Recursively print pyramid of numbers
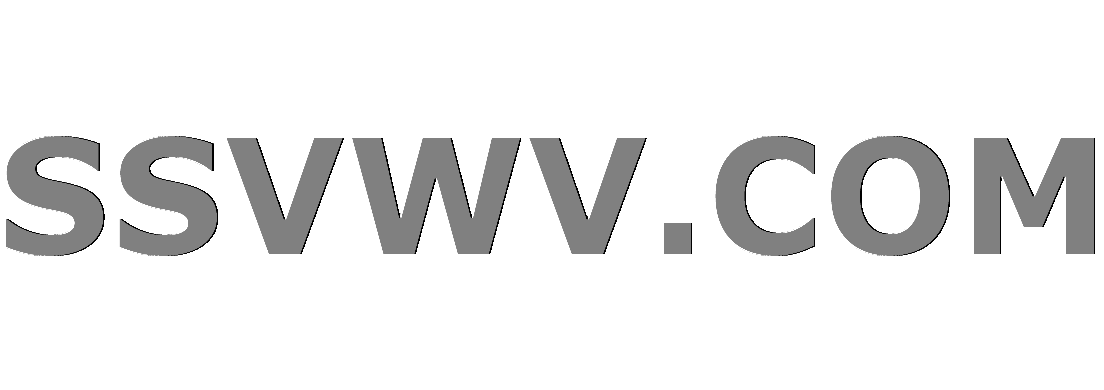
Multi tool use
up vote
5
down vote
favorite
I have to print a pyramid of numbers with this rule:
odd index: 1 to index,
even index: index to 1:
1
21
123
4321
12345
654321
1234567
87654321
123456789
I wrote this code:
def printFigure(rows):
if rows > 0:
if rows%2 == 0:
printFigure(rows-1)
while(rows>0):
print(str(rows)[::-1], end = '')
rows -= 1
print('')
if rows%2 == 1:
printFigure(rows-1)
while (rows>0):
print(str(rows),end = '')
rows -= 1
print('')
but the output is:
1
21
321,
4321
54321
654321
7654321
87654321
987654321
I'm a beginner with recursion, I'll be glad for your explanations too.
thanks.
python recursion
|
show 1 more comment
up vote
5
down vote
favorite
I have to print a pyramid of numbers with this rule:
odd index: 1 to index,
even index: index to 1:
1
21
123
4321
12345
654321
1234567
87654321
123456789
I wrote this code:
def printFigure(rows):
if rows > 0:
if rows%2 == 0:
printFigure(rows-1)
while(rows>0):
print(str(rows)[::-1], end = '')
rows -= 1
print('')
if rows%2 == 1:
printFigure(rows-1)
while (rows>0):
print(str(rows),end = '')
rows -= 1
print('')
but the output is:
1
21
321,
4321
54321
654321
7654321
87654321
987654321
I'm a beginner with recursion, I'll be glad for your explanations too.
thanks.
python recursion
2
Do you need to use recursion?
– Daniel Mesejo
Nov 29 at 10:32
1
print('n'.join([''.join(map(str, range(1, n+1)[::(-1)**(n%2)])) for n in range(1, 10)]))
– Ev. Kounis
Nov 29 at 10:40
1
@Ev.Kounis(1,-1)[n%2]
:D
– Mateen Ulhaq
Nov 29 at 10:54
@MateenUlhaq It is the same number of bytes though, right? But nice!
– Ev. Kounis
Nov 29 at 10:54
1
@MateenUlhaqn%2*2-1
and in python2 you can skip parenthesis. And range(9):print'n'.join('123456789'[:n+1][::1-n%2*2]for n in range(9))
– zch
Nov 29 at 12:57
|
show 1 more comment
up vote
5
down vote
favorite
up vote
5
down vote
favorite
I have to print a pyramid of numbers with this rule:
odd index: 1 to index,
even index: index to 1:
1
21
123
4321
12345
654321
1234567
87654321
123456789
I wrote this code:
def printFigure(rows):
if rows > 0:
if rows%2 == 0:
printFigure(rows-1)
while(rows>0):
print(str(rows)[::-1], end = '')
rows -= 1
print('')
if rows%2 == 1:
printFigure(rows-1)
while (rows>0):
print(str(rows),end = '')
rows -= 1
print('')
but the output is:
1
21
321,
4321
54321
654321
7654321
87654321
987654321
I'm a beginner with recursion, I'll be glad for your explanations too.
thanks.
python recursion
I have to print a pyramid of numbers with this rule:
odd index: 1 to index,
even index: index to 1:
1
21
123
4321
12345
654321
1234567
87654321
123456789
I wrote this code:
def printFigure(rows):
if rows > 0:
if rows%2 == 0:
printFigure(rows-1)
while(rows>0):
print(str(rows)[::-1], end = '')
rows -= 1
print('')
if rows%2 == 1:
printFigure(rows-1)
while (rows>0):
print(str(rows),end = '')
rows -= 1
print('')
but the output is:
1
21
321,
4321
54321
654321
7654321
87654321
987654321
I'm a beginner with recursion, I'll be glad for your explanations too.
thanks.
python recursion
python recursion
edited Nov 29 at 11:55


Glorfindel
16.4k114869
16.4k114869
asked Nov 29 at 10:23
Benjamin Yakobi
525
525
2
Do you need to use recursion?
– Daniel Mesejo
Nov 29 at 10:32
1
print('n'.join([''.join(map(str, range(1, n+1)[::(-1)**(n%2)])) for n in range(1, 10)]))
– Ev. Kounis
Nov 29 at 10:40
1
@Ev.Kounis(1,-1)[n%2]
:D
– Mateen Ulhaq
Nov 29 at 10:54
@MateenUlhaq It is the same number of bytes though, right? But nice!
– Ev. Kounis
Nov 29 at 10:54
1
@MateenUlhaqn%2*2-1
and in python2 you can skip parenthesis. And range(9):print'n'.join('123456789'[:n+1][::1-n%2*2]for n in range(9))
– zch
Nov 29 at 12:57
|
show 1 more comment
2
Do you need to use recursion?
– Daniel Mesejo
Nov 29 at 10:32
1
print('n'.join([''.join(map(str, range(1, n+1)[::(-1)**(n%2)])) for n in range(1, 10)]))
– Ev. Kounis
Nov 29 at 10:40
1
@Ev.Kounis(1,-1)[n%2]
:D
– Mateen Ulhaq
Nov 29 at 10:54
@MateenUlhaq It is the same number of bytes though, right? But nice!
– Ev. Kounis
Nov 29 at 10:54
1
@MateenUlhaqn%2*2-1
and in python2 you can skip parenthesis. And range(9):print'n'.join('123456789'[:n+1][::1-n%2*2]for n in range(9))
– zch
Nov 29 at 12:57
2
2
Do you need to use recursion?
– Daniel Mesejo
Nov 29 at 10:32
Do you need to use recursion?
– Daniel Mesejo
Nov 29 at 10:32
1
1
print('n'.join([''.join(map(str, range(1, n+1)[::(-1)**(n%2)])) for n in range(1, 10)]))
– Ev. Kounis
Nov 29 at 10:40
print('n'.join([''.join(map(str, range(1, n+1)[::(-1)**(n%2)])) for n in range(1, 10)]))
– Ev. Kounis
Nov 29 at 10:40
1
1
@Ev.Kounis
(1,-1)[n%2]
:D– Mateen Ulhaq
Nov 29 at 10:54
@Ev.Kounis
(1,-1)[n%2]
:D– Mateen Ulhaq
Nov 29 at 10:54
@MateenUlhaq It is the same number of bytes though, right? But nice!
– Ev. Kounis
Nov 29 at 10:54
@MateenUlhaq It is the same number of bytes though, right? But nice!
– Ev. Kounis
Nov 29 at 10:54
1
1
@MateenUlhaq
n%2*2-1
and in python2 you can skip parenthesis. And range(9): print'n'.join('123456789'[:n+1][::1-n%2*2]for n in range(9))
– zch
Nov 29 at 12:57
@MateenUlhaq
n%2*2-1
and in python2 you can skip parenthesis. And range(9): print'n'.join('123456789'[:n+1][::1-n%2*2]for n in range(9))
– zch
Nov 29 at 12:57
|
show 1 more comment
5 Answers
5
active
oldest
votes
up vote
4
down vote
accepted
You have two major problems with your current code. First, the check on whether the rows
is even or odd should be happening after the recursive call to printFigure
. The reason for this is that it is after backing out from the recursive call that you want to decide to print the line in order or backwards.
The second problem was with the logic in the else
printing condition. You need to print starting at 1, up to the number of rows. I used a dummy variable to achieve this, though there are probably a few other ways of doing it.
def printFigure(rows):
if rows > 0:
printFigure(rows-1)
if rows%2 == 0:
while(rows>0):
print(str(rows)[::-1], end='')
rows -= 1
print('')
else:
i = 1
while (i <= rows):
print(str(i), end='')
i += 1
print('')
printFigure(9)
1
21
123
4321
12345
654321
1234567
87654321
123456789
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
1
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
add a comment |
up vote
3
down vote
if you don't need to use recursivion, other simple solution is:
def printFigure(rows):
for x in range(rows):
items = [str(i) for i in range(1, x + 1)]
if x % 2 == 0:
items = items[::-1]
print(''.join(items))
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
glad you liked it )
– Bear Brown
Nov 29 at 11:10
add a comment |
up vote
2
down vote
You could use an inner function that prints from bottom to top, and the call this function from an outer function, for instance:
def print_rows(n, limit):
if n < limit:
numbers = range(1, n + 1) if n % 2 == 1 else reversed(range(1, n + 1))
print(''.join(map(str, numbers)))
print_rows(n + 1, limit)
def print_pyramid_recursive(n):
if n > 0:
print_rows(1, n)
print_pyramid_recursive(10)
Output
1
21
123
4321
12345
654321
1234567
87654321
123456789
In this case the inner function is print_rows
and the outer function is print_pyramid_recursive
. Note, that this problem has a very simple non-recursive solution, for example:
def print_pyramid(n):
for i in range(1, n + 1):
numbers = range(1, i + 1) if i % 2 == 1 else reversed(range(1, i + 1))
print(''.join(map(str, numbers)))
add a comment |
up vote
2
down vote
Here's the simplest loop version:
is_reversed = False
for i in range(1, 10):
step = -1 if is_reversed else 1
print(''.join(map(str, range(1, i + 1)))[::step])
is_reversed = not is_reversed
This regenerates a string every iteration. We can also store the value of the previous result and build on that:
s = ''
is_reversed = False
for i in range(1, 10):
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
And of course, this can easily (and pointlessly) be converted to a tail-recursive function by just passing along the stack:
def f(s, i, max_i, is_reversed):
if i == max_i:
return
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
i += 1
f(s, i, max_i, is_reversed)
f('', 1, 10, False)
The results for each of these increasingly weird looking pieces of code:
1
21
123
4321
12345
654321
1234567
87654321
123456789
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
add a comment |
up vote
1
down vote
You can use simple recursion:
def print_pyramid(_count = 1):
if _count < 10:
print((lambda x:x[::-1] if not _count%2 else x)(''.join(map(str, range(1, _count+1)))))
print_pyramid(_count+1)
print_pyramid()
Output:
1
21
123
4321
12345
654321
1234567
87654321
123456789
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
You have two major problems with your current code. First, the check on whether the rows
is even or odd should be happening after the recursive call to printFigure
. The reason for this is that it is after backing out from the recursive call that you want to decide to print the line in order or backwards.
The second problem was with the logic in the else
printing condition. You need to print starting at 1, up to the number of rows. I used a dummy variable to achieve this, though there are probably a few other ways of doing it.
def printFigure(rows):
if rows > 0:
printFigure(rows-1)
if rows%2 == 0:
while(rows>0):
print(str(rows)[::-1], end='')
rows -= 1
print('')
else:
i = 1
while (i <= rows):
print(str(i), end='')
i += 1
print('')
printFigure(9)
1
21
123
4321
12345
654321
1234567
87654321
123456789
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
1
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
add a comment |
up vote
4
down vote
accepted
You have two major problems with your current code. First, the check on whether the rows
is even or odd should be happening after the recursive call to printFigure
. The reason for this is that it is after backing out from the recursive call that you want to decide to print the line in order or backwards.
The second problem was with the logic in the else
printing condition. You need to print starting at 1, up to the number of rows. I used a dummy variable to achieve this, though there are probably a few other ways of doing it.
def printFigure(rows):
if rows > 0:
printFigure(rows-1)
if rows%2 == 0:
while(rows>0):
print(str(rows)[::-1], end='')
rows -= 1
print('')
else:
i = 1
while (i <= rows):
print(str(i), end='')
i += 1
print('')
printFigure(9)
1
21
123
4321
12345
654321
1234567
87654321
123456789
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
1
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
You have two major problems with your current code. First, the check on whether the rows
is even or odd should be happening after the recursive call to printFigure
. The reason for this is that it is after backing out from the recursive call that you want to decide to print the line in order or backwards.
The second problem was with the logic in the else
printing condition. You need to print starting at 1, up to the number of rows. I used a dummy variable to achieve this, though there are probably a few other ways of doing it.
def printFigure(rows):
if rows > 0:
printFigure(rows-1)
if rows%2 == 0:
while(rows>0):
print(str(rows)[::-1], end='')
rows -= 1
print('')
else:
i = 1
while (i <= rows):
print(str(i), end='')
i += 1
print('')
printFigure(9)
1
21
123
4321
12345
654321
1234567
87654321
123456789
You have two major problems with your current code. First, the check on whether the rows
is even or odd should be happening after the recursive call to printFigure
. The reason for this is that it is after backing out from the recursive call that you want to decide to print the line in order or backwards.
The second problem was with the logic in the else
printing condition. You need to print starting at 1, up to the number of rows. I used a dummy variable to achieve this, though there are probably a few other ways of doing it.
def printFigure(rows):
if rows > 0:
printFigure(rows-1)
if rows%2 == 0:
while(rows>0):
print(str(rows)[::-1], end='')
rows -= 1
print('')
else:
i = 1
while (i <= rows):
print(str(i), end='')
i += 1
print('')
printFigure(9)
1
21
123
4321
12345
654321
1234567
87654321
123456789
answered Nov 29 at 10:39


Tim Biegeleisen
211k1383130
211k1383130
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
1
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
add a comment |
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
1
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
thanks! I undersatnd my mistake.
– Benjamin Yakobi
Nov 29 at 10:57
1
1
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
@BenjaminYakobi if the answer help you you should accept it, for other who will search the same issue.
– Bear Brown
Nov 29 at 12:51
add a comment |
up vote
3
down vote
if you don't need to use recursivion, other simple solution is:
def printFigure(rows):
for x in range(rows):
items = [str(i) for i in range(1, x + 1)]
if x % 2 == 0:
items = items[::-1]
print(''.join(items))
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
glad you liked it )
– Bear Brown
Nov 29 at 11:10
add a comment |
up vote
3
down vote
if you don't need to use recursivion, other simple solution is:
def printFigure(rows):
for x in range(rows):
items = [str(i) for i in range(1, x + 1)]
if x % 2 == 0:
items = items[::-1]
print(''.join(items))
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
glad you liked it )
– Bear Brown
Nov 29 at 11:10
add a comment |
up vote
3
down vote
up vote
3
down vote
if you don't need to use recursivion, other simple solution is:
def printFigure(rows):
for x in range(rows):
items = [str(i) for i in range(1, x + 1)]
if x % 2 == 0:
items = items[::-1]
print(''.join(items))
if you don't need to use recursivion, other simple solution is:
def printFigure(rows):
for x in range(rows):
items = [str(i) for i in range(1, x + 1)]
if x % 2 == 0:
items = items[::-1]
print(''.join(items))
answered Nov 29 at 10:38


Bear Brown
11.4k81839
11.4k81839
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
glad you liked it )
– Bear Brown
Nov 29 at 11:10
add a comment |
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
glad you liked it )
– Bear Brown
Nov 29 at 11:10
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
extremley short and much more simple than my code for sure.
– Benjamin Yakobi
Nov 29 at 11:08
glad you liked it )
– Bear Brown
Nov 29 at 11:10
glad you liked it )
– Bear Brown
Nov 29 at 11:10
add a comment |
up vote
2
down vote
You could use an inner function that prints from bottom to top, and the call this function from an outer function, for instance:
def print_rows(n, limit):
if n < limit:
numbers = range(1, n + 1) if n % 2 == 1 else reversed(range(1, n + 1))
print(''.join(map(str, numbers)))
print_rows(n + 1, limit)
def print_pyramid_recursive(n):
if n > 0:
print_rows(1, n)
print_pyramid_recursive(10)
Output
1
21
123
4321
12345
654321
1234567
87654321
123456789
In this case the inner function is print_rows
and the outer function is print_pyramid_recursive
. Note, that this problem has a very simple non-recursive solution, for example:
def print_pyramid(n):
for i in range(1, n + 1):
numbers = range(1, i + 1) if i % 2 == 1 else reversed(range(1, i + 1))
print(''.join(map(str, numbers)))
add a comment |
up vote
2
down vote
You could use an inner function that prints from bottom to top, and the call this function from an outer function, for instance:
def print_rows(n, limit):
if n < limit:
numbers = range(1, n + 1) if n % 2 == 1 else reversed(range(1, n + 1))
print(''.join(map(str, numbers)))
print_rows(n + 1, limit)
def print_pyramid_recursive(n):
if n > 0:
print_rows(1, n)
print_pyramid_recursive(10)
Output
1
21
123
4321
12345
654321
1234567
87654321
123456789
In this case the inner function is print_rows
and the outer function is print_pyramid_recursive
. Note, that this problem has a very simple non-recursive solution, for example:
def print_pyramid(n):
for i in range(1, n + 1):
numbers = range(1, i + 1) if i % 2 == 1 else reversed(range(1, i + 1))
print(''.join(map(str, numbers)))
add a comment |
up vote
2
down vote
up vote
2
down vote
You could use an inner function that prints from bottom to top, and the call this function from an outer function, for instance:
def print_rows(n, limit):
if n < limit:
numbers = range(1, n + 1) if n % 2 == 1 else reversed(range(1, n + 1))
print(''.join(map(str, numbers)))
print_rows(n + 1, limit)
def print_pyramid_recursive(n):
if n > 0:
print_rows(1, n)
print_pyramid_recursive(10)
Output
1
21
123
4321
12345
654321
1234567
87654321
123456789
In this case the inner function is print_rows
and the outer function is print_pyramid_recursive
. Note, that this problem has a very simple non-recursive solution, for example:
def print_pyramid(n):
for i in range(1, n + 1):
numbers = range(1, i + 1) if i % 2 == 1 else reversed(range(1, i + 1))
print(''.join(map(str, numbers)))
You could use an inner function that prints from bottom to top, and the call this function from an outer function, for instance:
def print_rows(n, limit):
if n < limit:
numbers = range(1, n + 1) if n % 2 == 1 else reversed(range(1, n + 1))
print(''.join(map(str, numbers)))
print_rows(n + 1, limit)
def print_pyramid_recursive(n):
if n > 0:
print_rows(1, n)
print_pyramid_recursive(10)
Output
1
21
123
4321
12345
654321
1234567
87654321
123456789
In this case the inner function is print_rows
and the outer function is print_pyramid_recursive
. Note, that this problem has a very simple non-recursive solution, for example:
def print_pyramid(n):
for i in range(1, n + 1):
numbers = range(1, i + 1) if i % 2 == 1 else reversed(range(1, i + 1))
print(''.join(map(str, numbers)))
answered Nov 29 at 10:36


Daniel Mesejo
9,5331923
9,5331923
add a comment |
add a comment |
up vote
2
down vote
Here's the simplest loop version:
is_reversed = False
for i in range(1, 10):
step = -1 if is_reversed else 1
print(''.join(map(str, range(1, i + 1)))[::step])
is_reversed = not is_reversed
This regenerates a string every iteration. We can also store the value of the previous result and build on that:
s = ''
is_reversed = False
for i in range(1, 10):
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
And of course, this can easily (and pointlessly) be converted to a tail-recursive function by just passing along the stack:
def f(s, i, max_i, is_reversed):
if i == max_i:
return
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
i += 1
f(s, i, max_i, is_reversed)
f('', 1, 10, False)
The results for each of these increasingly weird looking pieces of code:
1
21
123
4321
12345
654321
1234567
87654321
123456789
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
add a comment |
up vote
2
down vote
Here's the simplest loop version:
is_reversed = False
for i in range(1, 10):
step = -1 if is_reversed else 1
print(''.join(map(str, range(1, i + 1)))[::step])
is_reversed = not is_reversed
This regenerates a string every iteration. We can also store the value of the previous result and build on that:
s = ''
is_reversed = False
for i in range(1, 10):
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
And of course, this can easily (and pointlessly) be converted to a tail-recursive function by just passing along the stack:
def f(s, i, max_i, is_reversed):
if i == max_i:
return
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
i += 1
f(s, i, max_i, is_reversed)
f('', 1, 10, False)
The results for each of these increasingly weird looking pieces of code:
1
21
123
4321
12345
654321
1234567
87654321
123456789
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
add a comment |
up vote
2
down vote
up vote
2
down vote
Here's the simplest loop version:
is_reversed = False
for i in range(1, 10):
step = -1 if is_reversed else 1
print(''.join(map(str, range(1, i + 1)))[::step])
is_reversed = not is_reversed
This regenerates a string every iteration. We can also store the value of the previous result and build on that:
s = ''
is_reversed = False
for i in range(1, 10):
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
And of course, this can easily (and pointlessly) be converted to a tail-recursive function by just passing along the stack:
def f(s, i, max_i, is_reversed):
if i == max_i:
return
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
i += 1
f(s, i, max_i, is_reversed)
f('', 1, 10, False)
The results for each of these increasingly weird looking pieces of code:
1
21
123
4321
12345
654321
1234567
87654321
123456789
Here's the simplest loop version:
is_reversed = False
for i in range(1, 10):
step = -1 if is_reversed else 1
print(''.join(map(str, range(1, i + 1)))[::step])
is_reversed = not is_reversed
This regenerates a string every iteration. We can also store the value of the previous result and build on that:
s = ''
is_reversed = False
for i in range(1, 10):
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
And of course, this can easily (and pointlessly) be converted to a tail-recursive function by just passing along the stack:
def f(s, i, max_i, is_reversed):
if i == max_i:
return
s += str(i)
step = -1 if is_reversed else 1
print(s[::step])
is_reversed = not is_reversed
i += 1
f(s, i, max_i, is_reversed)
f('', 1, 10, False)
The results for each of these increasingly weird looking pieces of code:
1
21
123
4321
12345
654321
1234567
87654321
123456789
edited Nov 29 at 10:49
answered Nov 29 at 10:44
Mateen Ulhaq
11.2k114691
11.2k114691
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
add a comment |
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
thanks man! Its a homework and my function can get only one parameter, but I'll write this solution for my self learning
– Benjamin Yakobi
Nov 29 at 10:56
add a comment |
up vote
1
down vote
You can use simple recursion:
def print_pyramid(_count = 1):
if _count < 10:
print((lambda x:x[::-1] if not _count%2 else x)(''.join(map(str, range(1, _count+1)))))
print_pyramid(_count+1)
print_pyramid()
Output:
1
21
123
4321
12345
654321
1234567
87654321
123456789
add a comment |
up vote
1
down vote
You can use simple recursion:
def print_pyramid(_count = 1):
if _count < 10:
print((lambda x:x[::-1] if not _count%2 else x)(''.join(map(str, range(1, _count+1)))))
print_pyramid(_count+1)
print_pyramid()
Output:
1
21
123
4321
12345
654321
1234567
87654321
123456789
add a comment |
up vote
1
down vote
up vote
1
down vote
You can use simple recursion:
def print_pyramid(_count = 1):
if _count < 10:
print((lambda x:x[::-1] if not _count%2 else x)(''.join(map(str, range(1, _count+1)))))
print_pyramid(_count+1)
print_pyramid()
Output:
1
21
123
4321
12345
654321
1234567
87654321
123456789
You can use simple recursion:
def print_pyramid(_count = 1):
if _count < 10:
print((lambda x:x[::-1] if not _count%2 else x)(''.join(map(str, range(1, _count+1)))))
print_pyramid(_count+1)
print_pyramid()
Output:
1
21
123
4321
12345
654321
1234567
87654321
123456789
answered Nov 29 at 13:14


Ajax1234
39.2k42452
39.2k42452
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53536776%2frecursively-print-pyramid-of-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ofA WBA8z 0x82b2 sk,35biOD2QMAk1nekF JSXHBQeFMxe,zh8k 6 4VJ5pPk28Ad yw,eKD
2
Do you need to use recursion?
– Daniel Mesejo
Nov 29 at 10:32
1
print('n'.join([''.join(map(str, range(1, n+1)[::(-1)**(n%2)])) for n in range(1, 10)]))
– Ev. Kounis
Nov 29 at 10:40
1
@Ev.Kounis
(1,-1)[n%2]
:D– Mateen Ulhaq
Nov 29 at 10:54
@MateenUlhaq It is the same number of bytes though, right? But nice!
– Ev. Kounis
Nov 29 at 10:54
1
@MateenUlhaq
n%2*2-1
and in python2 you can skip parenthesis. And range(9):print'n'.join('123456789'[:n+1][::1-n%2*2]for n in range(9))
– zch
Nov 29 at 12:57