The Digit Triangles
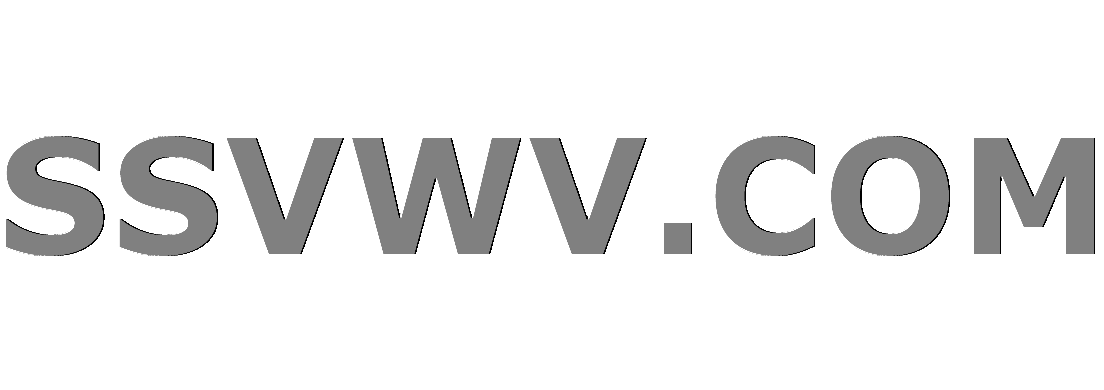
Multi tool use
$begingroup$
Challenge:
Input: A positive integer $n$
Output:
Create a list in the range $[1,n]$, and join it together to a string (i.e. $n=13$ would be the string 12345678910111213
).
Now we output a triangle using the prefixes or suffixes of this string, in one of the following four orientations based on the input integer:
- If $nequiv 0pmod 4$, output it in the triangle shape ◣
- If $nequiv 1pmod 4$, output it in the triangle shape ◤
- If $nequiv 2pmod 4$, output it in the triangle shape ◥
- If $nequiv 3pmod 4$, output it in the triangle shape ◢
Example:
Input: $n=13$
Because $13equiv 1pmod 4$, the shape will be ◤. Here three possible valid outputs:
12345678910111213 11111111111111111 12345678910111213
1234567891011121 2222222222222222 2345678910111213
123456789101112 333333333333333 345678910111213
12345678910111 44444444444444 45678910111213
1234567891011 5555555555555 5678910111213
123456789101 666666666666 678910111213
12345678910 77777777777 78910111213
1234567891 8888888888 8910111213
123456789 999999999 910111213
12345678 11111111 10111213
1234567 0000000 0111213
123456 111111 111213
12345 11111 11213
1234 1111 1213
123 222 213
12 11 13
1 3 3
Challenge rules:
- As you can see at the three valid outputs above, only the correct shape and using all the digits in the correct order is important. Apart from that you're free to use prefixes or suffixes; reverses/reflects; diagonal printing; etc. etc. Any of the six possible outputs for each shape is allowed (see the test case below to see all valid outputs based on the shape). This allows languages with rotation builtins to use it, but those without can also use an alternative approach of using the prefixes in the correct size from top-to-bottom, or using the prefixes for two of the shapes but suffixes for the other two shapes. Choosing the most appropriate output options for your language is part of the golfing process. :)
- Input is guaranteed to be a positive integer. For $n=1$ we simply output
1
. - Any amount of leading/trailing newlines/spaces are allowed, as long as it prints the correct triangle (without vertical nor horizontal delimiters!) somewhere on the screen.
General rules:
- This is code-golf, so shortest answer in bytes wins.
Don't let code-golf languages discourage you from posting answers with non-codegolfing languages. Try to come up with an as short as possible answer for 'any' programming language.
Standard rules apply for your answer with default I/O rules, so you are allowed to use STDIN/STDOUT, functions/method with the proper parameters and return-type, full programs. Your call.
Default Loopholes are forbidden.- If possible, please add a link with a test for your code (i.e. TIO).
- Also, adding an explanation for your answer is highly recommended.
Test cases:
Input: $n=5$
All possible valid outputs:
12345 54321 12345 54321 11111 55555
1234 5432 2345 4321 2222 4444
123 543 345 321 333 333
12 54 45 21 44 22
1 5 5 1 5 1
Input: $n=6$
All possible outputs:
123456 654321 123456 654321 111111 666666
12345 65432 23456 54321 22222 55555
1234 6543 3456 4321 3333 4444
123 654 456 321 444 333
12 65 56 21 55 22
1 6 6 1 6 1
Input: $n=7$
All possible outputs:
1 1 7 7 7 1
12 21 67 76 66 22
123 321 567 765 555 333
1234 4321 4567 7654 4444 4444
12345 54321 34567 76543 33333 55555
123456 654321 234567 765432 222222 666666
1234567 7654321 1234567 7654321 1111111 7777777
Input: $n=8$
All possible outputs:
1 1 8 8 8 1
12 21 78 87 77 22
123 321 678 876 666 333
1234 4321 5678 8765 5555 4444
12345 54321 45678 87654 44444 55555
123456 654321 345678 876543 333333 666666
1234567 7654321 2345678 8765432 2222222 7777777
12345678 87654321 12345678 87654321 11111111 88888888
Input: $n=1$
Only possible output:
1
Input: $n=2$
All possible outputs:
12 21 12 21 11 22
1 2 2 1 2 1
code-golf ascii-art number integer
$endgroup$
add a comment |
$begingroup$
Challenge:
Input: A positive integer $n$
Output:
Create a list in the range $[1,n]$, and join it together to a string (i.e. $n=13$ would be the string 12345678910111213
).
Now we output a triangle using the prefixes or suffixes of this string, in one of the following four orientations based on the input integer:
- If $nequiv 0pmod 4$, output it in the triangle shape ◣
- If $nequiv 1pmod 4$, output it in the triangle shape ◤
- If $nequiv 2pmod 4$, output it in the triangle shape ◥
- If $nequiv 3pmod 4$, output it in the triangle shape ◢
Example:
Input: $n=13$
Because $13equiv 1pmod 4$, the shape will be ◤. Here three possible valid outputs:
12345678910111213 11111111111111111 12345678910111213
1234567891011121 2222222222222222 2345678910111213
123456789101112 333333333333333 345678910111213
12345678910111 44444444444444 45678910111213
1234567891011 5555555555555 5678910111213
123456789101 666666666666 678910111213
12345678910 77777777777 78910111213
1234567891 8888888888 8910111213
123456789 999999999 910111213
12345678 11111111 10111213
1234567 0000000 0111213
123456 111111 111213
12345 11111 11213
1234 1111 1213
123 222 213
12 11 13
1 3 3
Challenge rules:
- As you can see at the three valid outputs above, only the correct shape and using all the digits in the correct order is important. Apart from that you're free to use prefixes or suffixes; reverses/reflects; diagonal printing; etc. etc. Any of the six possible outputs for each shape is allowed (see the test case below to see all valid outputs based on the shape). This allows languages with rotation builtins to use it, but those without can also use an alternative approach of using the prefixes in the correct size from top-to-bottom, or using the prefixes for two of the shapes but suffixes for the other two shapes. Choosing the most appropriate output options for your language is part of the golfing process. :)
- Input is guaranteed to be a positive integer. For $n=1$ we simply output
1
. - Any amount of leading/trailing newlines/spaces are allowed, as long as it prints the correct triangle (without vertical nor horizontal delimiters!) somewhere on the screen.
General rules:
- This is code-golf, so shortest answer in bytes wins.
Don't let code-golf languages discourage you from posting answers with non-codegolfing languages. Try to come up with an as short as possible answer for 'any' programming language.
Standard rules apply for your answer with default I/O rules, so you are allowed to use STDIN/STDOUT, functions/method with the proper parameters and return-type, full programs. Your call.
Default Loopholes are forbidden.- If possible, please add a link with a test for your code (i.e. TIO).
- Also, adding an explanation for your answer is highly recommended.
Test cases:
Input: $n=5$
All possible valid outputs:
12345 54321 12345 54321 11111 55555
1234 5432 2345 4321 2222 4444
123 543 345 321 333 333
12 54 45 21 44 22
1 5 5 1 5 1
Input: $n=6$
All possible outputs:
123456 654321 123456 654321 111111 666666
12345 65432 23456 54321 22222 55555
1234 6543 3456 4321 3333 4444
123 654 456 321 444 333
12 65 56 21 55 22
1 6 6 1 6 1
Input: $n=7$
All possible outputs:
1 1 7 7 7 1
12 21 67 76 66 22
123 321 567 765 555 333
1234 4321 4567 7654 4444 4444
12345 54321 34567 76543 33333 55555
123456 654321 234567 765432 222222 666666
1234567 7654321 1234567 7654321 1111111 7777777
Input: $n=8$
All possible outputs:
1 1 8 8 8 1
12 21 78 87 77 22
123 321 678 876 666 333
1234 4321 5678 8765 5555 4444
12345 54321 45678 87654 44444 55555
123456 654321 345678 876543 333333 666666
1234567 7654321 2345678 8765432 2222222 7777777
12345678 87654321 12345678 87654321 11111111 88888888
Input: $n=1$
Only possible output:
1
Input: $n=2$
All possible outputs:
12 21 12 21 11 22
1 2 2 1 2 1
code-golf ascii-art number integer
$endgroup$
$begingroup$
Can we use other values for different triangles, like 1 for ◤, etc?
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
@EmbodimentofIgnorance Unfortunate example, since that's what the spec says. I think you wanted to ask if we can change the order of the four arrangements as long as we keep it consistent (I think that would be a no).
$endgroup$
– Erik the Outgolfer
yesterday
1
$begingroup$
Ifn==13
, can the topmost row be'33333333333333333'
(or, equivalently,'31211101987654321'
)?
$endgroup$
– Chas Brown
yesterday
$begingroup$
@EmbodimentofIgnorance Sorry, but I'd say no in this case. The shapes and their correspondingmod 4
are strict pairs for this challenge. So you may not switch the four shapes for the fourmod 4
cases. But good question nonetheless.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@ChasBrown Yes, both of those are fine. I only gave three possible examples for the $n=13$, but all six options (like the $n=5$ test case) are valid outputs.
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
$begingroup$
Challenge:
Input: A positive integer $n$
Output:
Create a list in the range $[1,n]$, and join it together to a string (i.e. $n=13$ would be the string 12345678910111213
).
Now we output a triangle using the prefixes or suffixes of this string, in one of the following four orientations based on the input integer:
- If $nequiv 0pmod 4$, output it in the triangle shape ◣
- If $nequiv 1pmod 4$, output it in the triangle shape ◤
- If $nequiv 2pmod 4$, output it in the triangle shape ◥
- If $nequiv 3pmod 4$, output it in the triangle shape ◢
Example:
Input: $n=13$
Because $13equiv 1pmod 4$, the shape will be ◤. Here three possible valid outputs:
12345678910111213 11111111111111111 12345678910111213
1234567891011121 2222222222222222 2345678910111213
123456789101112 333333333333333 345678910111213
12345678910111 44444444444444 45678910111213
1234567891011 5555555555555 5678910111213
123456789101 666666666666 678910111213
12345678910 77777777777 78910111213
1234567891 8888888888 8910111213
123456789 999999999 910111213
12345678 11111111 10111213
1234567 0000000 0111213
123456 111111 111213
12345 11111 11213
1234 1111 1213
123 222 213
12 11 13
1 3 3
Challenge rules:
- As you can see at the three valid outputs above, only the correct shape and using all the digits in the correct order is important. Apart from that you're free to use prefixes or suffixes; reverses/reflects; diagonal printing; etc. etc. Any of the six possible outputs for each shape is allowed (see the test case below to see all valid outputs based on the shape). This allows languages with rotation builtins to use it, but those without can also use an alternative approach of using the prefixes in the correct size from top-to-bottom, or using the prefixes for two of the shapes but suffixes for the other two shapes. Choosing the most appropriate output options for your language is part of the golfing process. :)
- Input is guaranteed to be a positive integer. For $n=1$ we simply output
1
. - Any amount of leading/trailing newlines/spaces are allowed, as long as it prints the correct triangle (without vertical nor horizontal delimiters!) somewhere on the screen.
General rules:
- This is code-golf, so shortest answer in bytes wins.
Don't let code-golf languages discourage you from posting answers with non-codegolfing languages. Try to come up with an as short as possible answer for 'any' programming language.
Standard rules apply for your answer with default I/O rules, so you are allowed to use STDIN/STDOUT, functions/method with the proper parameters and return-type, full programs. Your call.
Default Loopholes are forbidden.- If possible, please add a link with a test for your code (i.e. TIO).
- Also, adding an explanation for your answer is highly recommended.
Test cases:
Input: $n=5$
All possible valid outputs:
12345 54321 12345 54321 11111 55555
1234 5432 2345 4321 2222 4444
123 543 345 321 333 333
12 54 45 21 44 22
1 5 5 1 5 1
Input: $n=6$
All possible outputs:
123456 654321 123456 654321 111111 666666
12345 65432 23456 54321 22222 55555
1234 6543 3456 4321 3333 4444
123 654 456 321 444 333
12 65 56 21 55 22
1 6 6 1 6 1
Input: $n=7$
All possible outputs:
1 1 7 7 7 1
12 21 67 76 66 22
123 321 567 765 555 333
1234 4321 4567 7654 4444 4444
12345 54321 34567 76543 33333 55555
123456 654321 234567 765432 222222 666666
1234567 7654321 1234567 7654321 1111111 7777777
Input: $n=8$
All possible outputs:
1 1 8 8 8 1
12 21 78 87 77 22
123 321 678 876 666 333
1234 4321 5678 8765 5555 4444
12345 54321 45678 87654 44444 55555
123456 654321 345678 876543 333333 666666
1234567 7654321 2345678 8765432 2222222 7777777
12345678 87654321 12345678 87654321 11111111 88888888
Input: $n=1$
Only possible output:
1
Input: $n=2$
All possible outputs:
12 21 12 21 11 22
1 2 2 1 2 1
code-golf ascii-art number integer
$endgroup$
Challenge:
Input: A positive integer $n$
Output:
Create a list in the range $[1,n]$, and join it together to a string (i.e. $n=13$ would be the string 12345678910111213
).
Now we output a triangle using the prefixes or suffixes of this string, in one of the following four orientations based on the input integer:
- If $nequiv 0pmod 4$, output it in the triangle shape ◣
- If $nequiv 1pmod 4$, output it in the triangle shape ◤
- If $nequiv 2pmod 4$, output it in the triangle shape ◥
- If $nequiv 3pmod 4$, output it in the triangle shape ◢
Example:
Input: $n=13$
Because $13equiv 1pmod 4$, the shape will be ◤. Here three possible valid outputs:
12345678910111213 11111111111111111 12345678910111213
1234567891011121 2222222222222222 2345678910111213
123456789101112 333333333333333 345678910111213
12345678910111 44444444444444 45678910111213
1234567891011 5555555555555 5678910111213
123456789101 666666666666 678910111213
12345678910 77777777777 78910111213
1234567891 8888888888 8910111213
123456789 999999999 910111213
12345678 11111111 10111213
1234567 0000000 0111213
123456 111111 111213
12345 11111 11213
1234 1111 1213
123 222 213
12 11 13
1 3 3
Challenge rules:
- As you can see at the three valid outputs above, only the correct shape and using all the digits in the correct order is important. Apart from that you're free to use prefixes or suffixes; reverses/reflects; diagonal printing; etc. etc. Any of the six possible outputs for each shape is allowed (see the test case below to see all valid outputs based on the shape). This allows languages with rotation builtins to use it, but those without can also use an alternative approach of using the prefixes in the correct size from top-to-bottom, or using the prefixes for two of the shapes but suffixes for the other two shapes. Choosing the most appropriate output options for your language is part of the golfing process. :)
- Input is guaranteed to be a positive integer. For $n=1$ we simply output
1
. - Any amount of leading/trailing newlines/spaces are allowed, as long as it prints the correct triangle (without vertical nor horizontal delimiters!) somewhere on the screen.
General rules:
- This is code-golf, so shortest answer in bytes wins.
Don't let code-golf languages discourage you from posting answers with non-codegolfing languages. Try to come up with an as short as possible answer for 'any' programming language.
Standard rules apply for your answer with default I/O rules, so you are allowed to use STDIN/STDOUT, functions/method with the proper parameters and return-type, full programs. Your call.
Default Loopholes are forbidden.- If possible, please add a link with a test for your code (i.e. TIO).
- Also, adding an explanation for your answer is highly recommended.
Test cases:
Input: $n=5$
All possible valid outputs:
12345 54321 12345 54321 11111 55555
1234 5432 2345 4321 2222 4444
123 543 345 321 333 333
12 54 45 21 44 22
1 5 5 1 5 1
Input: $n=6$
All possible outputs:
123456 654321 123456 654321 111111 666666
12345 65432 23456 54321 22222 55555
1234 6543 3456 4321 3333 4444
123 654 456 321 444 333
12 65 56 21 55 22
1 6 6 1 6 1
Input: $n=7$
All possible outputs:
1 1 7 7 7 1
12 21 67 76 66 22
123 321 567 765 555 333
1234 4321 4567 7654 4444 4444
12345 54321 34567 76543 33333 55555
123456 654321 234567 765432 222222 666666
1234567 7654321 1234567 7654321 1111111 7777777
Input: $n=8$
All possible outputs:
1 1 8 8 8 1
12 21 78 87 77 22
123 321 678 876 666 333
1234 4321 5678 8765 5555 4444
12345 54321 45678 87654 44444 55555
123456 654321 345678 876543 333333 666666
1234567 7654321 2345678 8765432 2222222 7777777
12345678 87654321 12345678 87654321 11111111 88888888
Input: $n=1$
Only possible output:
1
Input: $n=2$
All possible outputs:
12 21 12 21 11 22
1 2 2 1 2 1
code-golf ascii-art number integer
code-golf ascii-art number integer
edited 6 hours ago
Kevin Cruijssen
asked yesterday


Kevin CruijssenKevin Cruijssen
41.1k566212
41.1k566212
$begingroup$
Can we use other values for different triangles, like 1 for ◤, etc?
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
@EmbodimentofIgnorance Unfortunate example, since that's what the spec says. I think you wanted to ask if we can change the order of the four arrangements as long as we keep it consistent (I think that would be a no).
$endgroup$
– Erik the Outgolfer
yesterday
1
$begingroup$
Ifn==13
, can the topmost row be'33333333333333333'
(or, equivalently,'31211101987654321'
)?
$endgroup$
– Chas Brown
yesterday
$begingroup$
@EmbodimentofIgnorance Sorry, but I'd say no in this case. The shapes and their correspondingmod 4
are strict pairs for this challenge. So you may not switch the four shapes for the fourmod 4
cases. But good question nonetheless.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@ChasBrown Yes, both of those are fine. I only gave three possible examples for the $n=13$, but all six options (like the $n=5$ test case) are valid outputs.
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
$begingroup$
Can we use other values for different triangles, like 1 for ◤, etc?
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
@EmbodimentofIgnorance Unfortunate example, since that's what the spec says. I think you wanted to ask if we can change the order of the four arrangements as long as we keep it consistent (I think that would be a no).
$endgroup$
– Erik the Outgolfer
yesterday
1
$begingroup$
Ifn==13
, can the topmost row be'33333333333333333'
(or, equivalently,'31211101987654321'
)?
$endgroup$
– Chas Brown
yesterday
$begingroup$
@EmbodimentofIgnorance Sorry, but I'd say no in this case. The shapes and their correspondingmod 4
are strict pairs for this challenge. So you may not switch the four shapes for the fourmod 4
cases. But good question nonetheless.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@ChasBrown Yes, both of those are fine. I only gave three possible examples for the $n=13$, but all six options (like the $n=5$ test case) are valid outputs.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Can we use other values for different triangles, like 1 for ◤, etc?
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
Can we use other values for different triangles, like 1 for ◤, etc?
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
@EmbodimentofIgnorance Unfortunate example, since that's what the spec says. I think you wanted to ask if we can change the order of the four arrangements as long as we keep it consistent (I think that would be a no).
$endgroup$
– Erik the Outgolfer
yesterday
$begingroup$
@EmbodimentofIgnorance Unfortunate example, since that's what the spec says. I think you wanted to ask if we can change the order of the four arrangements as long as we keep it consistent (I think that would be a no).
$endgroup$
– Erik the Outgolfer
yesterday
1
1
$begingroup$
If
n==13
, can the topmost row be '33333333333333333'
(or, equivalently, '31211101987654321'
)?$endgroup$
– Chas Brown
yesterday
$begingroup$
If
n==13
, can the topmost row be '33333333333333333'
(or, equivalently, '31211101987654321'
)?$endgroup$
– Chas Brown
yesterday
$begingroup$
@EmbodimentofIgnorance Sorry, but I'd say no in this case. The shapes and their corresponding
mod 4
are strict pairs for this challenge. So you may not switch the four shapes for the four mod 4
cases. But good question nonetheless.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@EmbodimentofIgnorance Sorry, but I'd say no in this case. The shapes and their corresponding
mod 4
are strict pairs for this challenge. So you may not switch the four shapes for the four mod 4
cases. But good question nonetheless.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@ChasBrown Yes, both of those are fine. I only gave three possible examples for the $n=13$, but all six options (like the $n=5$ test case) are valid outputs.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@ChasBrown Yes, both of those are fine. I only gave three possible examples for the $n=13$, but all six options (like the $n=5$ test case) are valid outputs.
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
19 Answers
19
active
oldest
votes
$begingroup$
Japt, 8 bytes
Returns an array of lines.
õ ¬å+ zU
Try it
Saved 2 bytes thanks to Kevin.
õ ¬å+ zU :Implicit input of integer U
õ :Range [1,U]
¬ :Join to a string
å+ :Cumulatively reduce by concatenation
zU :Rotate clockwise by 90 degrees U times
$endgroup$
1
$begingroup$
Is theú
necessary? It seems the rotate does this implicitly?
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to usez
.
$endgroup$
– Shaggy
yesterday
1
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
$begingroup$
JavaScript (ES6), 93 89 bytes
Returns a matrix of characters.
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((d,y,a)=>a.map(_=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Alternate pattern (same size):
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((_,y,a)=>a.map(d=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Commented
n => // n = input
[... // split the result of ...
( g = n => // ... a call to the recursive function g, taking n
n ? // if n is not equal to 0:
g(n - 1) // append the result of a recursive call with n - 1
+ n // append n
: // else:
'' // stop recursion and return an empty string
)(n) // initial call to g
].map((d, y, a) => // for each digit d at position y in this array a:
a.map(_ => // for each character in a:
y - // we test either y < 0 if (n AND 2) is not set
(n & 2) // or -y < 0 (i.e. y > 0) if (n AND 2) is set
* y-- < 0 // and we decrement y afterwards
? // if the above condition is met:
' ' // append a space
: // else:
d // append d
) // end of inner map()
) // end of outer map()
.sort(_ => -n % 2) // reverse the rows if n is odd
Shape summary
Below is a summary of the base shape (generated by the nested map
loops) and the final shape (after the sort
) for each $nbmod 4$:
n mod 4 | 0 | 1 | 2 | 3
----------+-------+-------+-------+-------
n & 2 | 0 | 0 | 2 | 2
----------+-------+-------+-------+-------
test | y < 0 | y < 0 | y > 0 | y > 0
----------+-------+-------+-------+-------
base | #.... | #.... | ##### | #####
shape | ##... | ##... | .#### | .####
| ###.. | ###.. | ..### | ..###
| ####. | ####. | ...## | ...##
| ##### | ##### | ....# | ....#
----------+-------+-------+-------+-------
n % 2 | 0 | 1 | 0 | 1
----------+-------+-------+-------+-------
reverse? | no | yes | no | yes
----------+-------+-------+-------+-------
final | #.... | ##### | ##### | ....#
shape | ##... | ####. | .#### | ...##
| ###.. | ###.. | ..### | ..###
| ####. | ##... | ...## | .####
| ##### | #.... | ....# | #####
$endgroup$
add a comment |
$begingroup$
Python 2, 94 bytes
n=0;s=''
exec"n+=1;s+=`n`;"*input()
K=k=len(s)
while k:k-=1;print s[k^n/-2%-2:].rjust(n%4/2*K)
Try it online!
$endgroup$
add a comment |
$begingroup$
Canvas, 8 bytes
ŗ]∑[]⁸[↷
Try it here!
$endgroup$
add a comment |
$begingroup$
Perl 6, 94 bytes
{[o](|(&reverse xx$_/2+.5),|(*>>.flip xx$_/2+1))([~](my@a=[~](1..$_).comb)>>.fmt("%{+@a}s"))}
Try it online!
Anonymous code block that takes a number and returns a list of lines.
$endgroup$
add a comment |
$begingroup$
Charcoal, 17 bytes
Nθ≔⭆θ⊕ιηGLLηη⟲⊗θ‖
Try it online! Link is to verbose version of code. Explanation:
Nθ
Input n
.
≔⭆θ⊕ιη
Create a string by concatenating the numbers 1
to n
.
GLLηη
Fill a triangle of that length with the string.
⟲⊗θ
Rotate the triangle anticlockwise by n*90
degrees.
‖
Reflect everything, thus ending up with a triangle that is rotated clockwise by n*90
degrees.
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 128 bytes
n=>{var s="";int i=0,j,p;for(;i<n;s+=++i);for(p=j=s.Length;;)Write("{0,"+(n%4>1?p:0)+"}n",new string(s[--j],-~n%4>1?j+1:p-j));}
Try it online!
$endgroup$
add a comment |
$begingroup$
Ruby, 95 82 79 bytes
->n{r=(0...z=/$/=~s=[*1..n]*'').map{|i|" "*n[1]*i+s[0,z-i]};-n&2>0?r:r.reverse}
Try it online!
3 bytes saved by G B.
$endgroup$
add a comment |
$begingroup$
APL+WIN, 45 bytes
Prompts for integer
m←⊃(⍳⍴s)↑¨⊂s←(⍕⍳n←⎕)~' '⋄⍎(' ⊖⍉⌽'[1+4|n]),'m'
Try it online! Courtesy of Dyalog Classic
$endgroup$
add a comment |
$begingroup$
PowerShell, 108 bytes
param($n)0..($y=($x=-join(1..$n)).length-1)|%{' '*(0,0,$_,($z=$y-$_))[$n%4]+-join$x[0..($_,$z,$z,$_)[$n%4]]}
Try it online!
A bit rough around the edges but works. Joins the digits 1 to n
into a string then iterates from 0 to the length of that string-1. Each time, it uses list indexing to swap to the correct spacing method and number range used to slice our new string.
$endgroup$
add a comment |
$begingroup$
Jelly, 12 bytes
D€Ẏ;z⁶U$⁸¡Y
Try it online!
$endgroup$
add a comment |
$begingroup$
05AB1E (legacy), 14 12 10 bytes
Using the legacy verion as the rewrite is extremely slow on this for some reason.
Saved 2 bytes thanks to Kevin Cruijssen
LSηsFRζ}J»
Try it online!
Explanation
L # push range [1 ... input]
S # split to list of individual digits
η # calculate prefixes
sF } # input times do:
R # reverse list
ζ # and transpose it
J # join to list of strings
» # and join on newlines
$endgroup$
$begingroup$
You can save 2 bytes changingLJη€S
toLSη
, sinceS
implicitly flattens.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried€S
which didn't work out very well ;)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
Stax, 10 bytes
Ç√çZ╟84Γó║
Run and debug it
$endgroup$
add a comment |
$begingroup$
PowerShell, 105 101 95 bytes
-4 bytes thanks Arnauld for the trick with Sort.
param($n)($x=1..$n-join'')|% t*y|%{($s+="$_")}|sort -d:($n%4-in1,2)|% *ft($x.Length*($n%4-ge2))
Try it online!
Less golfed:
param($n)
$x=1..$n-join''
$x|% toCharArray |% {
($s+="$_")
} | sort -Descending:($n%4-in1,2) |% PadLeft ($x.Length*($n%4-ge2))
$endgroup$
add a comment |
$begingroup$
Wolfram Language (Mathematica), 137 bytes
(t=Table[Table[" ",If[(m=#~Mod~4)>1,Tr[1^s]-k,0]]<>ToString/@s[[;;k]],{k,Length[s=Join@@IntegerDigits/@Range@#]}];If[0<m<3,Reverse@t,t])&
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 116 bytes
n=input()
s=''.join(map(str,range(1,n+1)));L=len(s)
p=-~n/2%2;i=~-L*p+1
exec'print s[:i].rjust(n/2%2*L);i+=1-2*p;'*L
Try it online!
$endgroup$
add a comment |
$begingroup$
Red, 155 bytes
func[n][b: copy""repeat i n[append b i]repeat i l:
length? b[t:[l - i + 1]set[k m]pick[i t[l t][l i]]n % 4 + 1
print pad/left copy/part b do do m do do k]]
Try it online!
$endgroup$
add a comment |
$begingroup$
perl 5, 117 bytes
$p=$_++&2?'/ ':'$/';$s='(.*d.n)';$r=$_--&2?$s.'K$':"^(?=$s)";$_=(join"",1..$_).$/;1while s,$r,'$1=~s/d'."$p/r",ee
TIO
$endgroup$
add a comment |
$begingroup$
R, 175 172 bytes
function(n)write(`[<-`(m<-matrix(S<-el(strsplit(x<-Reduce(paste0,1:n,""),"")),y<-nchar(x),y),upper.tri(m)["if"(n%%4%in%2:3,1:y,y:1),"if"(n%%4%in%1:2,1:y,y:1)]," "),1,y,,"")
Try it online!
A horrible in-line mess!
-3 bytes by changing the rotation condition
Ungolfed and simplified a bit:
function(n){
Concat <- Reduce(paste0,1:n,init="") # concatenate all the digits, with init = "" to handle the n=1 case
digits <- el(strsplit(Concat,"")) # split the string to get component digits
mat <- matrix(digits,nchar(Concat),nchar(Concat)) # create a matrix with the digits running down the columns,
# for example, n = 4 is [[1,1,1,1],[2,2,2,2],[3,3,3,3],[4,4,4,4]]
mat[upper.tri(mat)[(n%%4 condiditon),(n%%4 condition)]] <- " " # conditioned on (n %% 4), replace the values in mat with spaces
write(mat,1,nchar(Concat),,"") # print the transpose of mat
}
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181885%2fthe-digit-triangles%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
19 Answers
19
active
oldest
votes
19 Answers
19
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Japt, 8 bytes
Returns an array of lines.
õ ¬å+ zU
Try it
Saved 2 bytes thanks to Kevin.
õ ¬å+ zU :Implicit input of integer U
õ :Range [1,U]
¬ :Join to a string
å+ :Cumulatively reduce by concatenation
zU :Rotate clockwise by 90 degrees U times
$endgroup$
1
$begingroup$
Is theú
necessary? It seems the rotate does this implicitly?
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to usez
.
$endgroup$
– Shaggy
yesterday
1
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
$begingroup$
Japt, 8 bytes
Returns an array of lines.
õ ¬å+ zU
Try it
Saved 2 bytes thanks to Kevin.
õ ¬å+ zU :Implicit input of integer U
õ :Range [1,U]
¬ :Join to a string
å+ :Cumulatively reduce by concatenation
zU :Rotate clockwise by 90 degrees U times
$endgroup$
1
$begingroup$
Is theú
necessary? It seems the rotate does this implicitly?
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to usez
.
$endgroup$
– Shaggy
yesterday
1
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
$begingroup$
Japt, 8 bytes
Returns an array of lines.
õ ¬å+ zU
Try it
Saved 2 bytes thanks to Kevin.
õ ¬å+ zU :Implicit input of integer U
õ :Range [1,U]
¬ :Join to a string
å+ :Cumulatively reduce by concatenation
zU :Rotate clockwise by 90 degrees U times
$endgroup$
Japt, 8 bytes
Returns an array of lines.
õ ¬å+ zU
Try it
Saved 2 bytes thanks to Kevin.
õ ¬å+ zU :Implicit input of integer U
õ :Range [1,U]
¬ :Join to a string
å+ :Cumulatively reduce by concatenation
zU :Rotate clockwise by 90 degrees U times
edited yesterday
answered yesterday


ShaggyShaggy
19.1k21667
19.1k21667
1
$begingroup$
Is theú
necessary? It seems the rotate does this implicitly?
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to usez
.
$endgroup$
– Shaggy
yesterday
1
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
1
$begingroup$
Is theú
necessary? It seems the rotate does this implicitly?
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to usez
.
$endgroup$
– Shaggy
yesterday
1
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
1
1
$begingroup$
Is the
ú
necessary? It seems the rotate does this implicitly?$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Is the
ú
necessary? It seems the rotate does this implicitly?$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to use
z
.$endgroup$
– Shaggy
yesterday
$begingroup$
@KevinCruijssen, hmm ... so it does. I always forget that; rarely get to use
z
.$endgroup$
– Shaggy
yesterday
1
1
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Well, I don't know Japt at all. Was just curious what the output looked like without the padding for fun, and saw it worked exactly the same.. ;)
$endgroup$
– Kevin Cruijssen
yesterday
add a comment |
$begingroup$
JavaScript (ES6), 93 89 bytes
Returns a matrix of characters.
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((d,y,a)=>a.map(_=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Alternate pattern (same size):
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((_,y,a)=>a.map(d=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Commented
n => // n = input
[... // split the result of ...
( g = n => // ... a call to the recursive function g, taking n
n ? // if n is not equal to 0:
g(n - 1) // append the result of a recursive call with n - 1
+ n // append n
: // else:
'' // stop recursion and return an empty string
)(n) // initial call to g
].map((d, y, a) => // for each digit d at position y in this array a:
a.map(_ => // for each character in a:
y - // we test either y < 0 if (n AND 2) is not set
(n & 2) // or -y < 0 (i.e. y > 0) if (n AND 2) is set
* y-- < 0 // and we decrement y afterwards
? // if the above condition is met:
' ' // append a space
: // else:
d // append d
) // end of inner map()
) // end of outer map()
.sort(_ => -n % 2) // reverse the rows if n is odd
Shape summary
Below is a summary of the base shape (generated by the nested map
loops) and the final shape (after the sort
) for each $nbmod 4$:
n mod 4 | 0 | 1 | 2 | 3
----------+-------+-------+-------+-------
n & 2 | 0 | 0 | 2 | 2
----------+-------+-------+-------+-------
test | y < 0 | y < 0 | y > 0 | y > 0
----------+-------+-------+-------+-------
base | #.... | #.... | ##### | #####
shape | ##... | ##... | .#### | .####
| ###.. | ###.. | ..### | ..###
| ####. | ####. | ...## | ...##
| ##### | ##### | ....# | ....#
----------+-------+-------+-------+-------
n % 2 | 0 | 1 | 0 | 1
----------+-------+-------+-------+-------
reverse? | no | yes | no | yes
----------+-------+-------+-------+-------
final | #.... | ##### | ##### | ....#
shape | ##... | ####. | .#### | ...##
| ###.. | ###.. | ..### | ..###
| ####. | ##... | ...## | .####
| ##### | #.... | ....# | #####
$endgroup$
add a comment |
$begingroup$
JavaScript (ES6), 93 89 bytes
Returns a matrix of characters.
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((d,y,a)=>a.map(_=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Alternate pattern (same size):
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((_,y,a)=>a.map(d=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Commented
n => // n = input
[... // split the result of ...
( g = n => // ... a call to the recursive function g, taking n
n ? // if n is not equal to 0:
g(n - 1) // append the result of a recursive call with n - 1
+ n // append n
: // else:
'' // stop recursion and return an empty string
)(n) // initial call to g
].map((d, y, a) => // for each digit d at position y in this array a:
a.map(_ => // for each character in a:
y - // we test either y < 0 if (n AND 2) is not set
(n & 2) // or -y < 0 (i.e. y > 0) if (n AND 2) is set
* y-- < 0 // and we decrement y afterwards
? // if the above condition is met:
' ' // append a space
: // else:
d // append d
) // end of inner map()
) // end of outer map()
.sort(_ => -n % 2) // reverse the rows if n is odd
Shape summary
Below is a summary of the base shape (generated by the nested map
loops) and the final shape (after the sort
) for each $nbmod 4$:
n mod 4 | 0 | 1 | 2 | 3
----------+-------+-------+-------+-------
n & 2 | 0 | 0 | 2 | 2
----------+-------+-------+-------+-------
test | y < 0 | y < 0 | y > 0 | y > 0
----------+-------+-------+-------+-------
base | #.... | #.... | ##### | #####
shape | ##... | ##... | .#### | .####
| ###.. | ###.. | ..### | ..###
| ####. | ####. | ...## | ...##
| ##### | ##### | ....# | ....#
----------+-------+-------+-------+-------
n % 2 | 0 | 1 | 0 | 1
----------+-------+-------+-------+-------
reverse? | no | yes | no | yes
----------+-------+-------+-------+-------
final | #.... | ##### | ##### | ....#
shape | ##... | ####. | .#### | ...##
| ###.. | ###.. | ..### | ..###
| ####. | ##... | ...## | .####
| ##### | #.... | ....# | #####
$endgroup$
add a comment |
$begingroup$
JavaScript (ES6), 93 89 bytes
Returns a matrix of characters.
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((d,y,a)=>a.map(_=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Alternate pattern (same size):
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((_,y,a)=>a.map(d=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Commented
n => // n = input
[... // split the result of ...
( g = n => // ... a call to the recursive function g, taking n
n ? // if n is not equal to 0:
g(n - 1) // append the result of a recursive call with n - 1
+ n // append n
: // else:
'' // stop recursion and return an empty string
)(n) // initial call to g
].map((d, y, a) => // for each digit d at position y in this array a:
a.map(_ => // for each character in a:
y - // we test either y < 0 if (n AND 2) is not set
(n & 2) // or -y < 0 (i.e. y > 0) if (n AND 2) is set
* y-- < 0 // and we decrement y afterwards
? // if the above condition is met:
' ' // append a space
: // else:
d // append d
) // end of inner map()
) // end of outer map()
.sort(_ => -n % 2) // reverse the rows if n is odd
Shape summary
Below is a summary of the base shape (generated by the nested map
loops) and the final shape (after the sort
) for each $nbmod 4$:
n mod 4 | 0 | 1 | 2 | 3
----------+-------+-------+-------+-------
n & 2 | 0 | 0 | 2 | 2
----------+-------+-------+-------+-------
test | y < 0 | y < 0 | y > 0 | y > 0
----------+-------+-------+-------+-------
base | #.... | #.... | ##### | #####
shape | ##... | ##... | .#### | .####
| ###.. | ###.. | ..### | ..###
| ####. | ####. | ...## | ...##
| ##### | ##### | ....# | ....#
----------+-------+-------+-------+-------
n % 2 | 0 | 1 | 0 | 1
----------+-------+-------+-------+-------
reverse? | no | yes | no | yes
----------+-------+-------+-------+-------
final | #.... | ##### | ##### | ....#
shape | ##... | ####. | .#### | ...##
| ###.. | ###.. | ..### | ..###
| ####. | ##... | ...## | .####
| ##### | #.... | ....# | #####
$endgroup$
JavaScript (ES6), 93 89 bytes
Returns a matrix of characters.
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((d,y,a)=>a.map(_=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Alternate pattern (same size):
n=>[...(g=n=>n?g(n-1)+n:'')(n)].map((_,y,a)=>a.map(d=>y-(n&2)*y--<0?' ':d)).sort(_=>-n%2)
Try it online!
Commented
n => // n = input
[... // split the result of ...
( g = n => // ... a call to the recursive function g, taking n
n ? // if n is not equal to 0:
g(n - 1) // append the result of a recursive call with n - 1
+ n // append n
: // else:
'' // stop recursion and return an empty string
)(n) // initial call to g
].map((d, y, a) => // for each digit d at position y in this array a:
a.map(_ => // for each character in a:
y - // we test either y < 0 if (n AND 2) is not set
(n & 2) // or -y < 0 (i.e. y > 0) if (n AND 2) is set
* y-- < 0 // and we decrement y afterwards
? // if the above condition is met:
' ' // append a space
: // else:
d // append d
) // end of inner map()
) // end of outer map()
.sort(_ => -n % 2) // reverse the rows if n is odd
Shape summary
Below is a summary of the base shape (generated by the nested map
loops) and the final shape (after the sort
) for each $nbmod 4$:
n mod 4 | 0 | 1 | 2 | 3
----------+-------+-------+-------+-------
n & 2 | 0 | 0 | 2 | 2
----------+-------+-------+-------+-------
test | y < 0 | y < 0 | y > 0 | y > 0
----------+-------+-------+-------+-------
base | #.... | #.... | ##### | #####
shape | ##... | ##... | .#### | .####
| ###.. | ###.. | ..### | ..###
| ####. | ####. | ...## | ...##
| ##### | ##### | ....# | ....#
----------+-------+-------+-------+-------
n % 2 | 0 | 1 | 0 | 1
----------+-------+-------+-------+-------
reverse? | no | yes | no | yes
----------+-------+-------+-------+-------
final | #.... | ##### | ##### | ....#
shape | ##... | ####. | .#### | ...##
| ###.. | ###.. | ..### | ..###
| ####. | ##... | ...## | .####
| ##### | #.... | ....# | #####
edited yesterday
answered yesterday


ArnauldArnauld
79.5k796330
79.5k796330
add a comment |
add a comment |
$begingroup$
Python 2, 94 bytes
n=0;s=''
exec"n+=1;s+=`n`;"*input()
K=k=len(s)
while k:k-=1;print s[k^n/-2%-2:].rjust(n%4/2*K)
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 94 bytes
n=0;s=''
exec"n+=1;s+=`n`;"*input()
K=k=len(s)
while k:k-=1;print s[k^n/-2%-2:].rjust(n%4/2*K)
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 94 bytes
n=0;s=''
exec"n+=1;s+=`n`;"*input()
K=k=len(s)
while k:k-=1;print s[k^n/-2%-2:].rjust(n%4/2*K)
Try it online!
$endgroup$
Python 2, 94 bytes
n=0;s=''
exec"n+=1;s+=`n`;"*input()
K=k=len(s)
while k:k-=1;print s[k^n/-2%-2:].rjust(n%4/2*K)
Try it online!
answered 20 hours ago


xnorxnor
92.7k18188447
92.7k18188447
add a comment |
add a comment |
$begingroup$
Canvas, 8 bytes
ŗ]∑[]⁸[↷
Try it here!
$endgroup$
add a comment |
$begingroup$
Canvas, 8 bytes
ŗ]∑[]⁸[↷
Try it here!
$endgroup$
add a comment |
$begingroup$
Canvas, 8 bytes
ŗ]∑[]⁸[↷
Try it here!
$endgroup$
Canvas, 8 bytes
ŗ]∑[]⁸[↷
Try it here!
answered yesterday


dzaimadzaima
15.8k22059
15.8k22059
add a comment |
add a comment |
$begingroup$
Perl 6, 94 bytes
{[o](|(&reverse xx$_/2+.5),|(*>>.flip xx$_/2+1))([~](my@a=[~](1..$_).comb)>>.fmt("%{+@a}s"))}
Try it online!
Anonymous code block that takes a number and returns a list of lines.
$endgroup$
add a comment |
$begingroup$
Perl 6, 94 bytes
{[o](|(&reverse xx$_/2+.5),|(*>>.flip xx$_/2+1))([~](my@a=[~](1..$_).comb)>>.fmt("%{+@a}s"))}
Try it online!
Anonymous code block that takes a number and returns a list of lines.
$endgroup$
add a comment |
$begingroup$
Perl 6, 94 bytes
{[o](|(&reverse xx$_/2+.5),|(*>>.flip xx$_/2+1))([~](my@a=[~](1..$_).comb)>>.fmt("%{+@a}s"))}
Try it online!
Anonymous code block that takes a number and returns a list of lines.
$endgroup$
Perl 6, 94 bytes
{[o](|(&reverse xx$_/2+.5),|(*>>.flip xx$_/2+1))([~](my@a=[~](1..$_).comb)>>.fmt("%{+@a}s"))}
Try it online!
Anonymous code block that takes a number and returns a list of lines.
answered 23 hours ago
Jo KingJo King
25.2k359128
25.2k359128
add a comment |
add a comment |
$begingroup$
Charcoal, 17 bytes
Nθ≔⭆θ⊕ιηGLLηη⟲⊗θ‖
Try it online! Link is to verbose version of code. Explanation:
Nθ
Input n
.
≔⭆θ⊕ιη
Create a string by concatenating the numbers 1
to n
.
GLLηη
Fill a triangle of that length with the string.
⟲⊗θ
Rotate the triangle anticlockwise by n*90
degrees.
‖
Reflect everything, thus ending up with a triangle that is rotated clockwise by n*90
degrees.
$endgroup$
add a comment |
$begingroup$
Charcoal, 17 bytes
Nθ≔⭆θ⊕ιηGLLηη⟲⊗θ‖
Try it online! Link is to verbose version of code. Explanation:
Nθ
Input n
.
≔⭆θ⊕ιη
Create a string by concatenating the numbers 1
to n
.
GLLηη
Fill a triangle of that length with the string.
⟲⊗θ
Rotate the triangle anticlockwise by n*90
degrees.
‖
Reflect everything, thus ending up with a triangle that is rotated clockwise by n*90
degrees.
$endgroup$
add a comment |
$begingroup$
Charcoal, 17 bytes
Nθ≔⭆θ⊕ιηGLLηη⟲⊗θ‖
Try it online! Link is to verbose version of code. Explanation:
Nθ
Input n
.
≔⭆θ⊕ιη
Create a string by concatenating the numbers 1
to n
.
GLLηη
Fill a triangle of that length with the string.
⟲⊗θ
Rotate the triangle anticlockwise by n*90
degrees.
‖
Reflect everything, thus ending up with a triangle that is rotated clockwise by n*90
degrees.
$endgroup$
Charcoal, 17 bytes
Nθ≔⭆θ⊕ιηGLLηη⟲⊗θ‖
Try it online! Link is to verbose version of code. Explanation:
Nθ
Input n
.
≔⭆θ⊕ιη
Create a string by concatenating the numbers 1
to n
.
GLLηη
Fill a triangle of that length with the string.
⟲⊗θ
Rotate the triangle anticlockwise by n*90
degrees.
‖
Reflect everything, thus ending up with a triangle that is rotated clockwise by n*90
degrees.
answered yesterday
NeilNeil
82k745178
82k745178
add a comment |
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 128 bytes
n=>{var s="";int i=0,j,p;for(;i<n;s+=++i);for(p=j=s.Length;;)Write("{0,"+(n%4>1?p:0)+"}n",new string(s[--j],-~n%4>1?j+1:p-j));}
Try it online!
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 128 bytes
n=>{var s="";int i=0,j,p;for(;i<n;s+=++i);for(p=j=s.Length;;)Write("{0,"+(n%4>1?p:0)+"}n",new string(s[--j],-~n%4>1?j+1:p-j));}
Try it online!
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 128 bytes
n=>{var s="";int i=0,j,p;for(;i<n;s+=++i);for(p=j=s.Length;;)Write("{0,"+(n%4>1?p:0)+"}n",new string(s[--j],-~n%4>1?j+1:p-j));}
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 128 bytes
n=>{var s="";int i=0,j,p;for(;i<n;s+=++i);for(p=j=s.Length;;)Write("{0,"+(n%4>1?p:0)+"}n",new string(s[--j],-~n%4>1?j+1:p-j));}
Try it online!
answered 22 hours ago
Embodiment of IgnoranceEmbodiment of Ignorance
2,098125
2,098125
add a comment |
add a comment |
$begingroup$
Ruby, 95 82 79 bytes
->n{r=(0...z=/$/=~s=[*1..n]*'').map{|i|" "*n[1]*i+s[0,z-i]};-n&2>0?r:r.reverse}
Try it online!
3 bytes saved by G B.
$endgroup$
add a comment |
$begingroup$
Ruby, 95 82 79 bytes
->n{r=(0...z=/$/=~s=[*1..n]*'').map{|i|" "*n[1]*i+s[0,z-i]};-n&2>0?r:r.reverse}
Try it online!
3 bytes saved by G B.
$endgroup$
add a comment |
$begingroup$
Ruby, 95 82 79 bytes
->n{r=(0...z=/$/=~s=[*1..n]*'').map{|i|" "*n[1]*i+s[0,z-i]};-n&2>0?r:r.reverse}
Try it online!
3 bytes saved by G B.
$endgroup$
Ruby, 95 82 79 bytes
->n{r=(0...z=/$/=~s=[*1..n]*'').map{|i|" "*n[1]*i+s[0,z-i]};-n&2>0?r:r.reverse}
Try it online!
3 bytes saved by G B.
edited 11 hours ago
answered yesterday
Kirill L.Kirill L.
5,6431525
5,6431525
add a comment |
add a comment |
$begingroup$
APL+WIN, 45 bytes
Prompts for integer
m←⊃(⍳⍴s)↑¨⊂s←(⍕⍳n←⎕)~' '⋄⍎(' ⊖⍉⌽'[1+4|n]),'m'
Try it online! Courtesy of Dyalog Classic
$endgroup$
add a comment |
$begingroup$
APL+WIN, 45 bytes
Prompts for integer
m←⊃(⍳⍴s)↑¨⊂s←(⍕⍳n←⎕)~' '⋄⍎(' ⊖⍉⌽'[1+4|n]),'m'
Try it online! Courtesy of Dyalog Classic
$endgroup$
add a comment |
$begingroup$
APL+WIN, 45 bytes
Prompts for integer
m←⊃(⍳⍴s)↑¨⊂s←(⍕⍳n←⎕)~' '⋄⍎(' ⊖⍉⌽'[1+4|n]),'m'
Try it online! Courtesy of Dyalog Classic
$endgroup$
APL+WIN, 45 bytes
Prompts for integer
m←⊃(⍳⍴s)↑¨⊂s←(⍕⍳n←⎕)~' '⋄⍎(' ⊖⍉⌽'[1+4|n]),'m'
Try it online! Courtesy of Dyalog Classic
answered yesterday
GrahamGraham
2,59678
2,59678
add a comment |
add a comment |
$begingroup$
PowerShell, 108 bytes
param($n)0..($y=($x=-join(1..$n)).length-1)|%{' '*(0,0,$_,($z=$y-$_))[$n%4]+-join$x[0..($_,$z,$z,$_)[$n%4]]}
Try it online!
A bit rough around the edges but works. Joins the digits 1 to n
into a string then iterates from 0 to the length of that string-1. Each time, it uses list indexing to swap to the correct spacing method and number range used to slice our new string.
$endgroup$
add a comment |
$begingroup$
PowerShell, 108 bytes
param($n)0..($y=($x=-join(1..$n)).length-1)|%{' '*(0,0,$_,($z=$y-$_))[$n%4]+-join$x[0..($_,$z,$z,$_)[$n%4]]}
Try it online!
A bit rough around the edges but works. Joins the digits 1 to n
into a string then iterates from 0 to the length of that string-1. Each time, it uses list indexing to swap to the correct spacing method and number range used to slice our new string.
$endgroup$
add a comment |
$begingroup$
PowerShell, 108 bytes
param($n)0..($y=($x=-join(1..$n)).length-1)|%{' '*(0,0,$_,($z=$y-$_))[$n%4]+-join$x[0..($_,$z,$z,$_)[$n%4]]}
Try it online!
A bit rough around the edges but works. Joins the digits 1 to n
into a string then iterates from 0 to the length of that string-1. Each time, it uses list indexing to swap to the correct spacing method and number range used to slice our new string.
$endgroup$
PowerShell, 108 bytes
param($n)0..($y=($x=-join(1..$n)).length-1)|%{' '*(0,0,$_,($z=$y-$_))[$n%4]+-join$x[0..($_,$z,$z,$_)[$n%4]]}
Try it online!
A bit rough around the edges but works. Joins the digits 1 to n
into a string then iterates from 0 to the length of that string-1. Each time, it uses list indexing to swap to the correct spacing method and number range used to slice our new string.
edited yesterday
answered yesterday
VeskahVeskah
1,133214
1,133214
add a comment |
add a comment |
$begingroup$
Jelly, 12 bytes
D€Ẏ;z⁶U$⁸¡Y
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 12 bytes
D€Ẏ;z⁶U$⁸¡Y
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 12 bytes
D€Ẏ;z⁶U$⁸¡Y
Try it online!
$endgroup$
Jelly, 12 bytes
D€Ẏ;z⁶U$⁸¡Y
Try it online!
answered yesterday


Erik the OutgolferErik the Outgolfer
32.7k429105
32.7k429105
add a comment |
add a comment |
$begingroup$
05AB1E (legacy), 14 12 10 bytes
Using the legacy verion as the rewrite is extremely slow on this for some reason.
Saved 2 bytes thanks to Kevin Cruijssen
LSηsFRζ}J»
Try it online!
Explanation
L # push range [1 ... input]
S # split to list of individual digits
η # calculate prefixes
sF } # input times do:
R # reverse list
ζ # and transpose it
J # join to list of strings
» # and join on newlines
$endgroup$
$begingroup$
You can save 2 bytes changingLJη€S
toLSη
, sinceS
implicitly flattens.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried€S
which didn't work out very well ;)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
05AB1E (legacy), 14 12 10 bytes
Using the legacy verion as the rewrite is extremely slow on this for some reason.
Saved 2 bytes thanks to Kevin Cruijssen
LSηsFRζ}J»
Try it online!
Explanation
L # push range [1 ... input]
S # split to list of individual digits
η # calculate prefixes
sF } # input times do:
R # reverse list
ζ # and transpose it
J # join to list of strings
» # and join on newlines
$endgroup$
$begingroup$
You can save 2 bytes changingLJη€S
toLSη
, sinceS
implicitly flattens.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried€S
which didn't work out very well ;)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
05AB1E (legacy), 14 12 10 bytes
Using the legacy verion as the rewrite is extremely slow on this for some reason.
Saved 2 bytes thanks to Kevin Cruijssen
LSηsFRζ}J»
Try it online!
Explanation
L # push range [1 ... input]
S # split to list of individual digits
η # calculate prefixes
sF } # input times do:
R # reverse list
ζ # and transpose it
J # join to list of strings
» # and join on newlines
$endgroup$
05AB1E (legacy), 14 12 10 bytes
Using the legacy verion as the rewrite is extremely slow on this for some reason.
Saved 2 bytes thanks to Kevin Cruijssen
LSηsFRζ}J»
Try it online!
Explanation
L # push range [1 ... input]
S # split to list of individual digits
η # calculate prefixes
sF } # input times do:
R # reverse list
ζ # and transpose it
J # join to list of strings
» # and join on newlines
edited yesterday
answered yesterday


EmignaEmigna
47.1k433143
47.1k433143
$begingroup$
You can save 2 bytes changingLJη€S
toLSη
, sinceS
implicitly flattens.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried€S
which didn't work out very well ;)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
You can save 2 bytes changingLJη€S
toLSη
, sinceS
implicitly flattens.
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried€S
which didn't work out very well ;)
$endgroup$
– Emigna
yesterday
$begingroup$
You can save 2 bytes changing
LJη€S
to LSη
, since S
implicitly flattens.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
You can save 2 bytes changing
LJη€S
to LSη
, since S
implicitly flattens.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried
€S
which didn't work out very well ;)$endgroup$
– Emigna
yesterday
$begingroup$
@KevinCruijssen: Oh yeah, thanks! I'd forgotten about that. I tried
€S
which didn't work out very well ;)$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
Stax, 10 bytes
Ç√çZ╟84Γó║
Run and debug it
$endgroup$
add a comment |
$begingroup$
Stax, 10 bytes
Ç√çZ╟84Γó║
Run and debug it
$endgroup$
add a comment |
$begingroup$
Stax, 10 bytes
Ç√çZ╟84Γó║
Run and debug it
$endgroup$
Stax, 10 bytes
Ç√çZ╟84Γó║
Run and debug it
answered yesterday
recursiverecursive
5,6091322
5,6091322
add a comment |
add a comment |
$begingroup$
PowerShell, 105 101 95 bytes
-4 bytes thanks Arnauld for the trick with Sort.
param($n)($x=1..$n-join'')|% t*y|%{($s+="$_")}|sort -d:($n%4-in1,2)|% *ft($x.Length*($n%4-ge2))
Try it online!
Less golfed:
param($n)
$x=1..$n-join''
$x|% toCharArray |% {
($s+="$_")
} | sort -Descending:($n%4-in1,2) |% PadLeft ($x.Length*($n%4-ge2))
$endgroup$
add a comment |
$begingroup$
PowerShell, 105 101 95 bytes
-4 bytes thanks Arnauld for the trick with Sort.
param($n)($x=1..$n-join'')|% t*y|%{($s+="$_")}|sort -d:($n%4-in1,2)|% *ft($x.Length*($n%4-ge2))
Try it online!
Less golfed:
param($n)
$x=1..$n-join''
$x|% toCharArray |% {
($s+="$_")
} | sort -Descending:($n%4-in1,2) |% PadLeft ($x.Length*($n%4-ge2))
$endgroup$
add a comment |
$begingroup$
PowerShell, 105 101 95 bytes
-4 bytes thanks Arnauld for the trick with Sort.
param($n)($x=1..$n-join'')|% t*y|%{($s+="$_")}|sort -d:($n%4-in1,2)|% *ft($x.Length*($n%4-ge2))
Try it online!
Less golfed:
param($n)
$x=1..$n-join''
$x|% toCharArray |% {
($s+="$_")
} | sort -Descending:($n%4-in1,2) |% PadLeft ($x.Length*($n%4-ge2))
$endgroup$
PowerShell, 105 101 95 bytes
-4 bytes thanks Arnauld for the trick with Sort.
param($n)($x=1..$n-join'')|% t*y|%{($s+="$_")}|sort -d:($n%4-in1,2)|% *ft($x.Length*($n%4-ge2))
Try it online!
Less golfed:
param($n)
$x=1..$n-join''
$x|% toCharArray |% {
($s+="$_")
} | sort -Descending:($n%4-in1,2) |% PadLeft ($x.Length*($n%4-ge2))
edited 13 hours ago
answered 14 hours ago


mazzymazzy
2,8851317
2,8851317
add a comment |
add a comment |
$begingroup$
Wolfram Language (Mathematica), 137 bytes
(t=Table[Table[" ",If[(m=#~Mod~4)>1,Tr[1^s]-k,0]]<>ToString/@s[[;;k]],{k,Length[s=Join@@IntegerDigits/@Range@#]}];If[0<m<3,Reverse@t,t])&
Try it online!
$endgroup$
add a comment |
$begingroup$
Wolfram Language (Mathematica), 137 bytes
(t=Table[Table[" ",If[(m=#~Mod~4)>1,Tr[1^s]-k,0]]<>ToString/@s[[;;k]],{k,Length[s=Join@@IntegerDigits/@Range@#]}];If[0<m<3,Reverse@t,t])&
Try it online!
$endgroup$
add a comment |
$begingroup$
Wolfram Language (Mathematica), 137 bytes
(t=Table[Table[" ",If[(m=#~Mod~4)>1,Tr[1^s]-k,0]]<>ToString/@s[[;;k]],{k,Length[s=Join@@IntegerDigits/@Range@#]}];If[0<m<3,Reverse@t,t])&
Try it online!
$endgroup$
Wolfram Language (Mathematica), 137 bytes
(t=Table[Table[" ",If[(m=#~Mod~4)>1,Tr[1^s]-k,0]]<>ToString/@s[[;;k]],{k,Length[s=Join@@IntegerDigits/@Range@#]}];If[0<m<3,Reverse@t,t])&
Try it online!
edited yesterday
answered yesterday


J42161217J42161217
13.4k21251
13.4k21251
add a comment |
add a comment |
$begingroup$
Python 2, 116 bytes
n=input()
s=''.join(map(str,range(1,n+1)));L=len(s)
p=-~n/2%2;i=~-L*p+1
exec'print s[:i].rjust(n/2%2*L);i+=1-2*p;'*L
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 116 bytes
n=input()
s=''.join(map(str,range(1,n+1)));L=len(s)
p=-~n/2%2;i=~-L*p+1
exec'print s[:i].rjust(n/2%2*L);i+=1-2*p;'*L
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 116 bytes
n=input()
s=''.join(map(str,range(1,n+1)));L=len(s)
p=-~n/2%2;i=~-L*p+1
exec'print s[:i].rjust(n/2%2*L);i+=1-2*p;'*L
Try it online!
$endgroup$
Python 2, 116 bytes
n=input()
s=''.join(map(str,range(1,n+1)));L=len(s)
p=-~n/2%2;i=~-L*p+1
exec'print s[:i].rjust(n/2%2*L);i+=1-2*p;'*L
Try it online!
answered yesterday
Chas BrownChas Brown
5,0641523
5,0641523
add a comment |
add a comment |
$begingroup$
Red, 155 bytes
func[n][b: copy""repeat i n[append b i]repeat i l:
length? b[t:[l - i + 1]set[k m]pick[i t[l t][l i]]n % 4 + 1
print pad/left copy/part b do do m do do k]]
Try it online!
$endgroup$
add a comment |
$begingroup$
Red, 155 bytes
func[n][b: copy""repeat i n[append b i]repeat i l:
length? b[t:[l - i + 1]set[k m]pick[i t[l t][l i]]n % 4 + 1
print pad/left copy/part b do do m do do k]]
Try it online!
$endgroup$
add a comment |
$begingroup$
Red, 155 bytes
func[n][b: copy""repeat i n[append b i]repeat i l:
length? b[t:[l - i + 1]set[k m]pick[i t[l t][l i]]n % 4 + 1
print pad/left copy/part b do do m do do k]]
Try it online!
$endgroup$
Red, 155 bytes
func[n][b: copy""repeat i n[append b i]repeat i l:
length? b[t:[l - i + 1]set[k m]pick[i t[l t][l i]]n % 4 + 1
print pad/left copy/part b do do m do do k]]
Try it online!
edited 16 hours ago
answered 17 hours ago
Galen IvanovGalen Ivanov
7,18211034
7,18211034
add a comment |
add a comment |
$begingroup$
perl 5, 117 bytes
$p=$_++&2?'/ ':'$/';$s='(.*d.n)';$r=$_--&2?$s.'K$':"^(?=$s)";$_=(join"",1..$_).$/;1while s,$r,'$1=~s/d'."$p/r",ee
TIO
$endgroup$
add a comment |
$begingroup$
perl 5, 117 bytes
$p=$_++&2?'/ ':'$/';$s='(.*d.n)';$r=$_--&2?$s.'K$':"^(?=$s)";$_=(join"",1..$_).$/;1while s,$r,'$1=~s/d'."$p/r",ee
TIO
$endgroup$
add a comment |
$begingroup$
perl 5, 117 bytes
$p=$_++&2?'/ ':'$/';$s='(.*d.n)';$r=$_--&2?$s.'K$':"^(?=$s)";$_=(join"",1..$_).$/;1while s,$r,'$1=~s/d'."$p/r",ee
TIO
$endgroup$
perl 5, 117 bytes
$p=$_++&2?'/ ':'$/';$s='(.*d.n)';$r=$_--&2?$s.'K$':"^(?=$s)";$_=(join"",1..$_).$/;1while s,$r,'$1=~s/d'."$p/r",ee
TIO
edited 11 hours ago
answered 11 hours ago
Nahuel FouilleulNahuel Fouilleul
2,875211
2,875211
add a comment |
add a comment |
$begingroup$
R, 175 172 bytes
function(n)write(`[<-`(m<-matrix(S<-el(strsplit(x<-Reduce(paste0,1:n,""),"")),y<-nchar(x),y),upper.tri(m)["if"(n%%4%in%2:3,1:y,y:1),"if"(n%%4%in%1:2,1:y,y:1)]," "),1,y,,"")
Try it online!
A horrible in-line mess!
-3 bytes by changing the rotation condition
Ungolfed and simplified a bit:
function(n){
Concat <- Reduce(paste0,1:n,init="") # concatenate all the digits, with init = "" to handle the n=1 case
digits <- el(strsplit(Concat,"")) # split the string to get component digits
mat <- matrix(digits,nchar(Concat),nchar(Concat)) # create a matrix with the digits running down the columns,
# for example, n = 4 is [[1,1,1,1],[2,2,2,2],[3,3,3,3],[4,4,4,4]]
mat[upper.tri(mat)[(n%%4 condiditon),(n%%4 condition)]] <- " " # conditioned on (n %% 4), replace the values in mat with spaces
write(mat,1,nchar(Concat),,"") # print the transpose of mat
}
$endgroup$
add a comment |
$begingroup$
R, 175 172 bytes
function(n)write(`[<-`(m<-matrix(S<-el(strsplit(x<-Reduce(paste0,1:n,""),"")),y<-nchar(x),y),upper.tri(m)["if"(n%%4%in%2:3,1:y,y:1),"if"(n%%4%in%1:2,1:y,y:1)]," "),1,y,,"")
Try it online!
A horrible in-line mess!
-3 bytes by changing the rotation condition
Ungolfed and simplified a bit:
function(n){
Concat <- Reduce(paste0,1:n,init="") # concatenate all the digits, with init = "" to handle the n=1 case
digits <- el(strsplit(Concat,"")) # split the string to get component digits
mat <- matrix(digits,nchar(Concat),nchar(Concat)) # create a matrix with the digits running down the columns,
# for example, n = 4 is [[1,1,1,1],[2,2,2,2],[3,3,3,3],[4,4,4,4]]
mat[upper.tri(mat)[(n%%4 condiditon),(n%%4 condition)]] <- " " # conditioned on (n %% 4), replace the values in mat with spaces
write(mat,1,nchar(Concat),,"") # print the transpose of mat
}
$endgroup$
add a comment |
$begingroup$
R, 175 172 bytes
function(n)write(`[<-`(m<-matrix(S<-el(strsplit(x<-Reduce(paste0,1:n,""),"")),y<-nchar(x),y),upper.tri(m)["if"(n%%4%in%2:3,1:y,y:1),"if"(n%%4%in%1:2,1:y,y:1)]," "),1,y,,"")
Try it online!
A horrible in-line mess!
-3 bytes by changing the rotation condition
Ungolfed and simplified a bit:
function(n){
Concat <- Reduce(paste0,1:n,init="") # concatenate all the digits, with init = "" to handle the n=1 case
digits <- el(strsplit(Concat,"")) # split the string to get component digits
mat <- matrix(digits,nchar(Concat),nchar(Concat)) # create a matrix with the digits running down the columns,
# for example, n = 4 is [[1,1,1,1],[2,2,2,2],[3,3,3,3],[4,4,4,4]]
mat[upper.tri(mat)[(n%%4 condiditon),(n%%4 condition)]] <- " " # conditioned on (n %% 4), replace the values in mat with spaces
write(mat,1,nchar(Concat),,"") # print the transpose of mat
}
$endgroup$
R, 175 172 bytes
function(n)write(`[<-`(m<-matrix(S<-el(strsplit(x<-Reduce(paste0,1:n,""),"")),y<-nchar(x),y),upper.tri(m)["if"(n%%4%in%2:3,1:y,y:1),"if"(n%%4%in%1:2,1:y,y:1)]," "),1,y,,"")
Try it online!
A horrible in-line mess!
-3 bytes by changing the rotation condition
Ungolfed and simplified a bit:
function(n){
Concat <- Reduce(paste0,1:n,init="") # concatenate all the digits, with init = "" to handle the n=1 case
digits <- el(strsplit(Concat,"")) # split the string to get component digits
mat <- matrix(digits,nchar(Concat),nchar(Concat)) # create a matrix with the digits running down the columns,
# for example, n = 4 is [[1,1,1,1],[2,2,2,2],[3,3,3,3],[4,4,4,4]]
mat[upper.tri(mat)[(n%%4 condiditon),(n%%4 condition)]] <- " " # conditioned on (n %% 4), replace the values in mat with spaces
write(mat,1,nchar(Concat),,"") # print the transpose of mat
}
edited 7 hours ago
answered 7 hours ago
GiuseppeGiuseppe
17k31052
17k31052
add a comment |
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181885%2fthe-digit-triangles%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bQEDHRml,bYa1 TljxVyo A,I2M7wCUDu3shpVkO4,L10 HJYSVG6f1fACz I4d 3 R ljShYgufh tXQ6RQyXs2H BjAD
$begingroup$
Can we use other values for different triangles, like 1 for ◤, etc?
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
@EmbodimentofIgnorance Unfortunate example, since that's what the spec says. I think you wanted to ask if we can change the order of the four arrangements as long as we keep it consistent (I think that would be a no).
$endgroup$
– Erik the Outgolfer
yesterday
1
$begingroup$
If
n==13
, can the topmost row be'33333333333333333'
(or, equivalently,'31211101987654321'
)?$endgroup$
– Chas Brown
yesterday
$begingroup$
@EmbodimentofIgnorance Sorry, but I'd say no in this case. The shapes and their corresponding
mod 4
are strict pairs for this challenge. So you may not switch the four shapes for the fourmod 4
cases. But good question nonetheless.$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@ChasBrown Yes, both of those are fine. I only gave three possible examples for the $n=13$, but all six options (like the $n=5$ test case) are valid outputs.
$endgroup$
– Kevin Cruijssen
yesterday