Checking if rendered lightning:input components are populated
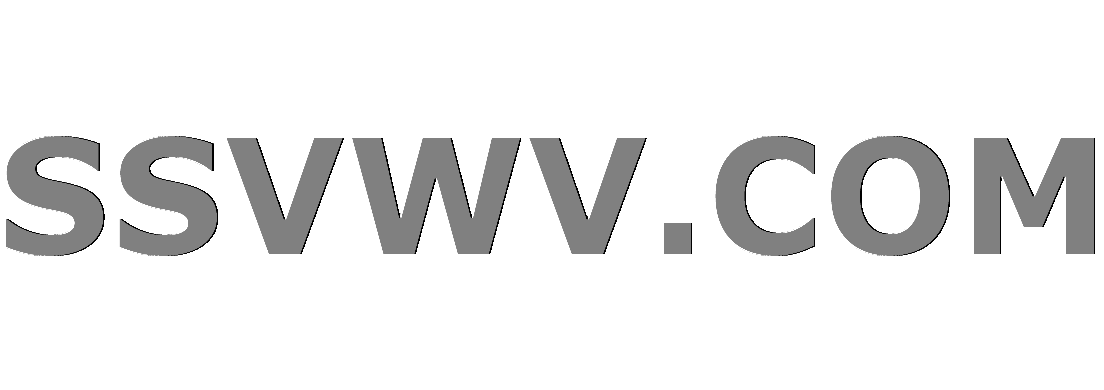
Multi tool use
I am building a Lightning Component form with sections. I want to display a big check mark icon on the section if all the rendered lightning:input fields are filled out.
I have successfully tested this approach:
Markup:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Controller:
testMe : function(component) {
var allComplete = component.find('fieldId').reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
}
The problem arises when I start making the form dynamic using aura:if to conditionally hide lightning:input fields.
If I change my markup to this, the function fails and nothing shows up in the console:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<aura:if isTrue="{! false }">
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
</aura:if>
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Based on the helpful answer from itzmukeshy7, here is my revised, working controller function:
testMe : function(component) {
var rows = component.find('fieldId');
var elements = .concat(rows || );
var allComplete = elements.reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
},
lightning-aura-components lightning
add a comment |
I am building a Lightning Component form with sections. I want to display a big check mark icon on the section if all the rendered lightning:input fields are filled out.
I have successfully tested this approach:
Markup:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Controller:
testMe : function(component) {
var allComplete = component.find('fieldId').reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
}
The problem arises when I start making the form dynamic using aura:if to conditionally hide lightning:input fields.
If I change my markup to this, the function fails and nothing shows up in the console:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<aura:if isTrue="{! false }">
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
</aura:if>
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Based on the helpful answer from itzmukeshy7, here is my revised, working controller function:
testMe : function(component) {
var rows = component.find('fieldId');
var elements = .concat(rows || );
var allComplete = elements.reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
},
lightning-aura-components lightning
add a comment |
I am building a Lightning Component form with sections. I want to display a big check mark icon on the section if all the rendered lightning:input fields are filled out.
I have successfully tested this approach:
Markup:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Controller:
testMe : function(component) {
var allComplete = component.find('fieldId').reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
}
The problem arises when I start making the form dynamic using aura:if to conditionally hide lightning:input fields.
If I change my markup to this, the function fails and nothing shows up in the console:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<aura:if isTrue="{! false }">
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
</aura:if>
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Based on the helpful answer from itzmukeshy7, here is my revised, working controller function:
testMe : function(component) {
var rows = component.find('fieldId');
var elements = .concat(rows || );
var allComplete = elements.reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
},
lightning-aura-components lightning
I am building a Lightning Component form with sections. I want to display a big check mark icon on the section if all the rendered lightning:input fields are filled out.
I have successfully tested this approach:
Markup:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Controller:
testMe : function(component) {
var allComplete = component.find('fieldId').reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
}
The problem arises when I start making the form dynamic using aura:if to conditionally hide lightning:input fields.
If I change my markup to this, the function fails and nothing shows up in the console:
<lightning:input aura:id="fieldId" type="number" name="input1" required="true" label="Enter a number" />
<aura:if isTrue="{! false }">
<lightning:input aura:id="fieldId" type="number" name="input2" required="true" label="Enter another number" />
</aura:if>
<lightning:button variant="brand" label="Check" value="check" onclick="{!c.testMe}"/>
Based on the helpful answer from itzmukeshy7, here is my revised, working controller function:
testMe : function(component) {
var rows = component.find('fieldId');
var elements = .concat(rows || );
var allComplete = elements.reduce(function (validSoFar, inputCmp) {
return validSoFar && !inputCmp.get('v.validity').valueMissing;
}, true);
if (allComplete) {
console.log('All fields complete!');
} else {
console.log('Some fields are missing values.');
}
},
lightning-aura-components lightning
lightning-aura-components lightning
edited 14 hours ago
Matthew Souther
asked 15 hours ago


Matthew SoutherMatthew Souther
935
935
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
This is all fine, there is a trick which is very much hidden in the documentation:
Here is what the documentation says:
So we can handle this with concat
method of array
:
var rows = c.find('rowSelector');
var elements = .concat(rows || );
/* Now here the elements will always be an array, and won't break your code anymore. */
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
Many thanks! Here is my updated controller function, which works.testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
add a comment |
When running your example, I'm getting an error saying reduce is not a valid function. You can enable debug mode for lightning components to get these errors.
The reason for this is your component.find(...) method.
Component.find has two return types. Either an Object or Object.
In the first example you have multiple instances of a component with id "fieldId". Thus the return type will be a list of aura components.
In the second example there is only one aura component as the other one is conditionally rendered. Hence only one component with id "fieldId" is found. Component.find will return a single object and not a array of objects.
You can resolve the issue by first checking if the result of component.find is an array. If not then add it to an array and apply the same reduce method for simplicity sake.
2
Actually, component.find(...) returns three types of value, not two: 1.An array
, if we have more than one element with the provided id. 2.An element
, if we have a unique element with the provided id. 3.undefined
, if we don't have any element with the provided id.
– itzmukeshy7
15 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "459"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f253856%2fchecking-if-rendered-lightninginput-components-are-populated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is all fine, there is a trick which is very much hidden in the documentation:
Here is what the documentation says:
So we can handle this with concat
method of array
:
var rows = c.find('rowSelector');
var elements = .concat(rows || );
/* Now here the elements will always be an array, and won't break your code anymore. */
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
Many thanks! Here is my updated controller function, which works.testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
add a comment |
This is all fine, there is a trick which is very much hidden in the documentation:
Here is what the documentation says:
So we can handle this with concat
method of array
:
var rows = c.find('rowSelector');
var elements = .concat(rows || );
/* Now here the elements will always be an array, and won't break your code anymore. */
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
Many thanks! Here is my updated controller function, which works.testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
add a comment |
This is all fine, there is a trick which is very much hidden in the documentation:
Here is what the documentation says:
So we can handle this with concat
method of array
:
var rows = c.find('rowSelector');
var elements = .concat(rows || );
/* Now here the elements will always be an array, and won't break your code anymore. */
This is all fine, there is a trick which is very much hidden in the documentation:
Here is what the documentation says:
So we can handle this with concat
method of array
:
var rows = c.find('rowSelector');
var elements = .concat(rows || );
/* Now here the elements will always be an array, and won't break your code anymore. */
edited 10 hours ago


Mark Pond
18.5k13288
18.5k13288
answered 15 hours ago
itzmukeshy7itzmukeshy7
2,349922
2,349922
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
Many thanks! Here is my updated controller function, which works.testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
add a comment |
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
Many thanks! Here is my updated controller function, which works.testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
+1 Love this solution, even shorter than what I was using previously.
– sfdcfox
14 hours ago
Many thanks! Here is my updated controller function, which works.
testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Many thanks! Here is my updated controller function, which works.
testMe : function(component) { var rows = component.find('fieldId'); var elements = .concat(rows || ); var allComplete = elements.reduce(function (validSoFar, inputCmp) { return validSoFar && !inputCmp.get('v.validity').valueMissing; }, true); if (allComplete) { console.log('All fields complete!'); } else { console.log('Some fields are missing values.'); } },
– Matthew Souther
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
Great, if it worked for you.
– itzmukeshy7
14 hours ago
add a comment |
When running your example, I'm getting an error saying reduce is not a valid function. You can enable debug mode for lightning components to get these errors.
The reason for this is your component.find(...) method.
Component.find has two return types. Either an Object or Object.
In the first example you have multiple instances of a component with id "fieldId". Thus the return type will be a list of aura components.
In the second example there is only one aura component as the other one is conditionally rendered. Hence only one component with id "fieldId" is found. Component.find will return a single object and not a array of objects.
You can resolve the issue by first checking if the result of component.find is an array. If not then add it to an array and apply the same reduce method for simplicity sake.
2
Actually, component.find(...) returns three types of value, not two: 1.An array
, if we have more than one element with the provided id. 2.An element
, if we have a unique element with the provided id. 3.undefined
, if we don't have any element with the provided id.
– itzmukeshy7
15 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
add a comment |
When running your example, I'm getting an error saying reduce is not a valid function. You can enable debug mode for lightning components to get these errors.
The reason for this is your component.find(...) method.
Component.find has two return types. Either an Object or Object.
In the first example you have multiple instances of a component with id "fieldId". Thus the return type will be a list of aura components.
In the second example there is only one aura component as the other one is conditionally rendered. Hence only one component with id "fieldId" is found. Component.find will return a single object and not a array of objects.
You can resolve the issue by first checking if the result of component.find is an array. If not then add it to an array and apply the same reduce method for simplicity sake.
2
Actually, component.find(...) returns three types of value, not two: 1.An array
, if we have more than one element with the provided id. 2.An element
, if we have a unique element with the provided id. 3.undefined
, if we don't have any element with the provided id.
– itzmukeshy7
15 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
add a comment |
When running your example, I'm getting an error saying reduce is not a valid function. You can enable debug mode for lightning components to get these errors.
The reason for this is your component.find(...) method.
Component.find has two return types. Either an Object or Object.
In the first example you have multiple instances of a component with id "fieldId". Thus the return type will be a list of aura components.
In the second example there is only one aura component as the other one is conditionally rendered. Hence only one component with id "fieldId" is found. Component.find will return a single object and not a array of objects.
You can resolve the issue by first checking if the result of component.find is an array. If not then add it to an array and apply the same reduce method for simplicity sake.
When running your example, I'm getting an error saying reduce is not a valid function. You can enable debug mode for lightning components to get these errors.
The reason for this is your component.find(...) method.
Component.find has two return types. Either an Object or Object.
In the first example you have multiple instances of a component with id "fieldId". Thus the return type will be a list of aura components.
In the second example there is only one aura component as the other one is conditionally rendered. Hence only one component with id "fieldId" is found. Component.find will return a single object and not a array of objects.
You can resolve the issue by first checking if the result of component.find is an array. If not then add it to an array and apply the same reduce method for simplicity sake.
answered 15 hours ago


Lieven JuwetLieven Juwet
762413
762413
2
Actually, component.find(...) returns three types of value, not two: 1.An array
, if we have more than one element with the provided id. 2.An element
, if we have a unique element with the provided id. 3.undefined
, if we don't have any element with the provided id.
– itzmukeshy7
15 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
add a comment |
2
Actually, component.find(...) returns three types of value, not two: 1.An array
, if we have more than one element with the provided id. 2.An element
, if we have a unique element with the provided id. 3.undefined
, if we don't have any element with the provided id.
– itzmukeshy7
15 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
2
2
Actually, component.find(...) returns three types of value, not two: 1.
An array
, if we have more than one element with the provided id. 2. An element
, if we have a unique element with the provided id. 3. undefined
, if we don't have any element with the provided id.– itzmukeshy7
15 hours ago
Actually, component.find(...) returns three types of value, not two: 1.
An array
, if we have more than one element with the provided id. 2. An element
, if we have a unique element with the provided id. 3. undefined
, if we don't have any element with the provided id.– itzmukeshy7
15 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
Thanks for the pointer to use debug mode for Lightning Components -- I will look into that.
– Matthew Souther
14 hours ago
add a comment |
Thanks for contributing an answer to Salesforce Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f253856%2fchecking-if-rendered-lightninginput-components-are-populated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5oo79w