How to describe a state with three items
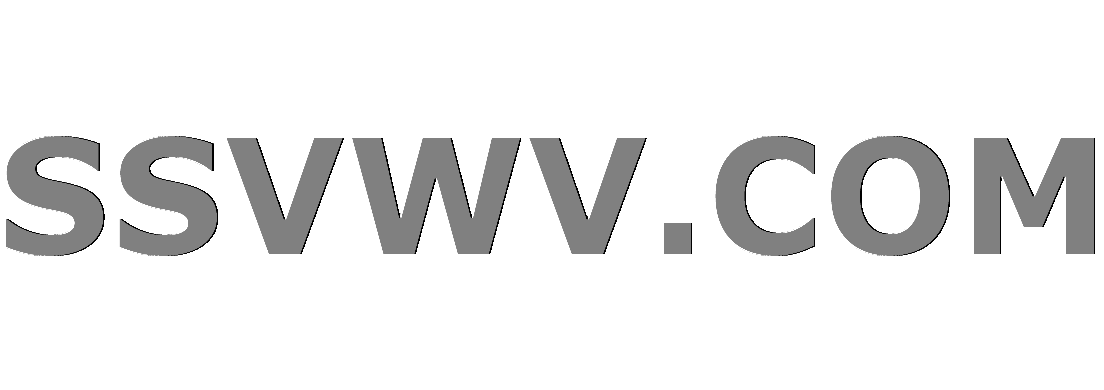
Multi tool use
up vote
6
down vote
favorite
I am creating the board game Tic Tac Toe in Java.
A cell will have three states: empty, X or O.
What is the best practice for representing this in Java? Should I create its own Cell class or just use integers (0/1/2) to represent the three states? If it had two states then I could use for example boolean to represent the two states, is there a similar already defined class for something with three states?
java
add a comment |
up vote
6
down vote
favorite
I am creating the board game Tic Tac Toe in Java.
A cell will have three states: empty, X or O.
What is the best practice for representing this in Java? Should I create its own Cell class or just use integers (0/1/2) to represent the three states? If it had two states then I could use for example boolean to represent the two states, is there a similar already defined class for something with three states?
java
Note that what you're calling a cell is really just the possible content values of a cell. A cell is a location on the board, for TTT you have 9 cells. Naming matters, especially for code readability purposes.
– Flater
Nov 27 at 13:14
1
Bool
of course ;-)
– Emil Vikström
Nov 27 at 13:23
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
I am creating the board game Tic Tac Toe in Java.
A cell will have three states: empty, X or O.
What is the best practice for representing this in Java? Should I create its own Cell class or just use integers (0/1/2) to represent the three states? If it had two states then I could use for example boolean to represent the two states, is there a similar already defined class for something with three states?
java
I am creating the board game Tic Tac Toe in Java.
A cell will have three states: empty, X or O.
What is the best practice for representing this in Java? Should I create its own Cell class or just use integers (0/1/2) to represent the three states? If it had two states then I could use for example boolean to represent the two states, is there a similar already defined class for something with three states?
java
java
edited Nov 27 at 12:56
yoozer8
5,70543872
5,70543872
asked Nov 27 at 11:31
Alex
406722
406722
Note that what you're calling a cell is really just the possible content values of a cell. A cell is a location on the board, for TTT you have 9 cells. Naming matters, especially for code readability purposes.
– Flater
Nov 27 at 13:14
1
Bool
of course ;-)
– Emil Vikström
Nov 27 at 13:23
add a comment |
Note that what you're calling a cell is really just the possible content values of a cell. A cell is a location on the board, for TTT you have 9 cells. Naming matters, especially for code readability purposes.
– Flater
Nov 27 at 13:14
1
Bool
of course ;-)
– Emil Vikström
Nov 27 at 13:23
Note that what you're calling a cell is really just the possible content values of a cell. A cell is a location on the board, for TTT you have 9 cells. Naming matters, especially for code readability purposes.
– Flater
Nov 27 at 13:14
Note that what you're calling a cell is really just the possible content values of a cell. A cell is a location on the board, for TTT you have 9 cells. Naming matters, especially for code readability purposes.
– Flater
Nov 27 at 13:14
1
1
Bool
of course ;-)– Emil Vikström
Nov 27 at 13:23
Bool
of course ;-)– Emil Vikström
Nov 27 at 13:23
add a comment |
6 Answers
6
active
oldest
votes
up vote
10
down vote
I would use an enum for this:
enum CellState {
EMPTY,
X,
O
}
And then in your code:
public static void main(String args) {
CellState cellStates = new CellState[3][3];
cellStates[0][0] = CellState.X;
// Do other stuff
}
I just defined the board structure as CellState
as example but this can be whatever.
add a comment |
up vote
3
down vote
About the most important thing when using an OO language is that objects model behaviour and, where possible, contain the data required to implement the behaviour. Behaviour is what the objects do, not what is done to them.
So unless there is a reason to in a requirement you haven't stated, the cell itself doesn't have any behaviour, it is just a place that the players mark.
So you could have a simple array of marks that both players update, with an enum with three values, or you could have each player update their own data of the marks they have made, in which case each player would have either a boolean array or a short bit mask to indicate their goes. In the latter case, each player then only changes the state of their own 'goes' and can implement the test for their winning condition rather than having shared state - the only sharing is they have to ask the other player whether the chosen cell is valid. It depends how strictly OO you want your design to be as to whether this is 'best practice' or not - for such a simple problem you could write in COBOL and the users would be as happy.
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
add a comment |
up vote
2
down vote
I would use an enum :
public enum CellState {
EMPTY,
X,
O;
}
And a Cell class that has a field of type CellState
add a comment |
up vote
2
down vote
You could use an enum which contains the three values, like:
public enum CellState {
EMPTY,
X,
O
}
And use it like in a way like this:
board.setCell(cellposition, CellState.X);
add a comment |
up vote
2
down vote
There are multiple approaches but in this case I prefer using an enum to represent your state.
public enum State {
EMPTY,
X,
O
}
And then your cell class would look something like this.
public class Cell {
private State state;
public Cell(State state) {
this.state = state;
}
public State getState {
return state;
}
public void setState(State state) {
this.state = state;
}
}
add a comment |
up vote
-8
down vote
The other way is just to use Boolean
object and to use null
as third state.
Boolean state = null; // => empty state
state = Boolean.TRUE // => X state
state = Boolean.FALSE // => O state
2
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
AlsoBoolean state;
is wrong, its not "empty" but rather uninitialized
– Lino
Nov 27 at 17:20
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
add a comment |
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
10
down vote
I would use an enum for this:
enum CellState {
EMPTY,
X,
O
}
And then in your code:
public static void main(String args) {
CellState cellStates = new CellState[3][3];
cellStates[0][0] = CellState.X;
// Do other stuff
}
I just defined the board structure as CellState
as example but this can be whatever.
add a comment |
up vote
10
down vote
I would use an enum for this:
enum CellState {
EMPTY,
X,
O
}
And then in your code:
public static void main(String args) {
CellState cellStates = new CellState[3][3];
cellStates[0][0] = CellState.X;
// Do other stuff
}
I just defined the board structure as CellState
as example but this can be whatever.
add a comment |
up vote
10
down vote
up vote
10
down vote
I would use an enum for this:
enum CellState {
EMPTY,
X,
O
}
And then in your code:
public static void main(String args) {
CellState cellStates = new CellState[3][3];
cellStates[0][0] = CellState.X;
// Do other stuff
}
I just defined the board structure as CellState
as example but this can be whatever.
I would use an enum for this:
enum CellState {
EMPTY,
X,
O
}
And then in your code:
public static void main(String args) {
CellState cellStates = new CellState[3][3];
cellStates[0][0] = CellState.X;
// Do other stuff
}
I just defined the board structure as CellState
as example but this can be whatever.
answered Nov 27 at 11:35
Mark
2,7191722
2,7191722
add a comment |
add a comment |
up vote
3
down vote
About the most important thing when using an OO language is that objects model behaviour and, where possible, contain the data required to implement the behaviour. Behaviour is what the objects do, not what is done to them.
So unless there is a reason to in a requirement you haven't stated, the cell itself doesn't have any behaviour, it is just a place that the players mark.
So you could have a simple array of marks that both players update, with an enum with three values, or you could have each player update their own data of the marks they have made, in which case each player would have either a boolean array or a short bit mask to indicate their goes. In the latter case, each player then only changes the state of their own 'goes' and can implement the test for their winning condition rather than having shared state - the only sharing is they have to ask the other player whether the chosen cell is valid. It depends how strictly OO you want your design to be as to whether this is 'best practice' or not - for such a simple problem you could write in COBOL and the users would be as happy.
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
add a comment |
up vote
3
down vote
About the most important thing when using an OO language is that objects model behaviour and, where possible, contain the data required to implement the behaviour. Behaviour is what the objects do, not what is done to them.
So unless there is a reason to in a requirement you haven't stated, the cell itself doesn't have any behaviour, it is just a place that the players mark.
So you could have a simple array of marks that both players update, with an enum with three values, or you could have each player update their own data of the marks they have made, in which case each player would have either a boolean array or a short bit mask to indicate their goes. In the latter case, each player then only changes the state of their own 'goes' and can implement the test for their winning condition rather than having shared state - the only sharing is they have to ask the other player whether the chosen cell is valid. It depends how strictly OO you want your design to be as to whether this is 'best practice' or not - for such a simple problem you could write in COBOL and the users would be as happy.
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
add a comment |
up vote
3
down vote
up vote
3
down vote
About the most important thing when using an OO language is that objects model behaviour and, where possible, contain the data required to implement the behaviour. Behaviour is what the objects do, not what is done to them.
So unless there is a reason to in a requirement you haven't stated, the cell itself doesn't have any behaviour, it is just a place that the players mark.
So you could have a simple array of marks that both players update, with an enum with three values, or you could have each player update their own data of the marks they have made, in which case each player would have either a boolean array or a short bit mask to indicate their goes. In the latter case, each player then only changes the state of their own 'goes' and can implement the test for their winning condition rather than having shared state - the only sharing is they have to ask the other player whether the chosen cell is valid. It depends how strictly OO you want your design to be as to whether this is 'best practice' or not - for such a simple problem you could write in COBOL and the users would be as happy.
About the most important thing when using an OO language is that objects model behaviour and, where possible, contain the data required to implement the behaviour. Behaviour is what the objects do, not what is done to them.
So unless there is a reason to in a requirement you haven't stated, the cell itself doesn't have any behaviour, it is just a place that the players mark.
So you could have a simple array of marks that both players update, with an enum with three values, or you could have each player update their own data of the marks they have made, in which case each player would have either a boolean array or a short bit mask to indicate their goes. In the latter case, each player then only changes the state of their own 'goes' and can implement the test for their winning condition rather than having shared state - the only sharing is they have to ask the other player whether the chosen cell is valid. It depends how strictly OO you want your design to be as to whether this is 'best practice' or not - for such a simple problem you could write in COBOL and the users would be as happy.
edited Nov 27 at 17:19


Lino
6,96321936
6,96321936
answered Nov 27 at 13:32
Pete Kirkham
43k378151
43k378151
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
add a comment |
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
As you said, in a properly-designed class, how the class stores the state of each cell should not matter, and should not be visible to, anything outside the class.
– Jeff Dege
Nov 27 at 13:39
add a comment |
up vote
2
down vote
I would use an enum :
public enum CellState {
EMPTY,
X,
O;
}
And a Cell class that has a field of type CellState
add a comment |
up vote
2
down vote
I would use an enum :
public enum CellState {
EMPTY,
X,
O;
}
And a Cell class that has a field of type CellState
add a comment |
up vote
2
down vote
up vote
2
down vote
I would use an enum :
public enum CellState {
EMPTY,
X,
O;
}
And a Cell class that has a field of type CellState
I would use an enum :
public enum CellState {
EMPTY,
X,
O;
}
And a Cell class that has a field of type CellState
answered Nov 27 at 11:34


TheWildHealer
403215
403215
add a comment |
add a comment |
up vote
2
down vote
You could use an enum which contains the three values, like:
public enum CellState {
EMPTY,
X,
O
}
And use it like in a way like this:
board.setCell(cellposition, CellState.X);
add a comment |
up vote
2
down vote
You could use an enum which contains the three values, like:
public enum CellState {
EMPTY,
X,
O
}
And use it like in a way like this:
board.setCell(cellposition, CellState.X);
add a comment |
up vote
2
down vote
up vote
2
down vote
You could use an enum which contains the three values, like:
public enum CellState {
EMPTY,
X,
O
}
And use it like in a way like this:
board.setCell(cellposition, CellState.X);
You could use an enum which contains the three values, like:
public enum CellState {
EMPTY,
X,
O
}
And use it like in a way like this:
board.setCell(cellposition, CellState.X);
answered Nov 27 at 11:35
Sven Hakvoort
1,583518
1,583518
add a comment |
add a comment |
up vote
2
down vote
There are multiple approaches but in this case I prefer using an enum to represent your state.
public enum State {
EMPTY,
X,
O
}
And then your cell class would look something like this.
public class Cell {
private State state;
public Cell(State state) {
this.state = state;
}
public State getState {
return state;
}
public void setState(State state) {
this.state = state;
}
}
add a comment |
up vote
2
down vote
There are multiple approaches but in this case I prefer using an enum to represent your state.
public enum State {
EMPTY,
X,
O
}
And then your cell class would look something like this.
public class Cell {
private State state;
public Cell(State state) {
this.state = state;
}
public State getState {
return state;
}
public void setState(State state) {
this.state = state;
}
}
add a comment |
up vote
2
down vote
up vote
2
down vote
There are multiple approaches but in this case I prefer using an enum to represent your state.
public enum State {
EMPTY,
X,
O
}
And then your cell class would look something like this.
public class Cell {
private State state;
public Cell(State state) {
this.state = state;
}
public State getState {
return state;
}
public void setState(State state) {
this.state = state;
}
}
There are multiple approaches but in this case I prefer using an enum to represent your state.
public enum State {
EMPTY,
X,
O
}
And then your cell class would look something like this.
public class Cell {
private State state;
public Cell(State state) {
this.state = state;
}
public State getState {
return state;
}
public void setState(State state) {
this.state = state;
}
}
answered Nov 27 at 11:38
David Baak
7291818
7291818
add a comment |
add a comment |
up vote
-8
down vote
The other way is just to use Boolean
object and to use null
as third state.
Boolean state = null; // => empty state
state = Boolean.TRUE // => X state
state = Boolean.FALSE // => O state
2
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
AlsoBoolean state;
is wrong, its not "empty" but rather uninitialized
– Lino
Nov 27 at 17:20
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
add a comment |
up vote
-8
down vote
The other way is just to use Boolean
object and to use null
as third state.
Boolean state = null; // => empty state
state = Boolean.TRUE // => X state
state = Boolean.FALSE // => O state
2
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
AlsoBoolean state;
is wrong, its not "empty" but rather uninitialized
– Lino
Nov 27 at 17:20
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
add a comment |
up vote
-8
down vote
up vote
-8
down vote
The other way is just to use Boolean
object and to use null
as third state.
Boolean state = null; // => empty state
state = Boolean.TRUE // => X state
state = Boolean.FALSE // => O state
The other way is just to use Boolean
object and to use null
as third state.
Boolean state = null; // => empty state
state = Boolean.TRUE // => X state
state = Boolean.FALSE // => O state
edited Nov 28 at 16:43
answered Nov 27 at 11:48


Tony
3718
3718
2
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
AlsoBoolean state;
is wrong, its not "empty" but rather uninitialized
– Lino
Nov 27 at 17:20
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
add a comment |
2
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
AlsoBoolean state;
is wrong, its not "empty" but rather uninitialized
– Lino
Nov 27 at 17:20
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
2
2
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
Using nulls for anything other than faliure cases is a bad idea.
– Tejas Kale
Nov 27 at 13:30
Also
Boolean state;
is wrong, its not "empty" but rather uninitialized– Lino
Nov 27 at 17:20
Also
Boolean state;
is wrong, its not "empty" but rather uninitialized– Lino
Nov 27 at 17:20
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
It depends on the context like everything in the programming world. If he wants something quick, without having to add additional classes, enums, etc., which will bring additional logic to handle them and tests, he can just use this approach for his game.
– Tony
Nov 28 at 16:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53498708%2fhow-to-describe-a-state-with-three-items%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4H,N lM8m6Buq
Note that what you're calling a cell is really just the possible content values of a cell. A cell is a location on the board, for TTT you have 9 cells. Naming matters, especially for code readability purposes.
– Flater
Nov 27 at 13:14
1
Bool
of course ;-)– Emil Vikström
Nov 27 at 13:23