Source permutation
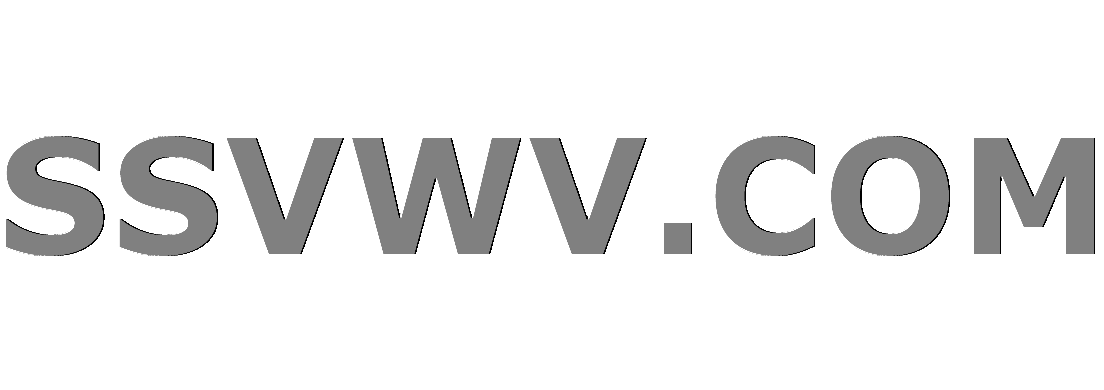
Multi tool use
$begingroup$
A permutation of a set $S = {s_1, s_2, dotsc, s_n}$ is a bijective function $pi: S to S$. For example, if $S = {1,2,3,4}$ then the function $pi: x mapsto 1 + (x + 1 mod 4)$ is a permutation:
$$
pi(1) = 3,quad
pi(2) = 4,quad
pi(3) = 1,quad
pi(4) = 2
$$
We can also have permutations on infinite sets, let's take $mathbb{N}$ as an example: The function $pi: x mapsto x-1 + 2cdot(x mod 2)$ is a permutation, swapping the odd and even integers in blocks of two. The first elements are as follows:
$$
2,1,4,3,6,5,8,7,10,9,12,11,14,13,16,15,dotsc
$$
Challenge
Your task for this challenge is to write a function/program implementing any1 permutation on the positive natural numbers. The score of your solution is the sum of codepoints after mapping them with the implemented permutation.
Example
Suppose we take the above permutation implemented with Python:
def pi(x):
return x - 1 + 2*(x % 2)
Try it online!
The character d
has codepoint $100$, $texttt{pi}(100) = 99$. If we do this for every character, we get:
$$
99,102,101,31,111,106,39,119,42,57,9,31,31,31,31,113,102,115,118,113,109,31,119,31,46,31,50,31,44,31,49,41,39,119,31,38,31,49,42
$$
The sum of all these mapped characters is $2463$, this would be the score for that function.
Rules
You will implement a permutation $pi$ either as a function or program
- given an natural number $x$, return/output $pi(x)$
- for the purpose of this challenge $mathbb{N}$ does not contain $0$
- the permutation must non-trivially permute an infinite subset of $mathbb{N}$
- your function/program is not allowed to read its own source
Scoring
The score is given by the sum of all codepoints (zero bytes may not be part of the source code) under that permutation (the codepoints depend on your language2, you're free to use SBCS, UTF-8 etc. as long as your language supports it).
The submission with the lowest score wins, ties are broken by earliest submission.
1: Except for permutations which only permute a finite subset of $mathbb{N}$, meaning that the set ${ x | pi(x) neq x }$ must be infinite.
2: If it improves your score, you can for example use a UTF-8 encoded Jelly submission instead of the usual SBCS.
code-challenge sequence integer permutations
$endgroup$
add a comment |
$begingroup$
A permutation of a set $S = {s_1, s_2, dotsc, s_n}$ is a bijective function $pi: S to S$. For example, if $S = {1,2,3,4}$ then the function $pi: x mapsto 1 + (x + 1 mod 4)$ is a permutation:
$$
pi(1) = 3,quad
pi(2) = 4,quad
pi(3) = 1,quad
pi(4) = 2
$$
We can also have permutations on infinite sets, let's take $mathbb{N}$ as an example: The function $pi: x mapsto x-1 + 2cdot(x mod 2)$ is a permutation, swapping the odd and even integers in blocks of two. The first elements are as follows:
$$
2,1,4,3,6,5,8,7,10,9,12,11,14,13,16,15,dotsc
$$
Challenge
Your task for this challenge is to write a function/program implementing any1 permutation on the positive natural numbers. The score of your solution is the sum of codepoints after mapping them with the implemented permutation.
Example
Suppose we take the above permutation implemented with Python:
def pi(x):
return x - 1 + 2*(x % 2)
Try it online!
The character d
has codepoint $100$, $texttt{pi}(100) = 99$. If we do this for every character, we get:
$$
99,102,101,31,111,106,39,119,42,57,9,31,31,31,31,113,102,115,118,113,109,31,119,31,46,31,50,31,44,31,49,41,39,119,31,38,31,49,42
$$
The sum of all these mapped characters is $2463$, this would be the score for that function.
Rules
You will implement a permutation $pi$ either as a function or program
- given an natural number $x$, return/output $pi(x)$
- for the purpose of this challenge $mathbb{N}$ does not contain $0$
- the permutation must non-trivially permute an infinite subset of $mathbb{N}$
- your function/program is not allowed to read its own source
Scoring
The score is given by the sum of all codepoints (zero bytes may not be part of the source code) under that permutation (the codepoints depend on your language2, you're free to use SBCS, UTF-8 etc. as long as your language supports it).
The submission with the lowest score wins, ties are broken by earliest submission.
1: Except for permutations which only permute a finite subset of $mathbb{N}$, meaning that the set ${ x | pi(x) neq x }$ must be infinite.
2: If it improves your score, you can for example use a UTF-8 encoded Jelly submission instead of the usual SBCS.
code-challenge sequence integer permutations
$endgroup$
$begingroup$
{s1..sn} (from who build every permutations) are in input? I would remember that a permutation is just one function {1..n}->{s1...sn} injective and on all the set of arrive
$endgroup$
– RosLuP
5 hours ago
add a comment |
$begingroup$
A permutation of a set $S = {s_1, s_2, dotsc, s_n}$ is a bijective function $pi: S to S$. For example, if $S = {1,2,3,4}$ then the function $pi: x mapsto 1 + (x + 1 mod 4)$ is a permutation:
$$
pi(1) = 3,quad
pi(2) = 4,quad
pi(3) = 1,quad
pi(4) = 2
$$
We can also have permutations on infinite sets, let's take $mathbb{N}$ as an example: The function $pi: x mapsto x-1 + 2cdot(x mod 2)$ is a permutation, swapping the odd and even integers in blocks of two. The first elements are as follows:
$$
2,1,4,3,6,5,8,7,10,9,12,11,14,13,16,15,dotsc
$$
Challenge
Your task for this challenge is to write a function/program implementing any1 permutation on the positive natural numbers. The score of your solution is the sum of codepoints after mapping them with the implemented permutation.
Example
Suppose we take the above permutation implemented with Python:
def pi(x):
return x - 1 + 2*(x % 2)
Try it online!
The character d
has codepoint $100$, $texttt{pi}(100) = 99$. If we do this for every character, we get:
$$
99,102,101,31,111,106,39,119,42,57,9,31,31,31,31,113,102,115,118,113,109,31,119,31,46,31,50,31,44,31,49,41,39,119,31,38,31,49,42
$$
The sum of all these mapped characters is $2463$, this would be the score for that function.
Rules
You will implement a permutation $pi$ either as a function or program
- given an natural number $x$, return/output $pi(x)$
- for the purpose of this challenge $mathbb{N}$ does not contain $0$
- the permutation must non-trivially permute an infinite subset of $mathbb{N}$
- your function/program is not allowed to read its own source
Scoring
The score is given by the sum of all codepoints (zero bytes may not be part of the source code) under that permutation (the codepoints depend on your language2, you're free to use SBCS, UTF-8 etc. as long as your language supports it).
The submission with the lowest score wins, ties are broken by earliest submission.
1: Except for permutations which only permute a finite subset of $mathbb{N}$, meaning that the set ${ x | pi(x) neq x }$ must be infinite.
2: If it improves your score, you can for example use a UTF-8 encoded Jelly submission instead of the usual SBCS.
code-challenge sequence integer permutations
$endgroup$
A permutation of a set $S = {s_1, s_2, dotsc, s_n}$ is a bijective function $pi: S to S$. For example, if $S = {1,2,3,4}$ then the function $pi: x mapsto 1 + (x + 1 mod 4)$ is a permutation:
$$
pi(1) = 3,quad
pi(2) = 4,quad
pi(3) = 1,quad
pi(4) = 2
$$
We can also have permutations on infinite sets, let's take $mathbb{N}$ as an example: The function $pi: x mapsto x-1 + 2cdot(x mod 2)$ is a permutation, swapping the odd and even integers in blocks of two. The first elements are as follows:
$$
2,1,4,3,6,5,8,7,10,9,12,11,14,13,16,15,dotsc
$$
Challenge
Your task for this challenge is to write a function/program implementing any1 permutation on the positive natural numbers. The score of your solution is the sum of codepoints after mapping them with the implemented permutation.
Example
Suppose we take the above permutation implemented with Python:
def pi(x):
return x - 1 + 2*(x % 2)
Try it online!
The character d
has codepoint $100$, $texttt{pi}(100) = 99$. If we do this for every character, we get:
$$
99,102,101,31,111,106,39,119,42,57,9,31,31,31,31,113,102,115,118,113,109,31,119,31,46,31,50,31,44,31,49,41,39,119,31,38,31,49,42
$$
The sum of all these mapped characters is $2463$, this would be the score for that function.
Rules
You will implement a permutation $pi$ either as a function or program
- given an natural number $x$, return/output $pi(x)$
- for the purpose of this challenge $mathbb{N}$ does not contain $0$
- the permutation must non-trivially permute an infinite subset of $mathbb{N}$
- your function/program is not allowed to read its own source
Scoring
The score is given by the sum of all codepoints (zero bytes may not be part of the source code) under that permutation (the codepoints depend on your language2, you're free to use SBCS, UTF-8 etc. as long as your language supports it).
The submission with the lowest score wins, ties are broken by earliest submission.
1: Except for permutations which only permute a finite subset of $mathbb{N}$, meaning that the set ${ x | pi(x) neq x }$ must be infinite.
2: If it improves your score, you can for example use a UTF-8 encoded Jelly submission instead of the usual SBCS.
code-challenge sequence integer permutations
code-challenge sequence integer permutations
edited 1 hour ago


xnor
92k18187445
92k18187445
asked 6 hours ago


ბიმობიმო
11.9k22392
11.9k22392
$begingroup$
{s1..sn} (from who build every permutations) are in input? I would remember that a permutation is just one function {1..n}->{s1...sn} injective and on all the set of arrive
$endgroup$
– RosLuP
5 hours ago
add a comment |
$begingroup$
{s1..sn} (from who build every permutations) are in input? I would remember that a permutation is just one function {1..n}->{s1...sn} injective and on all the set of arrive
$endgroup$
– RosLuP
5 hours ago
$begingroup$
{s1..sn} (from who build every permutations) are in input? I would remember that a permutation is just one function {1..n}->{s1...sn} injective and on all the set of arrive
$endgroup$
– RosLuP
5 hours ago
$begingroup$
{s1..sn} (from who build every permutations) are in input? I would remember that a permutation is just one function {1..n}->{s1...sn} injective and on all the set of arrive
$endgroup$
– RosLuP
5 hours ago
add a comment |
5 Answers
5
active
oldest
votes
$begingroup$
Jelly, score 288 250 212 199
-38 thanks to Erik the Outgolfer!
C-*+
Swaps even with odd.
The score is $67+45+44+43=199$ - see self-scoring here.
Try it online!
$endgroup$
$begingroup$
Apparently, Leaky Nun's-*ạ
has a score of 300... however,-*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so-*N+
scores 212
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
add a comment |
$begingroup$
JavaScript (ES6), Score = 276 268
$=>(--$^40)+!0
Try it online!
$endgroup$
$begingroup$
but54^54
is0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
add a comment |
$begingroup$
Retina 0.8.2, 6 bytes, score 260
T`O`RO
Try it online! Link includes self-scoring footer. Simply swaps digits 1
and 9
and 3
and 7
in the decimal representations, so that numbers that contain no digits coprime to 10
are unaffected.
$endgroup$
add a comment |
$begingroup$
Charcoal, 13 bytes, score 681
⁻⁺²³²ι⊗﹪⊖ι²³³
Try it online! Link is to self-scoring version with header to map over an array of byte codes. (Charcoal has a custom code page so I've manually inserted the correct byte codes in the input.) Works by reversing ranges of 233 numbers, so that 117, 350, 583 ... are unchanged. Explanation:
ι Value
⁺ Plus
²³² Literal 232
⁻ Minus
ι Value
⊖ Decremented
﹪ Modulo
²³³ Literal 233
⊗ Doubled
$endgroup$
add a comment |
$begingroup$
Haskell, 42 bytes, score 1114
((a,b)->a*200+mod(b-40)200).(`divMod`200)
Try it online!
$endgroup$
$begingroup$
I don't think this is a permutation, since addinga*200
has no effect modulo 200 soa
doesn't matter.
$endgroup$
– xnor
30 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181262%2fsource-permutation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Jelly, score 288 250 212 199
-38 thanks to Erik the Outgolfer!
C-*+
Swaps even with odd.
The score is $67+45+44+43=199$ - see self-scoring here.
Try it online!
$endgroup$
$begingroup$
Apparently, Leaky Nun's-*ạ
has a score of 300... however,-*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so-*N+
scores 212
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
add a comment |
$begingroup$
Jelly, score 288 250 212 199
-38 thanks to Erik the Outgolfer!
C-*+
Swaps even with odd.
The score is $67+45+44+43=199$ - see self-scoring here.
Try it online!
$endgroup$
$begingroup$
Apparently, Leaky Nun's-*ạ
has a score of 300... however,-*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so-*N+
scores 212
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
add a comment |
$begingroup$
Jelly, score 288 250 212 199
-38 thanks to Erik the Outgolfer!
C-*+
Swaps even with odd.
The score is $67+45+44+43=199$ - see self-scoring here.
Try it online!
$endgroup$
Jelly, score 288 250 212 199
-38 thanks to Erik the Outgolfer!
C-*+
Swaps even with odd.
The score is $67+45+44+43=199$ - see self-scoring here.
Try it online!
edited 4 hours ago
answered 5 hours ago


Jonathan AllanJonathan Allan
52.6k535170
52.6k535170
$begingroup$
Apparently, Leaky Nun's-*ạ
has a score of 300... however,-*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so-*N+
scores 212
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
add a comment |
$begingroup$
Apparently, Leaky Nun's-*ạ
has a score of 300... however,-*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so-*N+
scores 212
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Apparently, Leaky Nun's
-*ạ
has a score of 300... however, -*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Apparently, Leaky Nun's
-*ạ
has a score of 300... however, -*_@
has a score of 250. Maybe I should post that as my own, although it's the same permutation.$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so
-*N+
scores 212$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
Ah nice observation by Leaky Nun, so
-*N+
scores 212$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
$begingroup$
It wasn't an observation, it was a self-answer to his (now pretty old) challenge. ;-)
$endgroup$
– Erik the Outgolfer
5 hours ago
add a comment |
$begingroup$
JavaScript (ES6), Score = 276 268
$=>(--$^40)+!0
Try it online!
$endgroup$
$begingroup$
but54^54
is0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
add a comment |
$begingroup$
JavaScript (ES6), Score = 276 268
$=>(--$^40)+!0
Try it online!
$endgroup$
$begingroup$
but54^54
is0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
add a comment |
$begingroup$
JavaScript (ES6), Score = 276 268
$=>(--$^40)+!0
Try it online!
$endgroup$
JavaScript (ES6), Score = 276 268
$=>(--$^40)+!0
Try it online!
edited 4 hours ago
answered 5 hours ago


ArnauldArnauld
78.2k795326
78.2k795326
$begingroup$
but54^54
is0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
add a comment |
$begingroup$
but54^54
is0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
$begingroup$
but
54^54
is 0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
but
54^54
is 0
$endgroup$
– Jonathan Allan
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
$begingroup$
@JonathanAllan Thanks for notifying. I somehow missed that part. Should be correct now.
$endgroup$
– Arnauld
5 hours ago
add a comment |
$begingroup$
Retina 0.8.2, 6 bytes, score 260
T`O`RO
Try it online! Link includes self-scoring footer. Simply swaps digits 1
and 9
and 3
and 7
in the decimal representations, so that numbers that contain no digits coprime to 10
are unaffected.
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 6 bytes, score 260
T`O`RO
Try it online! Link includes self-scoring footer. Simply swaps digits 1
and 9
and 3
and 7
in the decimal representations, so that numbers that contain no digits coprime to 10
are unaffected.
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 6 bytes, score 260
T`O`RO
Try it online! Link includes self-scoring footer. Simply swaps digits 1
and 9
and 3
and 7
in the decimal representations, so that numbers that contain no digits coprime to 10
are unaffected.
$endgroup$
Retina 0.8.2, 6 bytes, score 260
T`O`RO
Try it online! Link includes self-scoring footer. Simply swaps digits 1
and 9
and 3
and 7
in the decimal representations, so that numbers that contain no digits coprime to 10
are unaffected.
answered 3 hours ago
NeilNeil
81.5k745178
81.5k745178
add a comment |
add a comment |
$begingroup$
Charcoal, 13 bytes, score 681
⁻⁺²³²ι⊗﹪⊖ι²³³
Try it online! Link is to self-scoring version with header to map over an array of byte codes. (Charcoal has a custom code page so I've manually inserted the correct byte codes in the input.) Works by reversing ranges of 233 numbers, so that 117, 350, 583 ... are unchanged. Explanation:
ι Value
⁺ Plus
²³² Literal 232
⁻ Minus
ι Value
⊖ Decremented
﹪ Modulo
²³³ Literal 233
⊗ Doubled
$endgroup$
add a comment |
$begingroup$
Charcoal, 13 bytes, score 681
⁻⁺²³²ι⊗﹪⊖ι²³³
Try it online! Link is to self-scoring version with header to map over an array of byte codes. (Charcoal has a custom code page so I've manually inserted the correct byte codes in the input.) Works by reversing ranges of 233 numbers, so that 117, 350, 583 ... are unchanged. Explanation:
ι Value
⁺ Plus
²³² Literal 232
⁻ Minus
ι Value
⊖ Decremented
﹪ Modulo
²³³ Literal 233
⊗ Doubled
$endgroup$
add a comment |
$begingroup$
Charcoal, 13 bytes, score 681
⁻⁺²³²ι⊗﹪⊖ι²³³
Try it online! Link is to self-scoring version with header to map over an array of byte codes. (Charcoal has a custom code page so I've manually inserted the correct byte codes in the input.) Works by reversing ranges of 233 numbers, so that 117, 350, 583 ... are unchanged. Explanation:
ι Value
⁺ Plus
²³² Literal 232
⁻ Minus
ι Value
⊖ Decremented
﹪ Modulo
²³³ Literal 233
⊗ Doubled
$endgroup$
Charcoal, 13 bytes, score 681
⁻⁺²³²ι⊗﹪⊖ι²³³
Try it online! Link is to self-scoring version with header to map over an array of byte codes. (Charcoal has a custom code page so I've manually inserted the correct byte codes in the input.) Works by reversing ranges of 233 numbers, so that 117, 350, 583 ... are unchanged. Explanation:
ι Value
⁺ Plus
²³² Literal 232
⁻ Minus
ι Value
⊖ Decremented
﹪ Modulo
²³³ Literal 233
⊗ Doubled
answered 3 hours ago
NeilNeil
81.5k745178
81.5k745178
add a comment |
add a comment |
$begingroup$
Haskell, 42 bytes, score 1114
((a,b)->a*200+mod(b-40)200).(`divMod`200)
Try it online!
$endgroup$
$begingroup$
I don't think this is a permutation, since addinga*200
has no effect modulo 200 soa
doesn't matter.
$endgroup$
– xnor
30 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
add a comment |
$begingroup$
Haskell, 42 bytes, score 1114
((a,b)->a*200+mod(b-40)200).(`divMod`200)
Try it online!
$endgroup$
$begingroup$
I don't think this is a permutation, since addinga*200
has no effect modulo 200 soa
doesn't matter.
$endgroup$
– xnor
30 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
add a comment |
$begingroup$
Haskell, 42 bytes, score 1114
((a,b)->a*200+mod(b-40)200).(`divMod`200)
Try it online!
$endgroup$
Haskell, 42 bytes, score 1114
((a,b)->a*200+mod(b-40)200).(`divMod`200)
Try it online!
edited 14 mins ago
answered 1 hour ago


Joseph SibleJoseph Sible
2175
2175
$begingroup$
I don't think this is a permutation, since addinga*200
has no effect modulo 200 soa
doesn't matter.
$endgroup$
– xnor
30 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
add a comment |
$begingroup$
I don't think this is a permutation, since addinga*200
has no effect modulo 200 soa
doesn't matter.
$endgroup$
– xnor
30 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
$begingroup$
I don't think this is a permutation, since adding
a*200
has no effect modulo 200 so a
doesn't matter.$endgroup$
– xnor
30 mins ago
$begingroup$
I don't think this is a permutation, since adding
a*200
has no effect modulo 200 so a
doesn't matter.$endgroup$
– xnor
30 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
$begingroup$
@xnor Good catch. That's supposed to be outside of the mod. At some point while golfing, I must have messed up order of operations. Fixed now, thanks!
$endgroup$
– Joseph Sible
13 mins ago
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f181262%2fsource-permutation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nE9yfqRuCw
$begingroup$
{s1..sn} (from who build every permutations) are in input? I would remember that a permutation is just one function {1..n}->{s1...sn} injective and on all the set of arrive
$endgroup$
– RosLuP
5 hours ago