcoal, 22 bytes
P-²FNF²«⟲T²+×⁺²κX²ι←‖O
Try it online! Link is to verbose version of code. 0-indexed. Explanation:
P-²
Print the initial three -
s, leaving the cursor in the middle.
FN
Repeat for the number of times given.
F²«
Repeat twice for each H
. Each loop creates a slightly bigger H
from the previous loop, but we only want alternate H
s.
⟲T²
Rotate the figure.
+×⁺²κX²ι←
Draw half of the next line.
‖O
Reflect to complete the step.
The result at each iteration is as follows:
---
| |
+---+
| |
-+- -+-
| |
+-----+
| |
-+- -+-
| | | |
+-+-+ +-+-+
| | | | | |
| |
+-------+
| |
| | | | | |
+-+-+ +-+-+
| | | |
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
If you wonder how a 5-th levelH
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png
– Paul
10 hours ago
add a comment |
up vote
6
down vote
up vote
6
down vote
Charcoal, 22 bytes
P-²FNF²«⟲T²+×⁺²κX²ι←‖O
Try it online! Link is to verbose version of code. 0-indexed. Explanation:
P-²
Print the initial three -
s, leaving the cursor in the middle.
FN
Repeat for the number of times given.
F²«
Repeat twice for each H
. Each loop creates a slightly bigger H
from the previous loop, but we only want alternate H
s.
⟲T²
Rotate the figure.
+×⁺²κX²ι←
Draw half of the next line.
‖O
Reflect to complete the step.
The result at each iteration is as follows:
---
| |
+---+
| |
-+- -+-
| |
+-----+
| |
-+- -+-
| | | |
+-+-+ +-+-+
| | | | | |
| |
+-------+
| |
| | | | | |
+-+-+ +-+-+
| | | |
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
Charcoal, 22 bytes
P-²FNF²«⟲T²+×⁺²κX²ι←‖O
Try it online! Link is to verbose version of code. 0-indexed. Explanation:
P-²
Print the initial three -
s, leaving the cursor in the middle.
FN
Repeat for the number of times given.
F²«
Repeat twice for each H
. Each loop creates a slightly bigger H
from the previous loop, but we only want alternate H
s.
⟲T²
Rotate the figure.
+×⁺²κX²ι←
Draw half of the next line.
‖O
Reflect to complete the step.
The result at each iteration is as follows:
---
| |
+---+
| |
-+- -+-
| |
+-----+
| |
-+- -+-
| | | |
+-+-+ +-+-+
| | | | | |
| |
+-------+
| |
| | | | | |
+-+-+ +-+-+
| | | |
-+- -+- -+- -+-
| | | |
+--+--+ +--+--+
| | | | | |
-+- | -+- -+- | -+-
| |
+-----------+
| |
-+- | -+- -+- | -+-
| | | | | |
+--+--+ +--+--+
| | | |
-+- -+- -+- -+-
answered 16 hours ago
Neil
78.2k744175
78.2k744175
If you wonder how a 5-th levelH
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png
– Paul
10 hours ago
add a comment |
If you wonder how a 5-th levelH
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png
– Paul
10 hours ago
If you wonder how a 5-th level
H
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png– Paul
10 hours ago
If you wonder how a 5-th level
H
looks like, a quick zoomed-out glance: i.imgur.com/EGapcrS.png– Paul
10 hours ago
add a comment |
up vote
4
down vote
Canvas, 20 19 bytes
ø⁸«╵[↷L⇵;l⇵└┌├-×╋‼│
Try it here!
Explanation:
ø push an empty canvas
⁸«╵[ repeat input*2 + 1 times
↷ rotate clockwise
L⇵ ceil(width/2)
;l⇵ ceil(height/2); leaves stack as [ ⌈½w⌉, canvas, ⌈½h⌉ ]
└┌ reorder stack to [ canvas, ⌈½w⌉, ⌈½h⌉, ⌈½w⌉ ]
├ add 2 to the top ⌈w÷2⌉
-× "-" * (2 + ⌈w÷2⌉)
╋ in the canvas, at (⌈w÷2⌉; ⌈h÷2⌉) insert the dashes
‼ normalize the canvas (the 0th iteration inserts at (0; 0) breaking things)
│ and palindromize horizontally
add a comment |
up vote
4
down vote
Canvas, 20 19 bytes
ø⁸«╵[↷L⇵;l⇵└┌├-×╋‼│
Try it here!
Explanation:
ø push an empty canvas
⁸«╵[ repeat input*2 + 1 times
↷ rotate clockwise
L⇵ ceil(width/2)
;l⇵ ceil(height/2); leaves stack as [ ⌈½w⌉, canvas, ⌈½h⌉ ]
└┌ reorder stack to [ canvas, ⌈½w⌉, ⌈½h⌉, ⌈½w⌉ ]
├ add 2 to the top ⌈w÷2⌉
-× "-" * (2 + ⌈w÷2⌉)
╋ in the canvas, at (⌈w÷2⌉; ⌈h÷2⌉) insert the dashes
‼ normalize the canvas (the 0th iteration inserts at (0; 0) breaking things)
│ and palindromize horizontally
add a comment |
up vote
4
down vote
up vote
4
down vote
Canvas, 20 19 bytes
ø⁸«╵[↷L⇵;l⇵└┌├-×╋‼│
Try it here!
Explanation:
ø push an empty canvas
⁸«╵[ repeat input*2 + 1 times
↷ rotate clockwise
L⇵ ceil(width/2)
;l⇵ ceil(height/2); leaves stack as [ ⌈½w⌉, canvas, ⌈½h⌉ ]
└┌ reorder stack to [ canvas, ⌈½w⌉, ⌈½h⌉, ⌈½w⌉ ]
├ add 2 to the top ⌈w÷2⌉
-× "-" * (2 + ⌈w÷2⌉)
╋ in the canvas, at (⌈w÷2⌉; ⌈h÷2⌉) insert the dashes
‼ normalize the canvas (the 0th iteration inserts at (0; 0) breaking things)
│ and palindromize horizontally
Canvas, 20 19 bytes
ø⁸«╵[↷L⇵;l⇵└┌├-×╋‼│
Try it here!
Explanation:
ø push an empty canvas
⁸«╵[ repeat input*2 + 1 times
↷ rotate clockwise
L⇵ ceil(width/2)
;l⇵ ceil(height/2); leaves stack as [ ⌈½w⌉, canvas, ⌈½h⌉ ]
└┌ reorder stack to [ canvas, ⌈½w⌉, ⌈½h⌉, ⌈½w⌉ ]
├ add 2 to the top ⌈w÷2⌉
-× "-" * (2 + ⌈w÷2⌉)
╋ in the canvas, at (⌈w÷2⌉; ⌈h÷2⌉) insert the dashes
‼ normalize the canvas (the 0th iteration inserts at (0; 0) breaking things)
│ and palindromize horizontally
edited 12 hours ago
answered 16 hours ago


dzaima
14.1k21754
14.1k21754
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f176521%2fascii-art-h-trees%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DLwpUJgZAkZATTsiIslvkuHFw191,0rkgbMrbthx,C0ESRCn
2
Related: Create an “H” from smaller “H”s
– nwellnhof
16 hours ago