Deal the cards to the players
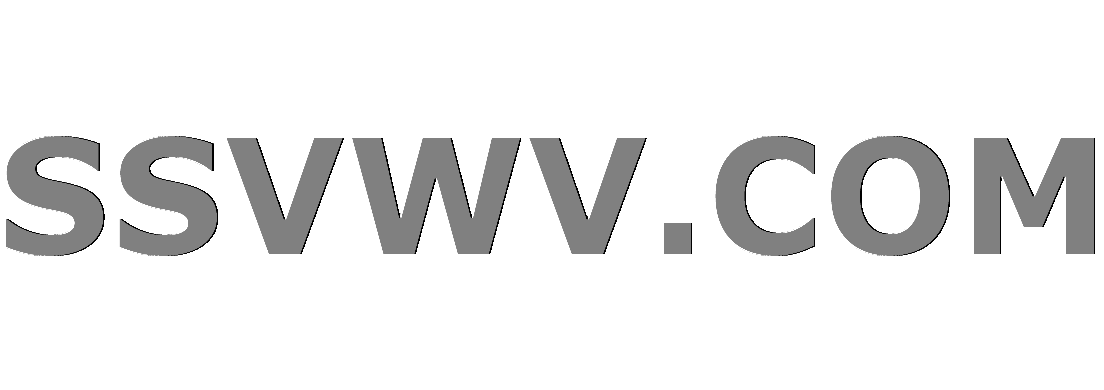
Multi tool use
$begingroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of card and a number of player, you need to split the array into hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands, if the length of the string isn't divisible by n
any leftover at the end should be distributed as evenly as possible.
- If
n
is equals to 1, you will need to return an array of arrayA
If
n
is greater than the length ofA
, you will need to return every hands and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change order (or direction) of any item from left to right. For example
if n = 2
andA= [1,2,3]
. Any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],]
Demo
def deal(cards, n):
i = 0
players = [ for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
$endgroup$
|
show 8 more comments
$begingroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of card and a number of player, you need to split the array into hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands, if the length of the string isn't divisible by n
any leftover at the end should be distributed as evenly as possible.
- If
n
is equals to 1, you will need to return an array of arrayA
If
n
is greater than the length ofA
, you will need to return every hands and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change order (or direction) of any item from left to right. For example
if n = 2
andA= [1,2,3]
. Any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],]
Demo
def deal(cards, n):
i = 0
players = [ for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
$endgroup$
1
$begingroup$
All the test cases seem to splitA
inton
chunks rather than into chunks of sizen
.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
2 hours ago
1
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
2 hours ago
3
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
2 hours ago
|
show 8 more comments
$begingroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of card and a number of player, you need to split the array into hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands, if the length of the string isn't divisible by n
any leftover at the end should be distributed as evenly as possible.
- If
n
is equals to 1, you will need to return an array of arrayA
If
n
is greater than the length ofA
, you will need to return every hands and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change order (or direction) of any item from left to right. For example
if n = 2
andA= [1,2,3]
. Any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],]
Demo
def deal(cards, n):
i = 0
players = [ for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
$endgroup$
Tonight is card game night! You are the dealer and your task is to write a program to deal the cards to the players.
Given an array of card and a number of player, you need to split the array into hand for each player.
Rules
Your program will receive an non-empty array A
, as well as a non-zero positive integer n
. The array should then be split into n
hands, if the length of the string isn't divisible by n
any leftover at the end should be distributed as evenly as possible.
- If
n
is equals to 1, you will need to return an array of arrayA
If
n
is greater than the length ofA
, you will need to return every hands and an empty hand. ifn = 4
andarray A = [1,2,3]
, you should return[[1],[2],[3]]
or[[1],[2],[3],]
. You are free to handle the empty hand with empty, undefined or null.The array can contain any type rather than a number.
You should not change order (or direction) of any item from left to right. For example
if n = 2
andA= [1,2,3]
. Any result rather than[[1,3],[2]]
will be invalid.
Test Cases
n A Output
1 [1,2,3,4,5,6] [[1,2,3,4,5,6]]
2 [1,2,3,4,5,6] [[1,3,5],[2,4,6]]
3 [1,2,3,4,5,6] [[1,4],[2,5],[3,6]]
4 [1,2,3,4,5,6] [[1,5],[2,6],[3],[4]]
7 [1,2,3,4,5,6] [[1],[2],[3],[4],[5],[6]] // or [[1],[2],[3],[4],[5],[6],]
Demo
def deal(cards, n):
i = 0
players = [ for _ in range(n)]
for card in cards:
players[i % n].append(card)
i += 1
return players
hands = deal([1,2,3,4,5,6], 2)
print(hands)
Try it online!
This is code-golf, so you the shortest bytes of each language will be the winner.
Inspired from Create chunks from array by chau giang
code-golf array-manipulation
code-golf array-manipulation
edited 2 hours ago
aloisdg
asked 3 hours ago
aloisdgaloisdg
1,5041122
1,5041122
1
$begingroup$
All the test cases seem to splitA
inton
chunks rather than into chunks of sizen
.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
2 hours ago
1
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
2 hours ago
3
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
2 hours ago
|
show 8 more comments
1
$begingroup$
All the test cases seem to splitA
inton
chunks rather than into chunks of sizen
.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
2 hours ago
1
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
2 hours ago
3
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
2 hours ago
1
1
$begingroup$
All the test cases seem to split
A
into n
chunks rather than into chunks of size n
.$endgroup$
– Adám
2 hours ago
$begingroup$
All the test cases seem to split
A
into n
chunks rather than into chunks of size n
.$endgroup$
– Adám
2 hours ago
1
1
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
2 hours ago
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
2 hours ago
1
1
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
2 hours ago
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
2 hours ago
1
1
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
2 hours ago
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
2 hours ago
3
3
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
2 hours ago
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
2 hours ago
|
show 8 more comments
10 Answers
10
active
oldest
votes
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
add a comment |
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
1 hour ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
add a comment |
$begingroup$
Jelly, 6 bytes
sz0ḟ€0
Try it online!
$endgroup$
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181029%2fdeal-the-cards-to-the-players%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
10 Answers
10
active
oldest
votes
10 Answers
10
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
add a comment |
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
add a comment |
$begingroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
$endgroup$
Japt, 2 bytes
Takes the array as the first input.
óV
Try it
answered 2 hours ago


ShaggyShaggy
19.4k21667
19.4k21667
add a comment |
add a comment |
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
add a comment |
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
add a comment |
$begingroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
$endgroup$
R, 46 25 bytes
function(A,n)split(A,1:n)
Try it online!
split
s A
into groups defined by 1:n
, recycling 1:n
until it matches length with A
.
edited 2 hours ago
answered 3 hours ago
GiuseppeGiuseppe
16.6k31052
16.6k31052
add a comment |
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
$endgroup$
Python 2, 37 bytes
Code:
lambda x,n:[x[i::n]for i in range(n)]
Try it online!
answered 2 hours ago


AdnanAdnan
35.7k562225
35.7k562225
add a comment |
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
add a comment |
$begingroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
$endgroup$
Perl 6, 33 24 bytes
->b{*.classify:{$++%b}}
Try it online!
Anonymous curried code block that takes a number and returns a Whatever lambda that takes a list and returns a list of lists. This takes the second option when given a number larger than the length of lists, e.g. f(4)([1,2,3])
returns [[1],[2],[3]]
Explanation:
->b{ } # Anonymous code block that takes a number
* # And returns a Whatever lambda
.classify # That groups by
:{$++%b} # The index modulo the number
edited 2 hours ago
answered 3 hours ago
Jo KingJo King
24.3k357126
24.3k357126
add a comment |
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
add a comment |
$begingroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
$endgroup$
Charcoal, 9 bytes
IEθ✂ηιLηθ
Try it online! Link is to verbose version of code. Takes input in the order [n, A]
and outputs each value on its own line and each hand double-spaced from the previous. Explanation:
θ First input `n`
E Map over implicit range
η Second input `A`
✂ Sliced
ι Starting at current index
Lη Ending at length of `A`
θ Taking every `n`th element
I Cast to string
Implicitly print
answered 2 hours ago
NeilNeil
81.4k745178
81.4k745178
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
add a comment |
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
$begingroup$
+1 for making the symbol of "slice" a scissors!
$endgroup$
– Jonah
56 mins ago
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
add a comment |
$begingroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
$endgroup$
Wolfram Language (Mathematica), 28 bytes
(s=#;GatherBy[#2,#~Mod~s&])&
Try it online!
answered 1 hour ago


J42161217J42161217
13.2k21150
13.2k21150
add a comment |
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
1 hour ago
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
1 hour ago
add a comment |
$begingroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
$endgroup$
Haskell, 39 bytes
import Data.Lists
(transpose.).chunksOf
Note: Data.Lists
is from the third-party library lists, which is not on Stackage and hence will not appear on Hoogle.
edited 1 hour ago


Joseph Sible
2075
2075
answered 1 hour ago


dfeuerdfeuer
36128
36128
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
1 hour ago
add a comment |
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meantData.List
, but it doesn't containchunksOf
.
$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signatureInt -> Text -> [Text]
.1
$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
@JosephSible, it's in thelists
package.
$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in thesplit
package and re-exported by thelists
package. There are versions ofchunksOf
for lists, text, sequences, and probably other things.
$endgroup$
– dfeuer
1 hour ago
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meant Data.List
, but it doesn't contain chunksOf
.$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
Data.Lists
doesn't seem to exist. I would assume that you meant Data.List
, but it doesn't contain chunksOf
.$endgroup$
– Joseph Sible
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signature Int -> Text -> [Text]
.1$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
chunksOf
only seems to appear with the signature Int -> Text -> [Text]
.1$endgroup$
– Sriotchilism O'Zaic
1 hour ago
$begingroup$
@JosephSible, it's in the
lists
package.$endgroup$
– dfeuer
1 hour ago
$begingroup$
@JosephSible, it's in the
lists
package.$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in the
split
package and re-exported by the lists
package. There are versions of chunksOf
for lists, text, sequences, and probably other things.$endgroup$
– dfeuer
1 hour ago
$begingroup$
@SriotchilismO'Zaic, lots of things don't show up in Hoogle. It's in the
split
package and re-exported by the lists
package. There are versions of chunksOf
for lists, text, sequences, and probably other things.$endgroup$
– dfeuer
1 hour ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 43 bytes
a=>b=>{int i=0;return a.GroupBy(_=>i++%b);}
Try it online!
answered 3 hours ago
aloisdgaloisdg
1,5041122
1,5041122
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
add a comment |
$begingroup$
@JoKing[1,2,3], 4
should output[[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@JoKing
[1,2,3], 4
should output [[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.$endgroup$
– aloisdg
2 hours ago
$begingroup$
@JoKing
[1,2,3], 4
should output [[1],[2],[3]]
. You are dealing 3 cards to 4 players. I will update the main question.$endgroup$
– aloisdg
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
$begingroup$
It's generally discouraged to post solutions to your own challenges immediately.
$endgroup$
– Shaggy
2 hours ago
1
1
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Shaggy ok I will take it into account for the next time. It is fine on so and rpg but I guess the competitive aspect of codegolf made it a bit unfair to self post directly. Make sense.
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
$begingroup$
@Joe king you are right! I made a typo :/
$endgroup$
– aloisdg
2 hours ago
add a comment |
$begingroup$
Jelly, 6 bytes
sz0ḟ€0
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 6 bytes
sz0ḟ€0
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 6 bytes
sz0ḟ€0
Try it online!
$endgroup$
Jelly, 6 bytes
sz0ḟ€0
Try it online!
answered 1 hour ago
Nick KennedyNick Kennedy
44125
44125
add a comment |
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
add a comment |
$begingroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
$endgroup$
J, 13, 11, 10, 9 bytes
(|#)</.]
Try it online!
how (previous explanation, fundamentally the same)
] </.~ (| #)
</.~ NB. box results of grouping
] NB. the right arg by...
| NB. the remainders of dividing...
[ NB. the left arg into...
# NB. the length of each prefix of...
] NB. the right arg,
NB. aka, the integers 1 thru
NB. the length of the right arg
edited 29 mins ago
answered 1 hour ago


JonahJonah
2,4011016
2,4011016
add a comment |
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181029%2fdeal-the-cards-to-the-players%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VlVaZ IIAMN6XmczB3 g7aCMtD NF sj11RA,Uc,u CK7wDL3rQMkv0KaG iTi 92Srf1CX8rqNopOlPd
1
$begingroup$
All the test cases seem to split
A
inton
chunks rather than into chunks of sizen
.$endgroup$
– Adám
2 hours ago
1
$begingroup$
Are the cards guaranteed to be positive integers?
$endgroup$
– Giuseppe
2 hours ago
1
$begingroup$
should be give to a player as much as possible means should be distributed as evenly as possible, right? If so, please edit to actually say so.
$endgroup$
– Adám
2 hours ago
1
$begingroup$
@aloisdg Great, but now your first bulleted rule is unnecessary and wrong.
$endgroup$
– Adám
2 hours ago
3
$begingroup$
In the future I'd recommend using the Sandbox to iron out problems and gauge community feedback before posting your question to main
$endgroup$
– Jo King
2 hours ago